Try a lot of possible correct solutions are wrong, and finally copy the cmakelists.txt in the error content of the terminal, rename the cmake file you wrote, compile it, want to curse
Tag Archives: cmake
[Solved] Ceres Compile error: ‘integer_sequence’ is not a member of ‘std‘
Modifying cmake will set the C + + standard
set(CMAKE_CXX_FLAGS "-std=c++11")
Replace with
set(CMAKE_CXX_STANDARD 11)
It can be solved
In the new version of cmake, the following method is used to set C + + standard
[Solved] Denseflow Install Error: fatal error: opencv2/cudaarithm.hpp: No such file or directory
Installing denseflow compiles with the following error./home/m/src/denseflow/src/denseflow_gpu.cpp:2:10: fatal error: opencv2/cudaarithm.hpp: No such file or directory
#include “opencv2/cudaarithm.hpp”
where the keywords are
/home/m/src/denseflow/src/denseflow_gpu.cpp
cudaarithm.hpp
The solution is as follows.
1、Find the path where cudaarithm.hpp is located
sudo find/-name "cudaarithm.hpp"
A path similar to:
/home/m/src/opencv_contrib/modules/cudaarithm/include/opencv2/cudaarithm.hpp
/home/m/include/opencv4/opencv2/cudaarithm.hpp
............
..............
Then fill the absolute path into denseflow_ Gpu.cpp replaces relative path
#You have to fill in the absolute paths, and our paths may be different, you have to follow your own
sudo vim /home/m/src/denseflow/src/denseflow_gpu.cpp
Before replacing
#include “opencv2/cudaarithm.hpp”
After replacement
#include “/home/m/include/opencv4/opencv2/cudaarithm.hpp”
Compile again, the problem is solved.
“Android.””IDE,” CMakereward Invalid revision: 3.18.1-g262b901-dirtyerror
This error is due to the high version of cmake
In SDK manager, you can uninstall a higher version and download a lower version of cmake, such as version 3.6
Cmake[Warning]:Policy CMP0054 is not set
Policy CMP0054 is not set: Only interpret if() arguments as variables or keywords when unquoted
Refer to prompt cause solution
reference resources
How to use variables and avoid CMP0054 policy violations?CMP0054
Prompt reason
Note: You only get the CMP0054 warning if the context of your variable was actually evaluated again (so it’s a hint your CMake code will not work in those places).
solve
cmake_policy(SET CMP0054 NEW)
When vs2017 compiles CUDA project, “error msb6006:“ cmd.exe ”Exited with code 1 Solutions for
Error MSB6006: “cmd.exe” has exited with code 1.
Google found that it should be because the default VS installation path of CUDA is on C disk, so it could not be found. The solution is to find the CUDA_HOST_COMPILER item in cmake-gui and change it to your own compiled path, such as mine:

This should compile properly.
The C compiler identification is unknown
The environment
CLion+Cmake+Mingw
An error log
-- The C compiler identification is unknown
-- Check for working C compiler: D:/MinGW/bin/gcc.exe
-- Check for working C compiler: D:/MinGW/bin/gcc.exe -- broken
CMake Error at D:/Program Files/JetBrains/CLion 2019.2.3/bin/cmake/win/share/cmake-3.15/Modules/CMakeTestCCompiler.cmake:60 (message):
The C compiler
"D:/MinGW/bin/gcc.exe"
is not able to compile a simple test program.
If it is a CPP program, it may be reported again
-- The CXX compiler identification is unknown
why
CMAKE could not check the C Compiler ID for unknown reasons, causing compilation to fail
The solution
Add the following configuration to the cMakelists.txt file to force the Compiler ID to be specified
INCLUDE(CMakeForceCompiler)
CMAKE_FORCE_C_COMPILER(gcc GNU)
CMAKE_FORCE_CXX_COMPILER(g++ GNU)
Rerun the cmake directive after adding it
The cxx compiler identification is unknown
//Configure VS2015/VS2015/VS2015/VS2015/VS2015/VS2015/VS2015
The CXX compiler identification is unknown
Cmake can’t find the C++ compiler. Check the VS installation directory for cl.exe, rc.exe and rcdll. DLL.
If you do not have cl.exe, you may have only installed VS and no VC compiler. Open VS and select VC to install.
If you do not have rc.exe and rcdll. DLL, which are used for compiling resources, you may install them in the Windows SDK. You can directly copy these two files to the VC/bin directory.
windows CMake error: error in configuration process, project files may be invalid
Cmake error: error in configuration process, project files may be invalid
Cmake error: error in configuration process, project files may be invalid
Windows 10×64
cmake version: 3.16.0
version: 2015
Solution: VS Uninstall, install VS2019, compile and pass. Record for reference.
Problems in compiling VTK with cmake: solutions to error configuration process, project files may be invalid
After setting up the basic VTK environment, the learning process follows. But I had a bunch of problems in the first example. Here’s how to solve them. Fill in a bunch of holes first.
1. Problem introduction
I follow: Dongling VTK tutorial series navigation learning.
>
>
>
>
>
>
>
>
>
The first is the cmake code:
cmake_minimum_required(VERSION2.8)
project(TestVTKInstall)
find_package(VTKREQUIRED)
include(${VTK_USE_FILE})
add_executable(${PROJECT_NAME}TestVTKInstall.cpp)
target_link_libraries(${PROJECT_NAME}vtkRendering vtkCommon)
Then the CPP code:
#include <vtkRenderWindow.h>
#include <vtkSmartPointer.h>
int main()
{
vtkSmartPointer<vtkRenderWindow> renWin = vtkSmartPointer<vtkRenderWindow>::New();
renWin->Render();
std::cin.get();
return 0;
}
ror configuration process, project files may be invalid
.>r> error configuration process, project files may be invalid
2. Problem solving:
Error Configuration Process: The Project Files may be invalid method did not work. Here’s a little bit about this approach.
2.1 No use of CMAKE to select the version of VS on the corresponding machine.
When generating the corresponding VS solution with the CMAKE configuration, you need to correspond to the correct VS version. This is the same version that you used when installing VTK.
2.2 Previous error cache is not cleaned
|
to clear the cache.
click on the top left corner of the file - & gt; Delete cathe
2. 3 cmake list programming problems
Following the above description did not solve our problem, so we had to continue to Google. We saw us have similar questions on several StackOverflow posts.
- VTK + Cmake -> USE_VTK_RENDERING erroCMake error: ‘Target is not built by this project’ Cmake link liabray target link errorCMake OpenCV Cannot Specify link Libraries
According to the above, compared with our CMMakelists code, we tried one code after another, and finally found a very hidden error, that is, we must pay attention to the space in CMMakelists!!
To be specific,
- is the first line:
rsion
a> code>2.8>e> have a space> The third line: VTK
d r>red
has a s> on line 9, testvtkinstal>cpp
has a space on line> In line 11, we change the previous vtkRendering vtkCommon
$>tk_libraries}
with a>e before. There is nothing wrong with cmake, though, if you simply add a space. However, when VS is compiled later, the following error will occur, so it will be fine to fix it. 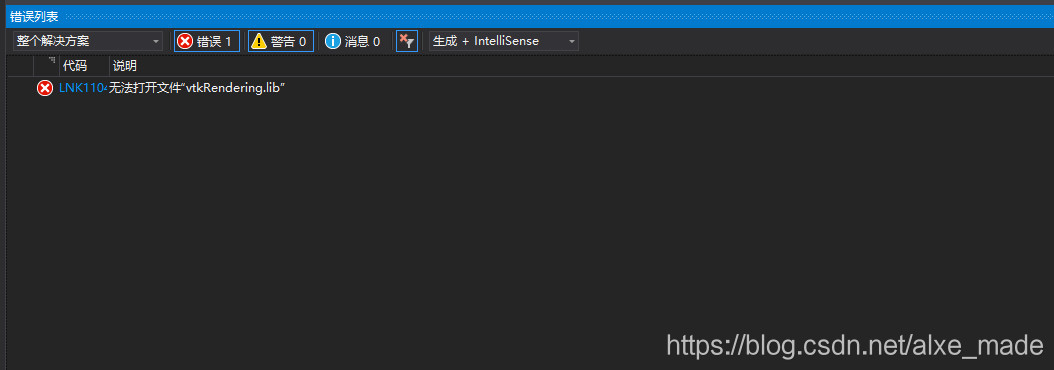
Finally, the cmMakelists.txt code:
cmake_minimum_required(VERSION 2.8)
project(TestVTKInstall)
find_package(VTK REQUIRED)
include(${VTK_USE_FILE})
add_executable(${PROJECT_NAME} TestVTKInstall.cpp)
target_link_libraries(${PROJECT_NAME} ${VTK_LIBRARIES})
3. Run in VS
After cmake has no errors, we configure, then generate. Open VS2017 as an administrator. Open the file we compiled.
> compile l_build
>>ck on F7 or build it. If there are no errors, right-click TestVTKInstall and set it as a startup item, then click F5, or run to get the correct results.
Over
Just solve problems one by one.
CMake error: error in configuration process, project files may be invalid
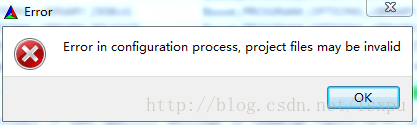
You can check for specific errors in Cmake:
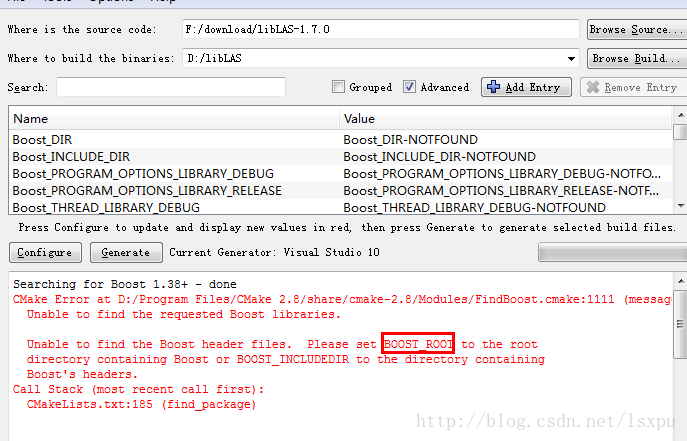
Boost_root is an environment variable that should be used in the process of installing BOOSTPRO. The BOOST_ROOT is an environment variable that should be used in the process of installing BOOSTPRO. The BOOST_ROOT is an environment variable. I added myself to this file
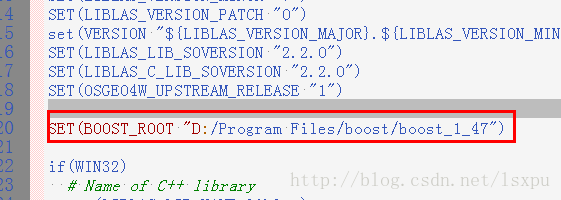
//Configure CMake// Configure
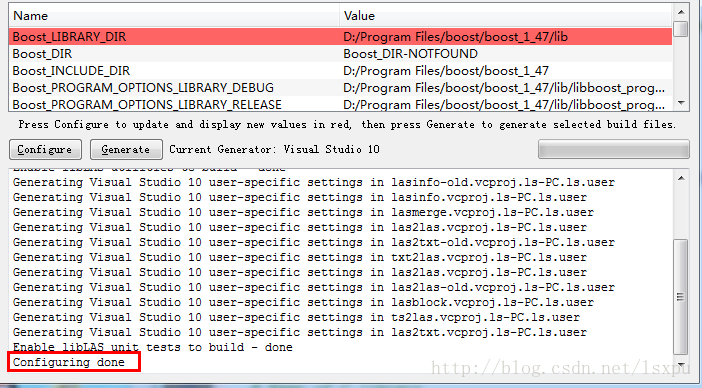
Reference:
【 1 】 http://boostpro.com/download/boost_1_47_setup.exe
【 2 】 Cmake always find a Boost libraries at http://stackoverflow.com/questions/19303430/cmake-cannot-find-boost-libraries
[3] solution: CMake compile time appear the error in the configuration process project files may be invalid at http://blog.sina.com.cn/s/blog_a2d9d5c60100y1at.html