In this case, the common problems are as follows:
1, mapper.xml inside the namespace and the actual mapper class path is not consistent.
There is a quick way to detect this is to hold down the ctrl key, and then click the package name inside the namespace, if you can jump to the corresponding class, it means there is no problem, if you use the IDEA is the same way, IDEA package name can be segmented, as long as you can point in are no problem.
2, mapper interface function name and mapper.xml inside the label id is not consistent.
This problem is also very common, the best way is to paste and copy it, so as to ensure that there is no problem.
The first point and 2 points are about spelling errors.
3, the build did not go in, please see if these exist below the target folder, no please rebuild
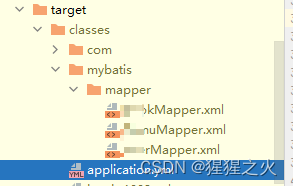
4. Check whether package scanning is added. I added it to the spring boot startup class.
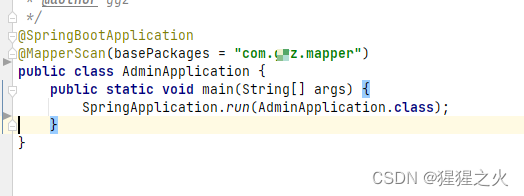
5. Check whether the configuration file is written incorrectly or not
#Is this place wrongly written?
mapper-locations: classpath:mybatis/mapper/**/*.xml
Mybatis-plus can be configured as an array:
mybatis-plus:
mapper-locations:
- classpath:mybatis/mapper/**/*.xml
Or
mybatis-plus:
mapper-locations: classpath:mybatis/**/*Mapper.xml
Note that the key is mapper-locations and not mapper-locations:
Other configurations:
mybatis-plus:
global-config:
#primary-key-type 0: "Database ID self-incrementing", 1: "User input ID", 2: "Global unique ID (numeric unique ID)", 3: "Global unique ID UUID";
id-type: 0
#field-strategy 0: "Ignore judgment",1: "Non-NULL judgment"),2: "Non-Null judgment"
field-strategy: 0
#hump-underline conversion
db-column-underline: true
#refresh-mapper debugging artifact
refresh-mapper: true
#database capital-underline conversion
#capital-mode: true
#sequence interface implementation class configuration
#key-generator: com.baomidou.springboot.xxx
#logic-delete-configuration (the following 3 configurations)
#logic-delete-value: 0 # logical-delete value (default is 1)
#logic-not-delete-value: 1 # logical-not-delete value (default is 0)
# Custom fill policy interface implementation
#meta-object-handler: com.zhengqing.config.
#custom SQL injector
#sql-injector: com.baomidou.springboot.xxx configuration:
#SQL parsing cache, multi-tenant @SqlParser annotation takes effect when enabled
#sql-parser-cache: true
configuration:
# hump conversion Similar mapping from database column names to Java property hump naming
map-underscore-to-camel-case: true
# Whether to enable caching
cache-enable: false
# If the query contains a column with a null value, MyBatis will not map this field when mapping
#call-setters-on-nulls: true
# 打印sql
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl