1. Go to GLFW official website to download the corresponding version of the configuration file: click the open link, no matter your system is 32-bit or 64-bit, it is recommended to download the 32-bit configuration file, as shown below:
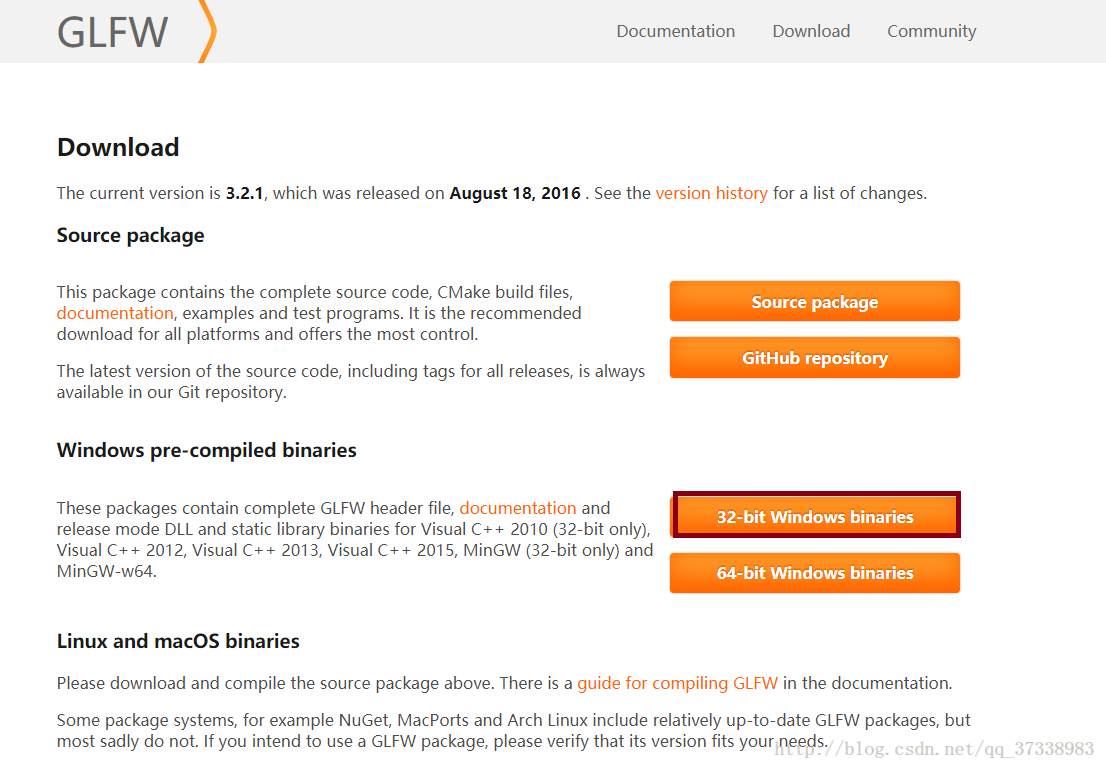
Go to the Glad Online service to get the Glad. H configuration file and click the link to open it. Fill in the information as shown below to get the Glad. Zip file:
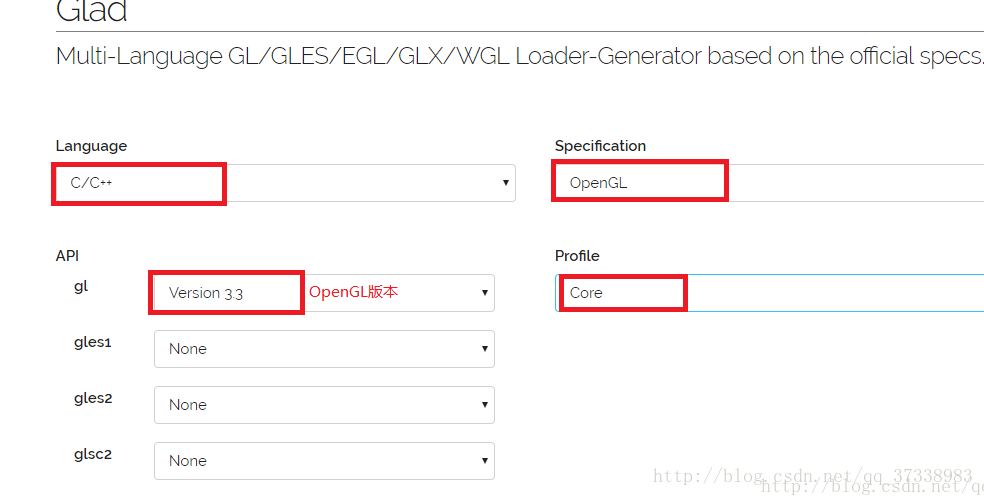
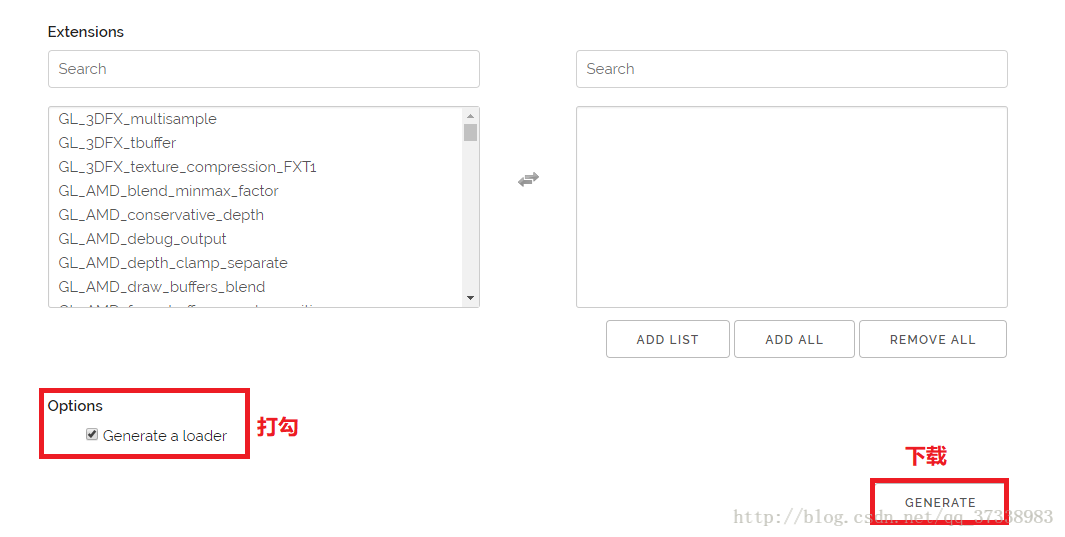
2. Unzip the downloaded file to get the configuration library of each version of VS. You can choose according to the version of VS you have installed. After decompression glad.zip can see two folders: include and SRC, we will include KHR and glad in folder folder to include new folder, as shown in the figure below:
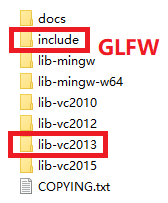
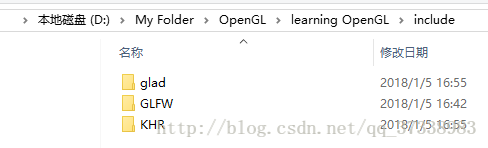
Then place the lib-vc2013 folder and include folder in the same folder, as shown in the figure below:
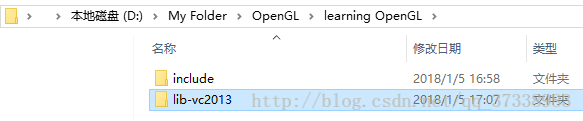
Good to here folder finishing work, then see how to configure!!
3. Copy the glfw3.dll files from the lib-vc2013 folder to the system folders: C:\ windows\ System32 and C:\ windows\ SysWOW64.
4. Open VS2013, create a new console application, create a new demo.cpp, and add the glad.c in the SRC folder from which the glad.zip is unzips to the project, as shown in the figure below:
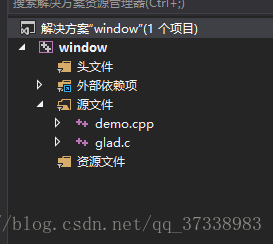
5. Link to contain directory and library directory, right-click Solution: Properties ->; Configure properties ->; VC++ Directory: Include the paths to the two folders from Step 2, as shown in the figure below:
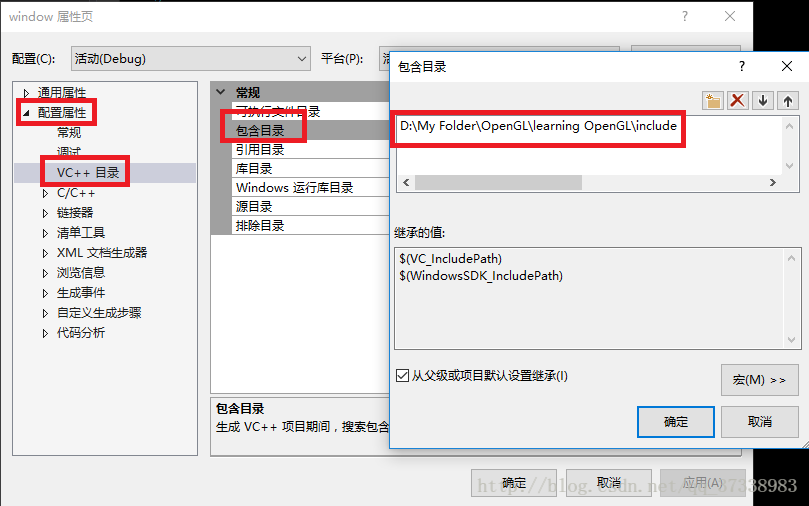
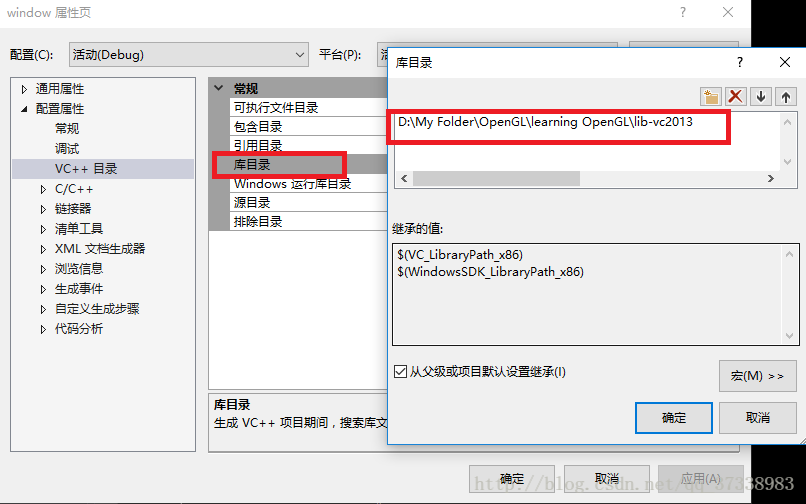
6. Link libraries: opengl32.lib and glfw3.lib. Right-click Solution: Properties ->; Configure properties ->; The linker – & gt; Add a dependency, as shown in the figure below:
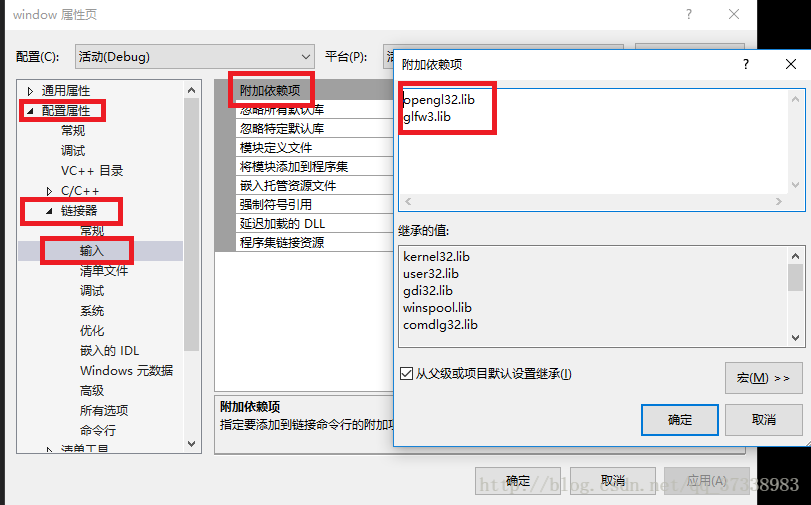
Here, the configuration is complete, let’s run the first example!!
7. Implement an OpenGL window: Hello Window.
The demo. CPP code:
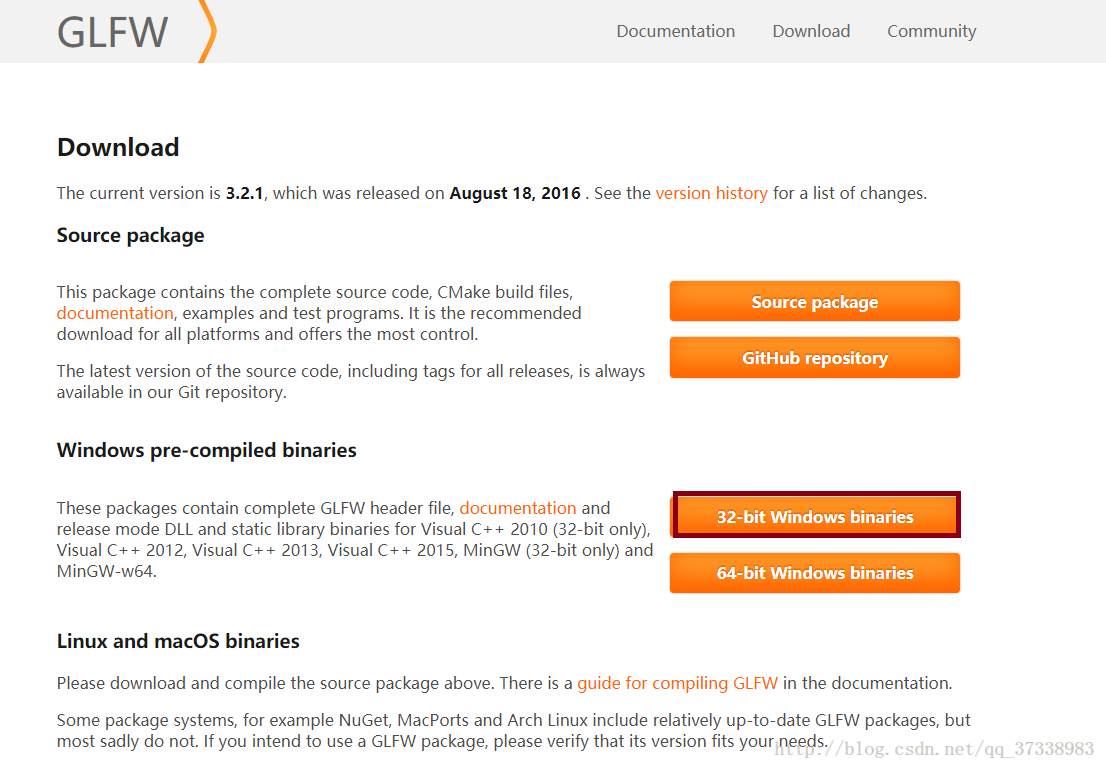
Go to the Glad Online service to get the Glad. H configuration file and click the link to open it. Fill in the information as shown below to get the Glad. Zip file:
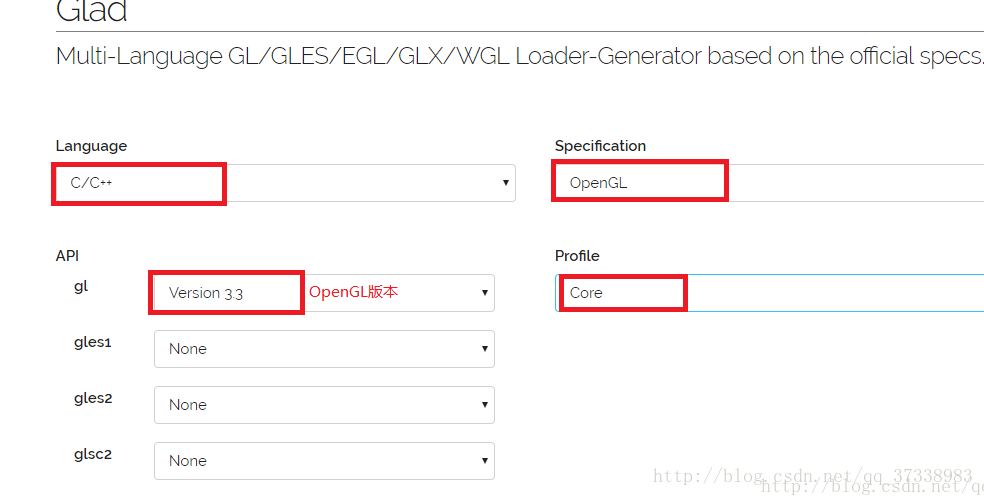
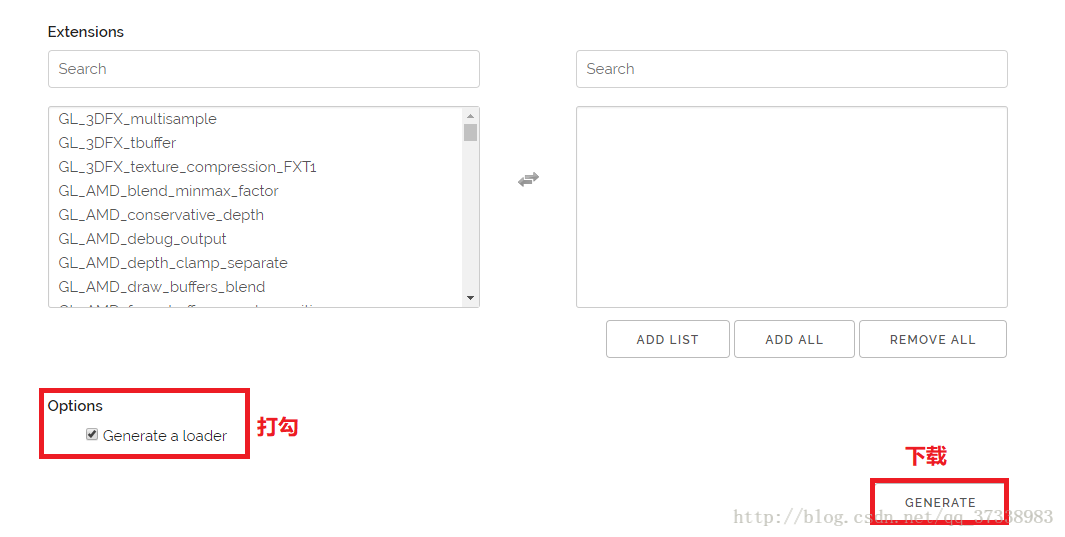
2. Unzip the downloaded file to get the configuration library of each version of VS. You can choose according to the version of VS you have installed. After decompression glad.zip can see two folders: include and SRC, we will include KHR and glad in folder folder to include new folder, as shown in the figure below:
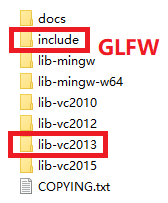
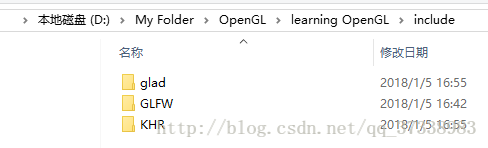
Then place the lib-vc2013 folder and include folder in the same folder, as shown in the figure below:
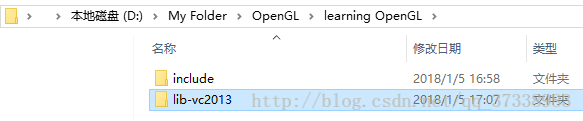
Good to here folder finishing work, then see how to configure!!
3. Copy the glfw3.dll files from the lib-vc2013 folder to the system folders: C:\ windows\ System32 and C:\ windows\ SysWOW64.
4. Open VS2013, create a new console application, create a new demo.cpp, and add the glad.c in the SRC folder from which the glad.zip is unzips to the project, as shown in the figure below:
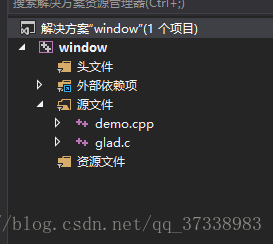
5. Link to contain directory and library directory, right-click Solution: Properties ->; Configure properties ->; VC++ Directory: Include the paths to the two folders from Step 2, as shown in the figure below:
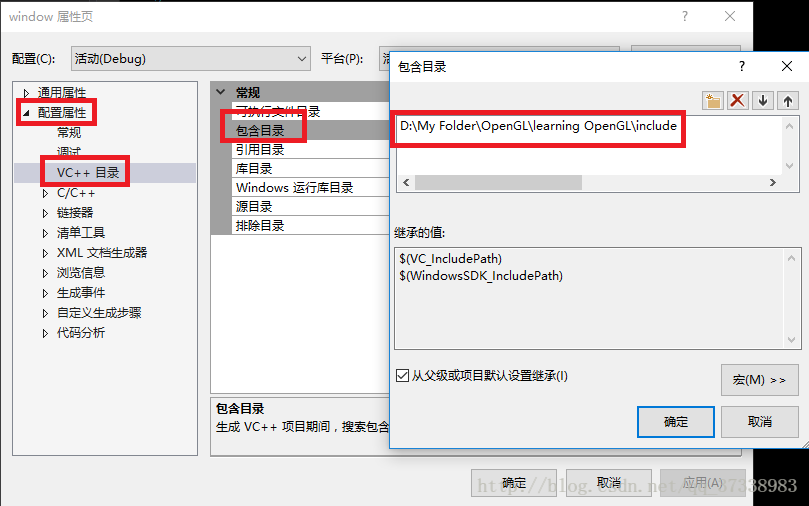
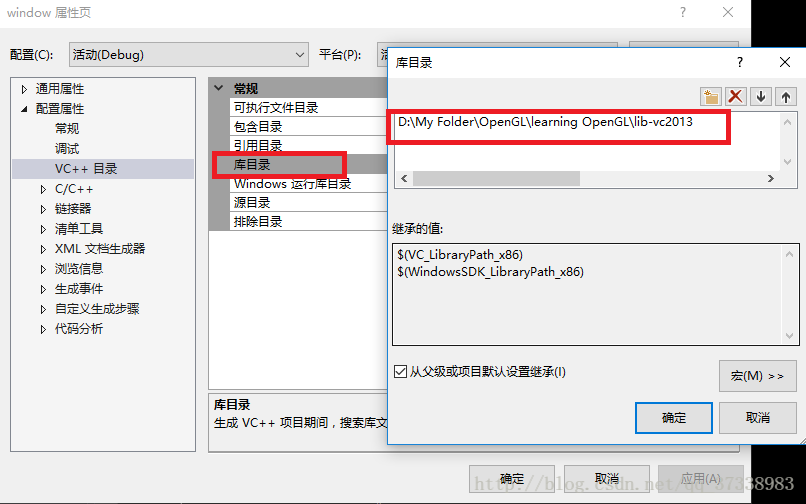
6. Link libraries: opengl32.lib and glfw3.lib. Right-click Solution: Properties ->; Configure properties ->; The linker – & gt; Add a dependency, as shown in the figure below:
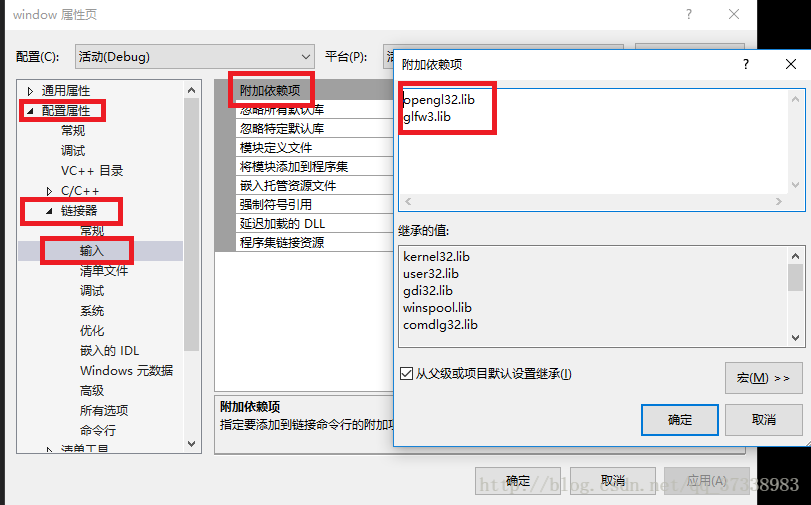
Here, the configuration is complete, let’s run the first example!!
7. Implement an OpenGL window: Hello Window.
The demo. CPP code:
#include <glad\glad.h>
#include <GLFW\glfw3.h>
#include <iostream>
void framebuffer_size_callback(GLFWwindow* window, int width, int height);
void processInput(GLFWwindow *window);
// settings
const unsigned int SCR_WIDTH = 800;
const unsigned int SCR_HEIGHT = 600;
int main()
{
// glfw: initialize and configure
// ------------------------------
glfwInit();
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
#ifdef __APPLE__
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE); // uncomment this statement to fix compilation on OS X
#endif
// glfw window creation
// --------------------
GLFWwindow* window = glfwCreateWindow(SCR_WIDTH, SCR_HEIGHT, "LearnOpenGL", NULL, NULL);
if (window == NULL)
{
std::cout << "Failed to create GLFW window" << std::endl;
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
glfwSetFramebufferSizeCallback(window, framebuffer_size_callback);
// glad: load all OpenGL function pointers
// ---------------------------------------
if (!gladLoadGLLoader((GLADloadproc)glfwGetProcAddress))
{
std::cout << "Failed to initialize GLAD" << std::endl;
return -1;
}
// render loop
// -----------
while (!glfwWindowShouldClose(window))
{
// input
// -----
processInput(window);
// glfw: swap buffers and poll IO events (keys pressed/released, mouse moved etc.)
// -------------------------------------------------------------------------------
glfwSwapBuffers(window);
glfwPollEvents();
}
// glfw: terminate, clearing all previously allocated GLFW resources.
// ------------------------------------------------------------------
glfwTerminate();
return 0;
}
// process all input: query GLFW whether relevant keys are pressed/released this frame and react accordingly
// ---------------------------------------------------------------------------------------------------------
void processInput(GLFWwindow *window)
{
if (glfwGetKey(window, GLFW_KEY_ESCAPE) == GLFW_PRESS)
glfwSetWindowShouldClose(window, true);
}
// glfw: whenever the window size changed (by OS or user resize) this callback function executes
// ---------------------------------------------------------------------------------------------
void framebuffer_size_callback(GLFWwindow* window, int width, int height)
{
// make sure the viewport matches the new window dimensions; note that width and
// height will be significantly larger than specified on retina displays.
glViewport(0, 0, width, height);
}
Running results:
Read More:
- Vs2015 configuring OpenGL development environment: configuration of glfw library and glad Library
- Configuration of OpenGL development environment under Windows environment, win10 + vs2019 + glfw + glad
- Simple configuration of glfw + glad in vs2015 OpenGL development environment
- Configuring glfw library and glad Library in opengl-vs2015
- OpenGL, such as glad library and glfw library, is incompatible
- Configure OpenGL development environment (glfw3 + glad) once and for all with visual studio
- OpenGL class library and environment configuration under win10 and vs2015
- Vs2013 + glfw + glew configure OpenGL development environment
- Vs2015 OpenGL environment configuration
- Vs2015 configuring OpenGL (glfw Library)
- (64 bit) OpenGL configuration + vs2017 + glew + glfw
- Construction of vs2015 OpenGL configuration environment
- OpenGL development environment configuration [vs2017] + common problems
- Clion MinGW super fast configuration OpenGL development environment
- Vs2015 + OpenGL environment configuration
- OpenGL environment configuration under VS2010 / vs2012 / vs2015
- On the configuration of OpenGL Red Book eighth edition environment in vs2013
- Problems encountered in vs2015 configuration using OpenGL environment
- OpenGL + vs2015 environment configuration problem solving
- Configure OpenGL development environment (vs2015)