glMatrixMode(gl_modelView);
glMatrixMode(gl_modelView);
// Reset the model observation matrix.
glLoadIdentity ();
//
selection model observation matrix gluLookAt (3.0 f to 2.5 f to 5.0 f to 0.0 f to 0.0 f to 0.0 f to 0.0 f to 1.0 f to 0.0 f);
Note: Be sure to call glLoIdentity to reset the currently specified matrix to the identity matrix before calling gluLookAt.
br> <>>
2. When you do texture mapping, you find that the texture and color are mixed.
To mask the effects of GLColor3F, simply add:
GlColor3f (1.0, 1.0, 1.0);
or add: glTexenvi (GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_REPLACE);
glgentextures6, &
glgentextures6, & texture[i]);
glBindTexture (GL_TEXTURE_2D, texture [I]);
glutInit(&
glutInit)
glutInit(&
glutInit)
glutInit(&
glutInit)
glutInit(& argc, argv);
glutInitDisplayMode(GLUT_DEPTH | GLUT_DOUBLE | GLUT_RGBA);
glutInitWindowPosition (0, 0);
glutInitWindowSize(800, 600);
glutCreateWindow(“Wander”);
wander.load_GL_textures1();
=
=
=
=
=
=
=
1.
GlFrontFace (GL_CCW); // Specifies counterclockwise as the front
GlCullFace (GL_BACK); // Make the back side invisible
GlEnable (GL_CULL_FACE);
2.
// Pyramid objects are not mapped, so you should not enable texture mapping, otherwise you will not see the object
//glEnable(GL_TEXTURE_2D);
// Enable texture mapping, enable texture mapping before the object to be mapped, disable texture mapping after the object is drawn, if enabled before all the objects to be drawn, the object will not be seen if GLTextCoord2f is not used
3.
GlClear (GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); // Clear Screen And Depth Buffer
GlMatrixMode (GL_MODELVIEW); // Set the Model View Matrix to determine the position of an object in 3D space
GlLoadIdentity (); //M=I; I is the identity matrix
GlColor3f (1.0f, 0.0f, 0.0f);
GlBegin (GL_TRIANGLES);
GlVertex3f (0.0 f, f, 2.0 0.0 f);
GlVertex3f (0.0-1.0 f to 1.0 f, f);
GlVertex3f (0.0 1.0 f to 1.0 f, f);
GlEnd ();
GlTranslatef (0.0 1.0 f, 3.0 f, f); // If x is shifted by 1, y is shifted by 3, and z is guaranteed to remain the same, the corresponding matrix is:
// After translation, M=M*K=I*K,K = x shifted by 1, y shifted by 3, z guaranteed invariant translation matrix
// GlRotatef (90.0F, 0.0F, 0.0F, 1.0F); // Rotate 90 degrees counterclockwise
GlRotatef (-90.0F, 0.0F, 0.0F, 1.0F); // Rotate 90 degrees clockwise
// After rotation, M=M*R=K*R,R is the corresponding selection matrix above
/ *
K =
1 0 0 1
0 1 0 3
1 0 0 0
0 0 0 to 1
The rotation matrix of @ degrees about the z axis, R is equal to
Cos @ – sin @ 0 0
Sin @ cos @ 0 0
1 0 0 0
0 0 0 to 1
Substitute @=-90 to get:
1 0 0 0
1 0 0 0
1 0 0 0
0 0 0 to 1
Have to M =
1 0 0 1
1 0 0. 3
1 0 0 0
0 0 0 to 1
And then multiply the homogeneous coordinates of the following three coordinates right by M to get the final coordinates,
P ‘= MP, P for the original coordinates of the corresponding homogeneous coordinates, such as the first point (0.0, 2.0, 0.0) of homogeneous coordinates (0.0, 2.0, 0.0, 1.0) for
The final three coordinate positions are:
A1 =
3
3
0
1
A2 =
0
4
0
1
A3 =
0
2
0
1
Finally, draw the triangle corresponding to the three points
* /
GlColor3f (0.0 1.0 f, 0.0 f, f);
GlBegin (GL_TRIANGLES);
GlVertex3f (0.0 f, f, 2.0 0.0 f);
GlVertex3f (0.0-1.0 f to 1.0 f, f);
GlVertex3f (0.0 1.0 f to 1.0 f, f);
GlEnd ();
The results are as follows:
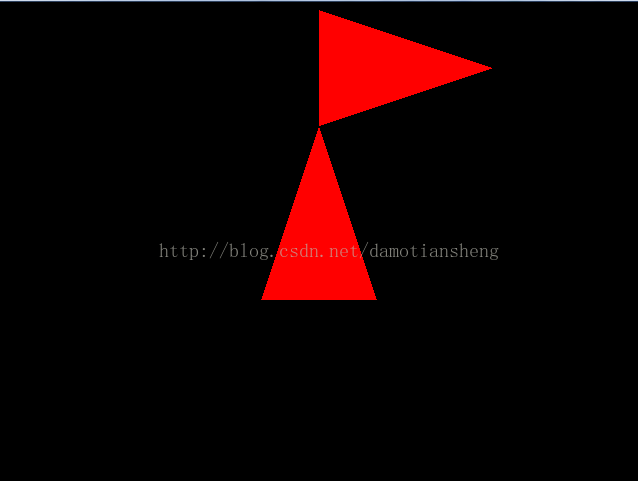
Note: OpenGL uses right multiplication
4. The maximum size of texture map should not exceed 256*256, preferably BMP image
The width and height of the image must be 2 to the power of n; Width and height must be 64 pixels minimum; And for compatibility reasons, the width and height of the image should not exceed 256 pixels. If the width and height of your raw material is not 64,128,256 pixels, use image processing software to resize the image.
I mentioned earlier that there are ways around OpenGL’s restrictions on texture width and height — 64, 128, 256, etc. The solution is gluBuild2DMipmaps. As I’ve found, you can use any bitmap to create a texture. OpenGL will automatically scale it to its normal size.
GluBuild2DMipmaps (GL_TEXTURE_2D, 3, TextureImage [0] – & gt; sizeX, TextureImage[0]-> sizeY, GL_RGB,GL_UNSIGNED_BYTE, TextureImage[0]-> data);
Note: It seems that only BMP images can be loaded, not other types of images
5.
/ *
The purpose is to reset the model observation matrix. If we call glTranslate directly without resetting, unexpected results will occur.
Because the axis has rotated, it’s probably not going in the direction you want. So we wanted to move the object left and right,
Depending on how much Angle you’ve rotated the axis, it might move up and down.
* /
6. Default viewpoint position:
The default viewpoint position is at the point (0,0,0) and the orientation is vertical into the screen (that is, negative z-axis).
When you draw an object, notice that you move the Z coordinate of the object back a few units and you see it.
Viewpoints are not changed by default, which can be done by setting the gllookAt function
7.
glGenTextures(3, & texture[0]); // The glGenTextures function returns n texture indexes based on the texture parameters,& Texture [0] stores the texture index
// Create Nearest filter map
/ *
GlBindTexture actually changes this state of OpenGL by telling OpenGL that any action done to the texture is done to the texture object to which it is bound,
For example, glBindTexture(GL_TEXTURE_2D,1) tells OpenGL that any Settings for 2D textures in the following code are for textures with index 1
* /
8. OpenGL does not set the color, directly draw four vertices, the resulting square, the default will be white;
9.