Click Project — Project Properties;
C/C++ — Linker — System — Subsystem — Parallel — Go to the right drop down menu — Click console — OK;
Tag Archives: C/C++
In vs programming, the code of Ctrl + F5 is flashback
When VS compiles and runs the code, if there is no delay or some kind of response, the window will close immediately after running
Solution a:
in the main function and getchar ()
; add system(” pause “) to the main function
Solution 3:
right-click on the project, select properties, choose the linker, selection system, in the subsystem, the console this
the above methods are debug mode, the way of carrying the shortcut Ctrl + F5
in debug mode, just set a breakpoint in the main function, then one by one to debug
Implementation of OpenGL rotating cube
Topic request
OpenGL is used to implement a rotating cube.
Process steps
It is mainly divided into two parts: setting up the environment and completing the code writing.
Set up the environment
TDM GCC 4.9.2
code>TDM GCC 4.9.2 TDM GCC 4.9.2
TDM GCC 4.9.2
TDM GCC 4.9.2
File - & gt; New - & gt; Project - & gt; Multimedia -> OpenGL
directly compile and run the Demo, which will show a rotating triangle as shown in the image below:
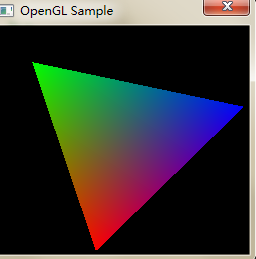
Will be relevant. DLL file copy to the C: \ Windows \ system32 directory
the related. Lib file copy to D: \ \ Program Files \ Dev - Cpp \ MinGW64 \ x86_64 - w64 - mingw32 \ lib32 directory
the related. H file copy to D: \ \ Program Files \ Dev - Cpp \ MinGW64 \ include \ GL directory
the last link is added in the compiler options, Set the appropriate compilation options under Linux and in the Makefile.
Draw cube
void cube()
/ code>void cube() glBegin() and glColor3f() c>>>
glVertex3f() glVertex3f() glVertex3f()glVertex3f()>e>
/code>
void cube()
{
glBegin(GL_QUADS);
glColor3f(1.0,1.0,0.0);
glVertex3f( 1.0, 1.0,-1.0);
glColor3f(0.0,1.0,0.0);
glVertex3f(-1.0, 1.0,-1.0);
glColor3f(0.0,1.0,1.0);
glVertex3f(-1.0, 1.0, 1.0);
glColor3f(1.0,1.0,1.0);
glVertex3f( 1.0, 1.0, 1.0);
glColor3f(1.0,0.0,1.0);
glVertex3f( 1.0,-1.0, 1.0);
glColor3f(0.0,0.0,1.0);
glVertex3f(-1.0,-1.0, 1.0);
glColor3f(0.0,0.0,0.0);
glVertex3f(-1.0,-1.0,-1.0);
glColor3f(1.0,0.0,0.0);
glVertex3f( 1.0,-1.0,-1.0);
glColor3f(1.0,1.0,1.0);
glVertex3f( 1.0, 1.0, 1.0);
glColor3f(0.0,1.0,1.0);
glVertex3f(-1.0, 1.0, 1.0);
glColor3f(0.0,0.0,1.0);
glVertex3f(-1.0,-1.0, 1.0);
glColor3f(1.0,0.0,1.0);
glVertex3f( 1.0,-1.0, 1.0);
glColor3f(1.0,0.0,0.0);
glVertex3f( 1.0,-1.0,-1.0);
glColor3f(0.0,0.0,0.0);
glVertex3f(-1.0,-1.0,-1.0);
glColor3f(0.0,1.0,0.0);
glVertex3f(-1.0, 1.0,-1.0);
glColor3f(1.0,1.0,0.0);
glVertex3f( 1.0, 1.0,-1.0);
glColor3f(0.0,1.0,1.0);
glVertex3f(-1.0, 1.0, 1.0);
glColor3f(0.0,1.0,0.0);
glVertex3f(-1.0, 1.0,-1.0);
glColor3f(0.0,0.0,0.0);
glVertex3f(-1.0,-1.0,-1.0);
glColor3f(0.0,0.0,1.0);
glVertex3f(-1.0,-1.0, 1.0);
glColor3f(1.0,1.0,0.0);
glVertex3f( 1.0, 1.0,-1.0);
glColor3f(1.0,1.0,1.0);
glVertex3f( 1.0, 1.0, 1.0);
glColor3f(1.0,0.0,1.0);
glVertex3f( 1.0,-1.0, 1.0);
glColor3f(1.0,0.0,0.0);
glVertex3f( 1.0,-1.0,-1.0);
glEnd();
}
The cube rotates about its axis
In OpenGL, glRotatef()
nction is used to achieve rotation at a specific Angle. However, to achieve rotation, it is necessary to keep changing the parameters of rotation Angle. Here, we control the rotation of the cube around three axes by setting three static variables and allowing them to increase automatically, and control the rotation speed by changing the increment.
static float xrot = 0.0;
static float yrot = 0.0;
static float zrot = 0.0;
glRotatef(xrot, 1, 0, 0);
glRotatef(yrot, 0, 1, 0);
glRotatef(zrot, 0, 0, 1);
cube();
xrot = xrot + 0.01;
yrot = yrot + 0.01;
zrot = zrot + 0.01;
The cube moves along an axis
glTranslatRef ()
nction Specifies the position of the cube in the 3D coordinate system in OpenGL. Here let’s take the Z axis as an example and let the cube move back and forth, where H is used to control the speed of motion:
static float z=-5;
static float h=-0.001;
glTranslatef(0, 0, z);
z=z+h;
if(z<-10)
h=-h;
if(z>-5)
h=-h;
This completes the simple rotation and axis movement of the cube.
The problem
p>
p>
p>
mainly compiler options. At the same time, references the glut in lib and glut32 lib, and in the compiler options - lglut code>
- lglut32 code> front, the linker when first looking for OPENGL. DLL without looking for opengl32. DLL, at the moment we entered the DEV in c + + project configuration in the face of the changes:
Project - & gt; Project Properties ->; Parameters - & gt; Link
- lopengl32
- lglut32// in front
- lglut
- LGLU
- lglu32
It compiles correctly and runs as expected:
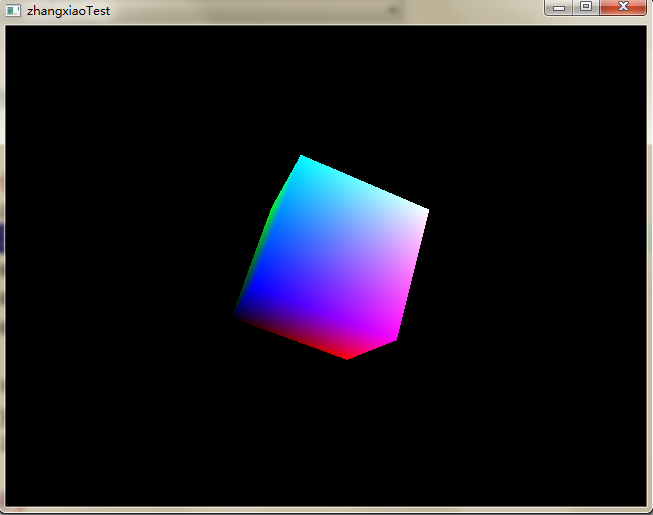
tips
It took a week to finally complete the computer graphics such a simple big job - to achieve the rotation of the cube.
in the code level, is very simple, because of the content is not much involved, and provided a good OpenGL function encapsulation, the Internet has a lot of similar sample.
The most troublesome part is the environment construction, because OpenGL is an open source project, there is no very authoritative manual guide, so the online case is also good and bad, learning efficiency is very low.
in the beginning, for example, to find a project, use the Glaux code>, finally you can compile all that trouble found that can't run, then online and others said the
Glaux basic deprecated code>, and Windows 7 didn't seem to support, had to start from scratch.
Vs series console flashback solution
Consult — Summary — Practice —
Press the red logo to go, perfect solution!
So far, the perfect solution: the principle is not deep research;
Reproduced in: https://www.cnblogs.com/melons/p/5791873.html
VS2010 debugging window flash solution
Take the VS2010 debugging window flash through the solution is as follows:
#include < iostream>
using namespace std;
void main()
int add(int,int,int);
float business (int);
int x, y, z, sum;
cout< < “Input x, y, z:”;
cin> > x> > y> > z;
the sum = add (x, y, z);
cout< <” sum=”< < sum< < endl;
cout< <” average=”< < average(sum)< < endl;
// system (” pause “);
}
}
Int add (int a, int b, int c)
{return (a+b+c); }
float business (int s)
{return (s/3.0); }
This is the test code.
The solution is as follows:
If you are compiling (F5), you can run the program (Ctrl+F5). If it is still flashing, use the following method to solve it.
method one:
1. If the c + + files, in the final to write a program before (return) add: system (” pause “);
= #include”stdlib.h”;
= #include”stdlib.h”; Then add: system(“pause”) at the end of the program (before the return).
Method 2:
. Right click on the current project – Properties
2> 3. Change the SUBSYSTEM configuration in System Options, select the first ‘/ CONSOLE’ in the drop-down menu and then select ‘Start Executing (Don’t Debug)’, that is Ctrl +F5;
This solves the flash window problem once and for all.
Solve the problem of flash back in Visual Studio 2010
Many people will face such a problem, VS2010 flash back, clearly the program is successful, clearly there is no mistake, the lack of a flash at the end.
The flashback problem is primarily a caching problem, which is added at the end of the program, after the main function
getchar();
getchar();
getchar();
getchar();
Solve the problem.
Reproduced in: https://www.cnblogs.com/yijianzhongqing/p/5208854.html
How to configure OpenGL on CodeBlocks
No such file or directory. This error is still present in the C++project. Hey, that’s weird!
The compiler path is set to the path of the NTL library. Now it does not need the NTL library.
Method: the link to delete the NYL library can be
Reproduced in: https://www.cnblogs.com/pualus/p/5900937.html
Solutions to several VTK compilation errors (vtk5.8 + VS2010)
There are several common errors that can occur during the compilation of a VTK project. The solutions are as follows:
error 1:
fatal error C1189: #error : This file requires _WIN32_WINNT to be #defined at least to 0x0403
cause:
The minimum version of the system set for version migration is too low
Visual C++ 2010 no longer supports targeting Windows 95, Windows 98, Windows ME, or Windows NT. If your WINVER or _WIN32_WINNT macros are assigned to one of these versions of Windows, you must modify the macros. When you upgrade a project that was created by using an earlier version of Visual C++, You may see compilation errors related to the WINVER or _WIN32_WINNT macros if they are assigned to a version of Windows that is no longer supported (From MSDN)
The immediate cause – there are three lines of code in atlcore.h:
#if _WIN32_WINNT < 0x0403
#error This file requires _WIN32_WINNT to be #defined at least to 0x0403. Value 0x0501 or higher is recommended.
#endif
> bbb>
All in VTK directory stdafx. H file reference to modify WINVER, http://msdn.microsoft.com/en-us/library/aa383745.aspx _WIN32_WINNT, _WIN32_WINDOWS and _WIN32_IE definition, for example: # define WINVER 0 x0501
error 2:
error C2371: ‘char16_t’ : redefinition
: font>
In VTK project files are generated in the process of choosing the Matlab support (tex-mex), and adopted the VS2010 and above programming environment (http://connect.microsoft.com/VisualStudio/feedback/details/498952/vs2010-iostream-is-incompatible-with-matlab-matrix-h) :
I looked at the source code for matrix.h < core/matlab/include/matrix.h>
It has a definition for char16_t
typedef char16_t char16_t;” . Also the C++ header “Microsoft Visual Studio 10.0\VC\ Include \ YVALS. H “Also defines the same identifier(CHAR16_T). Because of these two definitions we get the redefinition error when matrix iostream.h(it internally includes yvals.h) are included in some cpp file.
Declaration of char16_t in the yvals.h is newly introduced in dev10 and was not there in VS2008. Therefore you are getting this redefinition error now on VS 2010(Dev10).
> solution 1 (without follow-up test) : BBB>
//added by John for solving conflict with VS2010
#if (_MSC_VER) < 1600 //VS2010
# ifndef __STDC_UTF_16__
# ifndef CHAR16_T
# if defined(_WIN32) & & defined(_MSC_VER)
# define CHAR16_T wchar_t
# else
# define CHAR16_T UINT16_T
# endif
typedef CHAR16_T char16_t;
# endif
#endif
#else
#include < yvals.h>
#endif//VS2010
> solution 2 (without follow-up test) : BBB>
Rename all char16_t in matrix.h
error 3:
LNK4199: /DELAYLOAD:vtkIO.dll ignored; no imports found from vtkIO.dll
cause:
DelayLoad (selected from the in-depth analysis DelayLoad “http://blog.csdn.net/panda1987/article/details/5936770).
As we know, there are two basic methods of DLL loading: implicit loading and explicit loading. Implicit loading is the method introduced in the previous article, where the input table of a PE loads the DLL into the memory space before entering the entry function. Explicit loading uses methods like LoadLibrary and GetProAddress to load the DLL into the process space when needed. Both of these methods are the ones we use most often. So what is delay load?
A DLL designated as Delay Load will not actually load the process space until it is needed, which means that the DLL will not be loaded if no function located in the DLL is called. The loading process is done by LoadLibrary and GetProcAddress, which is, of course, transparent to the programmer.
What are the benefits of this?There is no doubt that the program starts faster, because many DLLs may not be used at startup. Even some DLLs may not be used in the entire life cycle, so with Delay Load, the DLL does not need any memory.
> bbb>
Because for dynamic libraries, the load information is still recorded in the corresponding lib file, so in the properties of the project Linker->; Input-> Additional Depandencies may also have #pragma comment(lib, “vtkio. lib”) at the beginning of the Cpp. If the file is not found by the compile prompt, it is also required in the Linder->; General-> Add the location of the file in the Additional Library Directory.
If someone is the difference between the Lib and Dll unclear, see: http://www.cppblog.com/amazon/archive/2011/01/01/95318.html
Reproduced in: https://www.cnblogs.com/johnzjq/archive/2011/12/14/2287823.html
Unhandled exception: 0xc0000005: access violation while reading location 0x00000000
Unhandled exception: 0xC0000005: Access conflict occurred while reading position 0x00000000
In the process of using, there was a mistake in the title, first of all, I searched some methods on the Internet, and finally solved it with a lot of effort.
About 0xC0000005:
0xC0000005: Access Cheesecake error debug —
1 “data out of bounds or defined pointer not freed.
2: Null Pointers are most likely. It is best to explicitly assign values before using Pointers!
should be a pointer problem
3 “memory access error, check the pointer, whether empty, whether out of bounds, etc
1:
ar *p;
p = new char[number];
delete [] p;
…
// always using p….
p = XXX;// access violation
2:
char * p;
memcpy (p, XXX, number); // Access Cheesecake
char *p;
p = new char[number];
delete [] p;
…
delete [] p;// the access violation
http://hi.baidu.com/yuruntsinghua/item/61dfa34e89809793823ae14d
Qt5_ Various paths
1. QT5.3.2 — VS2010 — OpenGL
1.1. The path of the required DLL file at the time of publication
F: \ ZC_software_installDir \ Qt5.3.2 _vs2010\5.3 \ msvc2010_opengl \ bin
1.2, Platforms folder
F: \ ZC_software_installDir \ Qt5.3.2 _vs2010\5.3 \ msvc2010_opengl \ plugins \ platforms
ZC: it feels platforms folder also belong to the plug-in, but seemingly qt. Conf no about its configuration items (have?Is it QLibraryInfo: : PrefixPath?To be tested and verified)…
1.3, libeay32.dll and ssleay32.dll (for SSL access to WebView)
F: \ ZC_software_installDir \ Qt5.3.2 _vs2010 \ Tools \ QtCreator \ bin \ libeay32 DLL
F: \ ZC_software_installDir \ Qt5.3.2 _vs2010 \ Tools \ QtCreator \ bin \ ssleay32 DLL
2,
3,
4,
5,
Reproduced in: https://www.cnblogs.com/cppskill/p/5652216.html
Code programming skills for novice programmers
Programmers aspire to work on teams where they spend 30% of their time writing code and 70% of their time drinking coffee and talking about how to get the product right.
Software work should be a high intellectual activity that integrates technology and art, and the project manager should be a person with a high understanding of the objective laws of quality, scope and schedule. “Work efficiently, live happily” should be the programmer’s motto.
The reality is that the team is overloaded with requirements while fixing endless bugs.
After a little eve, the project manager endure red eyes staring at us all night long overtime, quality specialist urged quality data again and again is not enough, software work has been irreparably reduced to physical labor, let alone happy life, life is gone.
Well, all of the above may be true. Project managers and quality specialists are devils who don’t understand objective laws and have no sympathy, leaving us programmers with no dignity and humble existence.
Just, there is a sentence held for a long time: “wake up, all of this, because your code is too bad, you make too many bugs!” .
You may complain that this is clearly the result of requirements changing too fast and leadership planning being too tight.
Well, that sounds reasonable, but you should know that requirement change itself is the objective law of software, and the leader requires progress, hehe, you can also think of it as an objective law.
This isn’t an argument for who causes programmers to work too much overtime, and I’m not going to give you a recipe for turning everyone into an expert programmer overnight, but at least here are some solid lessons and tips. In short, let everyone see a little more to get a little more real value.
Don’t start writing code right away
You may be in a hurry, or you may be itching to try a little programming trick you learned yesterday. Take it easy, I’m telling you, there are more important things to do before you get the requirements and are ready to write your first line of code.
I can’t stress enough how important this is, but in all of the code I’ve written that I’ve been very happy with, I’ve used this approach, and it has eliminated 90% of the bugs that would otherwise have been tested, or even achieved zero bugs, although it may take a while to get there.
Once you have the requirements, you should first ask yourself if you have fully understood the requirements. Once the answer is yes, we can begin:
1) Find an hour in your busy schedule that you have complete control over, that is your own hour, and that will be free of interruptions or interruptions that will prevent you from implementing this method. Remember that this one hour is very important, more important than all the activities you will perform later, and it is definitely worth it.
2) in the upper part of a piece of paper to write down “the demand characteristics of the normal process and scope of influence”, and then at the bottom of the paper to start writing down the demand characteristics of normal process contains a detailed content, which will probably be used to library function, which will provide the interface, will affect version upgrades, whether affect the resource file, whether to affect the original interface and so on.
3) On the top of the second piece of paper, write down “all the abnormal scenarios of this requirement feature and some mistakes I often made in the past”, and then write down one by one at the bottom of the paper.
4) Keep repeating steps 2) and 3).
You may find it’s not just about writing needs to clarify the material, I want to tell you that this is two different things, it is not a quality specialist activity requires you to do the quality of the process, it is a deep dialogue between you and yourself, it doesn’t need to tell anyone, don’t need any deliverables output to other fields, it is to oneself a self drive to write good code.
At first you may find hard to write a few can’t write out, or flash across the idea of “this stuff is really useful, don’t try so hard, got up and went to the window to play cups of water to drink, breath the fresh air or in short don’t interrupt, unless the fire in the office, don’t let it continue not to do.
As you work your way down to answer number 20 or 30, you might suddenly feel like, “Oh, I found this subtle anomaly. It’s awesome!” This time you will secretly exclaim a little can not contain their excitement, this means that you are close to the success of the completion, each later to write a line will let yourself moved.
Remember, don’t give up in the middle, and your decision to stick with it will turn that one hour into the most important hour of your entire requirement realization.
Second, forget about the damn quality activities behind
All quality activities outside of coding are based on the company’s distrust of your ability to write code.
This means that companies spend a lot of money on quality specialists, meta-testers, solution testers all because of the waste of code that you don’t write well.
Common some developers, just came to the quality specialist arrangement of quality activities quite complain, “my previous company to do projects do not need to do these things is not the same to finish the project”, “these quality activities, is simply the occupation of coding time.
All of this is fine, but isn’t it a bit of a shame when you write code while you’re saying it?These activities are designed by quality specialists to prevent your bad code from rushing to the customer at one checkpoint after another. When you do nothing to “write good code” and only want to cancel these activities, it can only be understood as a gangster.
So, do quality activities “write good code”?
The answer is no.
It is not a goal or even a method. Your goal in writing code is not to meet quality activity standards, but to achieve zero defects, and you do not write good code just because you do good WBIT testing.
One of the things you need to do is “don’t start writing code right away.” The other thing you need to do is learn to refactor as much as you can, the way you think about refactoring, and learn that refactoring isn’t necessarily about refactoring the original code, it’s about knowing how good code is going to be before you write it.
I ask you to forget quality activities, not to let you do not listen to the quality commissioner, but you should write code in the heart of awe, after the code is written all the activities are you caused waste, you have to eliminate these waste.
Remember, you’re writing code for people to see
I heard a colleague tell a chilling story from his last job:
A colleague of his original company left, left is a pile is very complex, see the c + + code can let a person a neurological disorder, he was gone, found the entire project team no one can take over his module, project managers have to high price and a dinner party in a way that he came to tell the person of whole project two days his code. This guy has a “look, only I can fix it” kind of homecoming attitude. I’m curious why the project manager didn’t fire him earlier. He should have called the police.
Good code is pleasing to the eye, any lack of ability or showing skills to increase people’s dyslexia behavior need to be improved, you can be a few words to clarify the context of your own written code, of course, this also involves you to master as many refactoring methods and refactoring way of thinking.
Another measure of self-judgment is to ask yourself, “With all this code you’ve written, have you ever been tempted by it?” Have you ever written the code and read it over and over again, marveling at how beautiful it is?
As a programmer, one of the many happy moments you’ll recall when you leave the company one day will be the feeling that you just produced a piece of code that caught your eye, not the guy who left the company only to realize how important he was.
Start now, practice deliberately
Do you find yourself maintaining a level of “just enough to complete a story” code for a long time, writing code for years and still being chased by testers?
The reason for this confusion is that it doesn’t matter how many years you’ve been coding, it’s all about deliberate practice.
Such as I mentioned earlier, the practice repeatedly, or you figured out the method of decomposition into every link, deliberately to practice, to get a feedback from the test, and constantly improve, you will slowly from a running all day by the tester, to find himself very easy to achieve quality process standards, then slowly you will find you write code tester is more and more difficult to found the problem, the last state better if you could often write zero defect in the code.
We all seem to know some of these things, but I think there are only two steps to understanding them. First, you need to experience them and practice them yourself. Second, you need to be able to communicate them and make them accessible to others.
So the best way to learn is to experience it yourself, and then write about it, so that you can really understand what you thought you knew, but you didn’t. .
If you want some video tutorials, please contact me at 756576218
Reproduced in: https://www.cnblogs.com/nice107/p/8109208.html
Solution of unable to open source file “StdAfx. H” in vs2013 / 2012
As shown in figure:
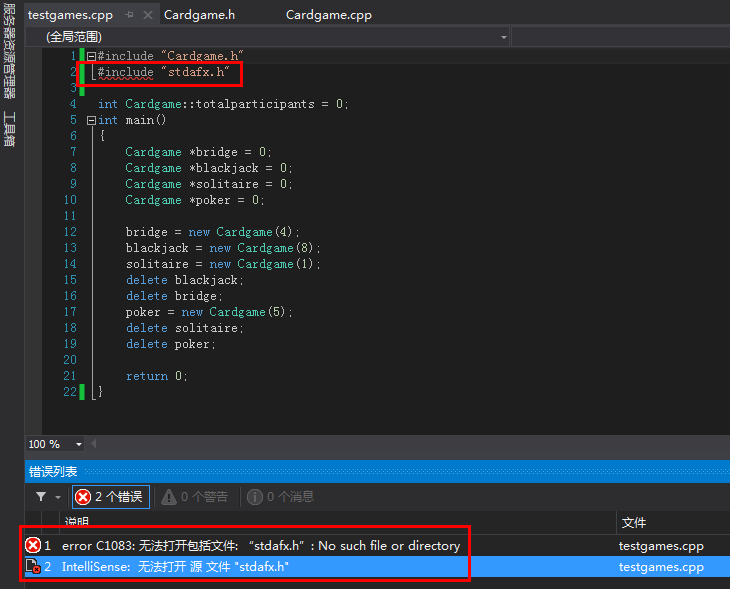
Baidu, for VS2010 there are such methods can be solved:
Expand C/C++ in Project Properties, select General, and add “$(projectDir)” in the Additional Includes directory.
As you can see, it doesn’t work at all in my VS2013.
And it worked:
The precompiled header file is its own, I think VS2013/2012 includes “stdafx.h” by default, so I don’t have to write it again