Recently, I started to plan to learn springcloud-alibaba, so I downloaded the Nacos installation package on the GitHub official website, and found an error on startup.
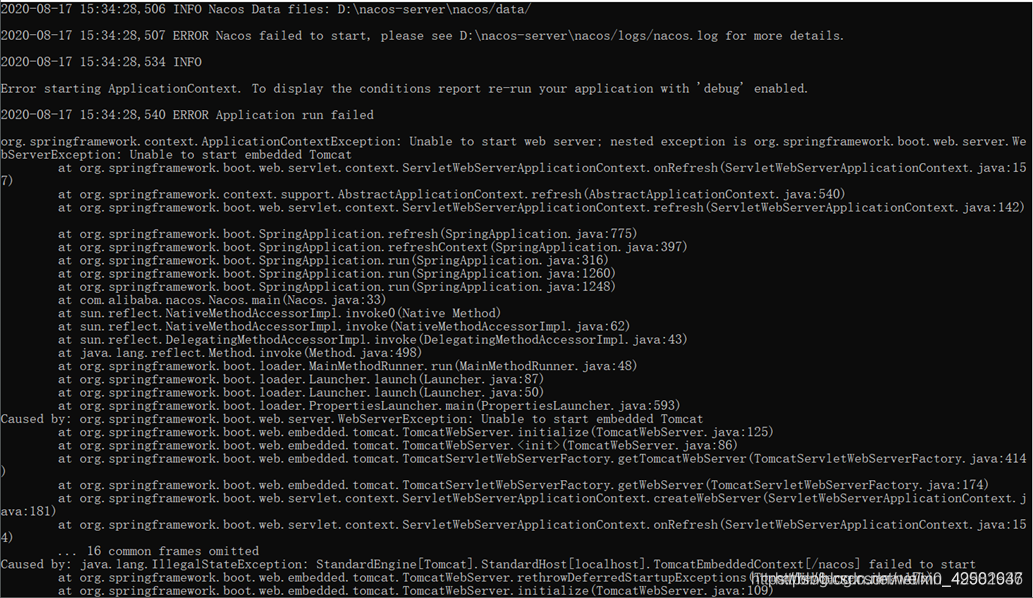
Error message: Unable to start embedded Tomcat
the built-in Tomcat cannot be loaded.
Opened the conf folder and saw a nacos-mysql.sql
It seems to import a database script, so I created a database named nacos in the local database
and executed this sql script, which generated some tables.
With a database and tables, we must change the configuration.
So I opened application.properties with an editor
I saw that there is a place to configure the db, so I changed it.
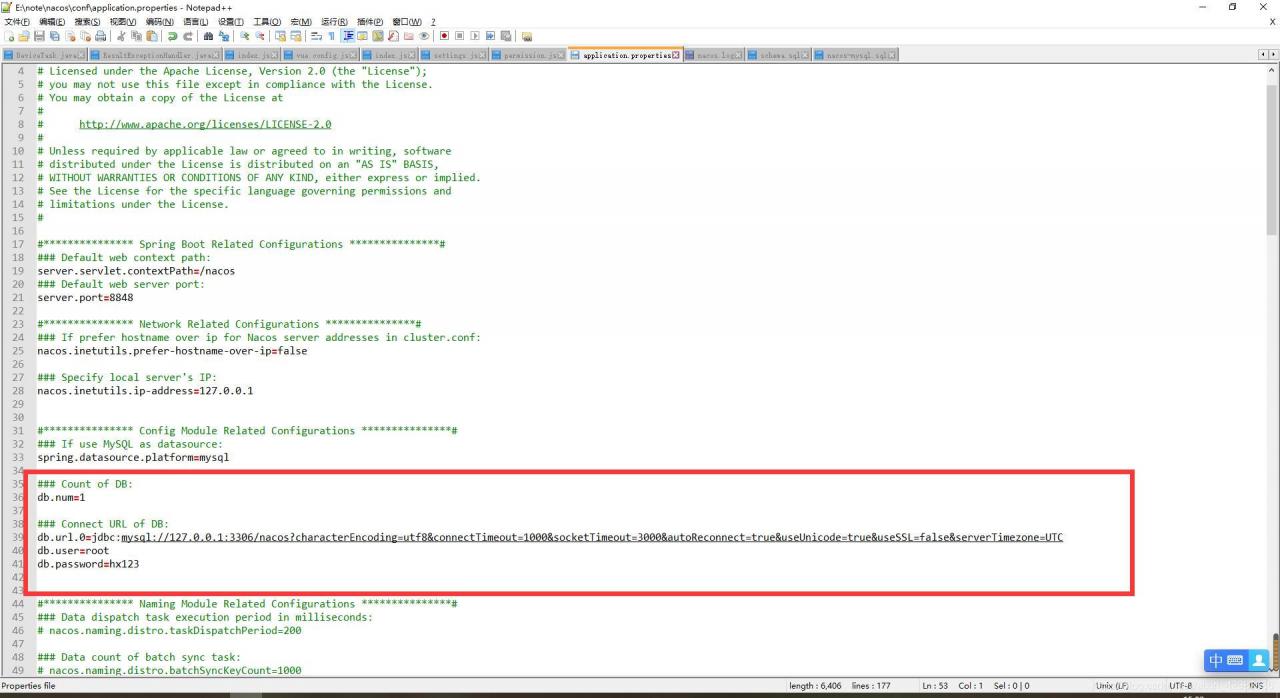
After saving
enter the bin directory again and double-click startbat.cmd to start.
I found that I still reported an error.
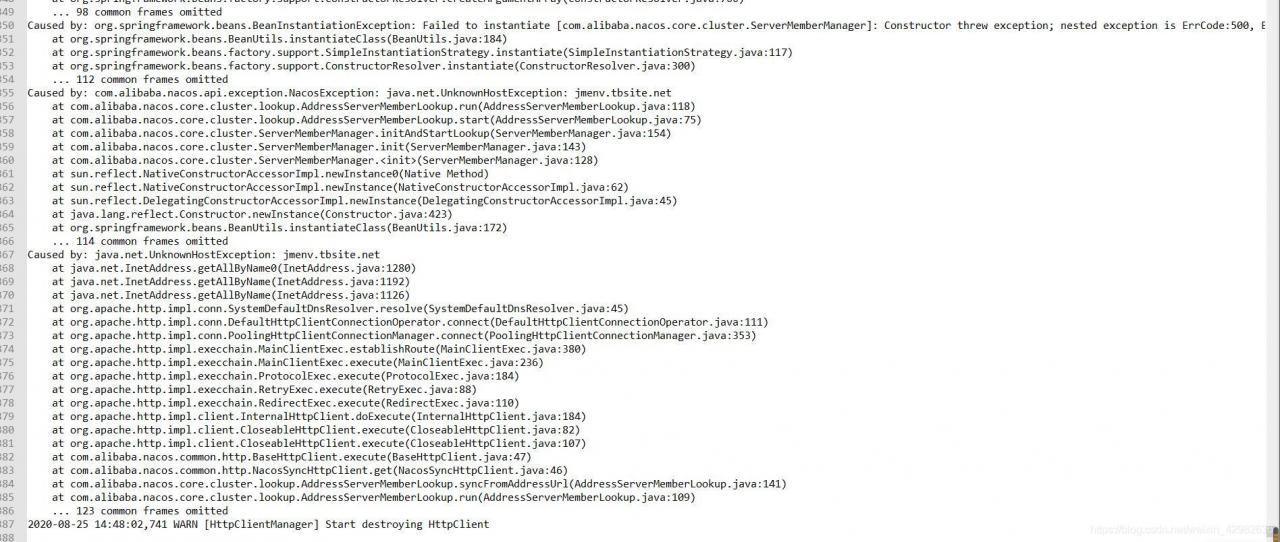
Caused by: java.net.UnknownHostException: jmenv.tbsite.net
Here I have changed the configuration file again, but it has no effect.
The key point is that during startup, I noticed a message,
Nacos has been started in cluster mode. Cluster list is []
I wonder if this is the problem, because I clicked run and did not configure the Nacos cluster.
So I use the editor to open the startup.cmd in the dictory bin
see a key message
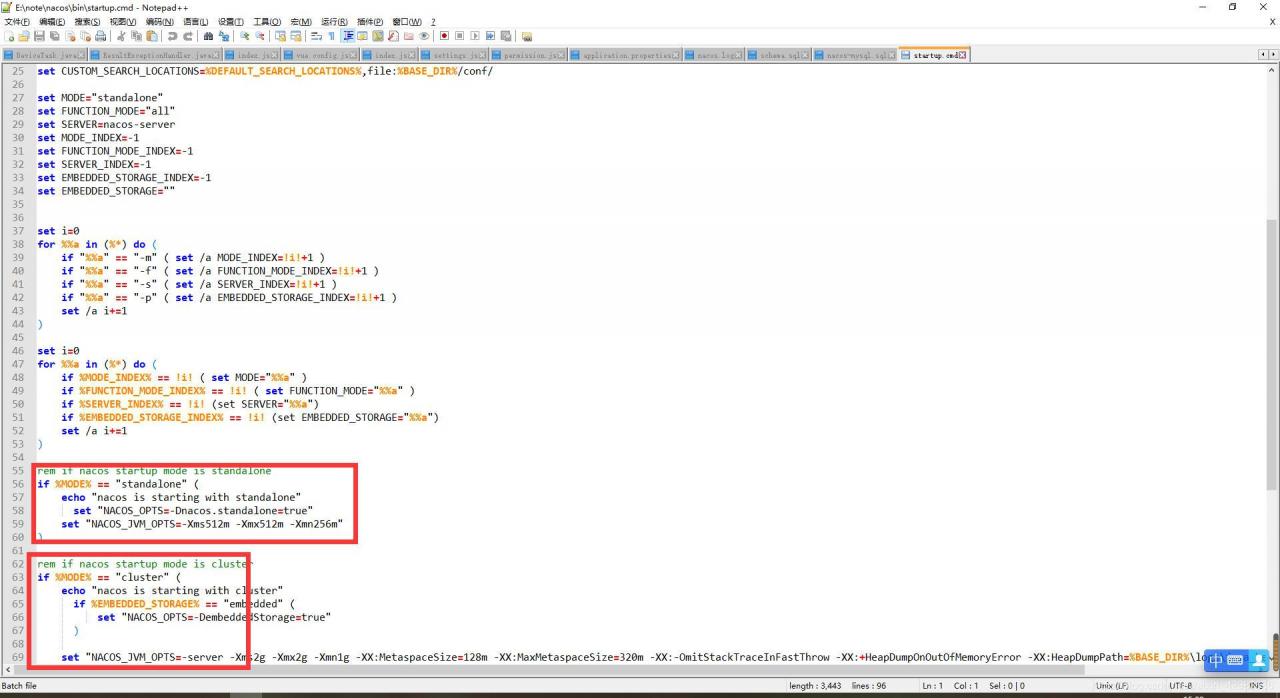
Here you can configure click mode startup, so try to change the startup configuration to click mode startup
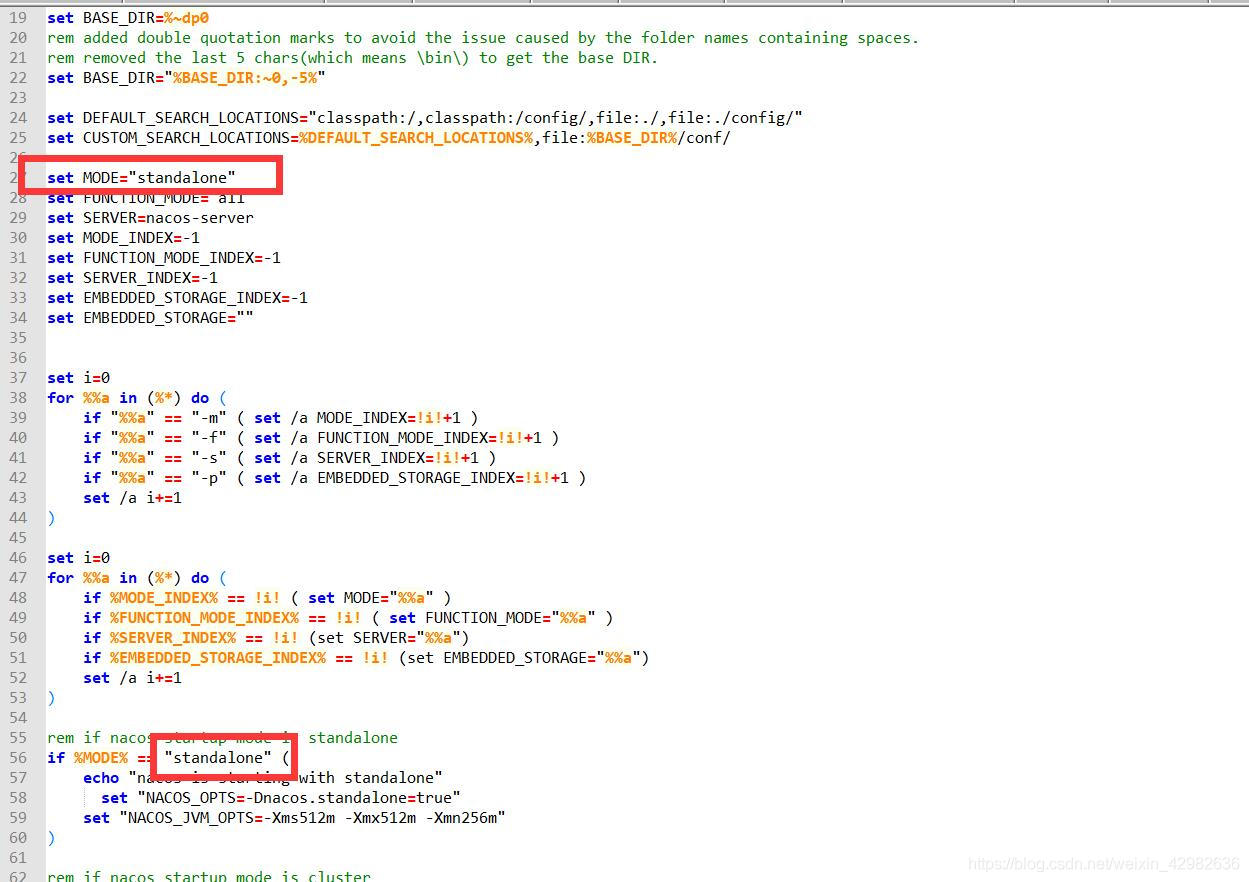
Exit after saving. Double click the startup file startup.cmd
It started normally this time.
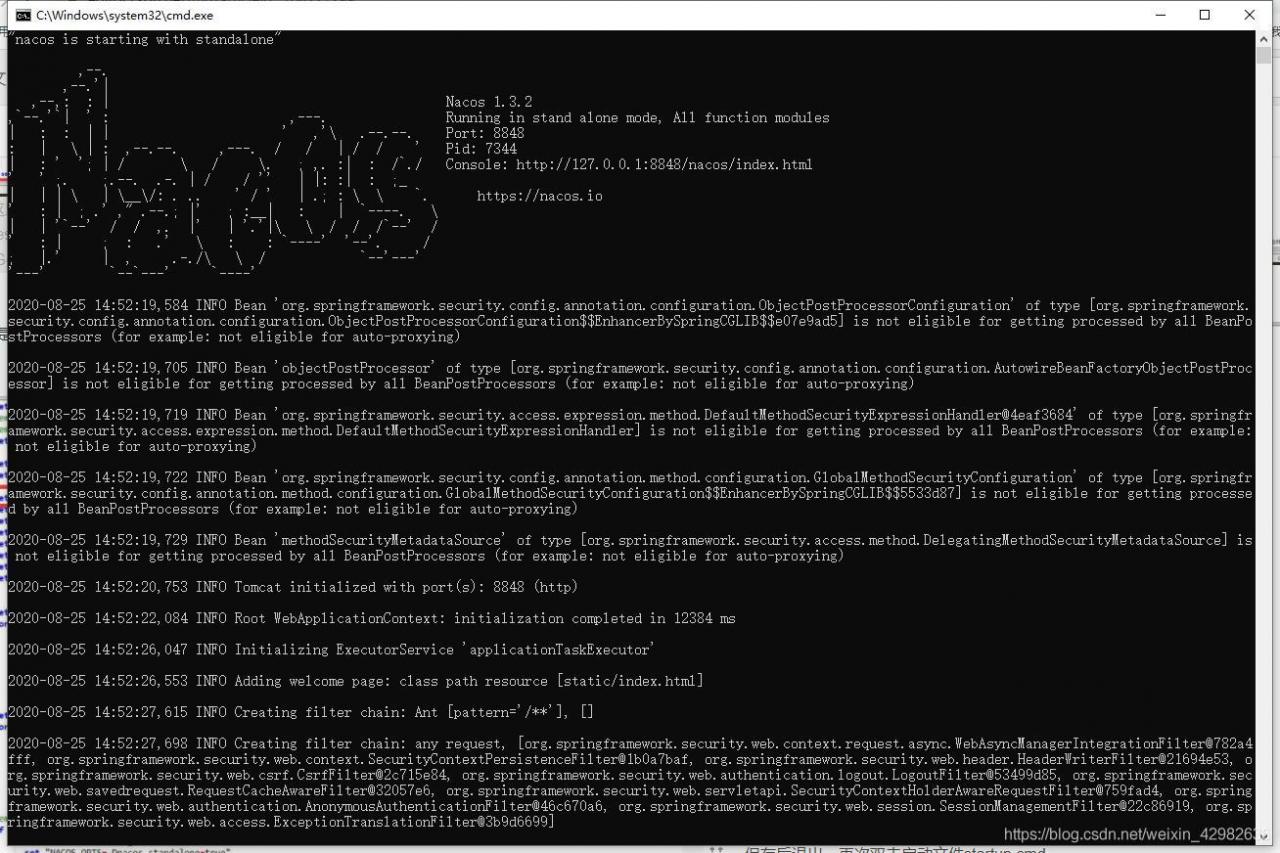
Enter in Browser: localhost:8848/nacos/index.html
Normally access the Nacos configuration center.
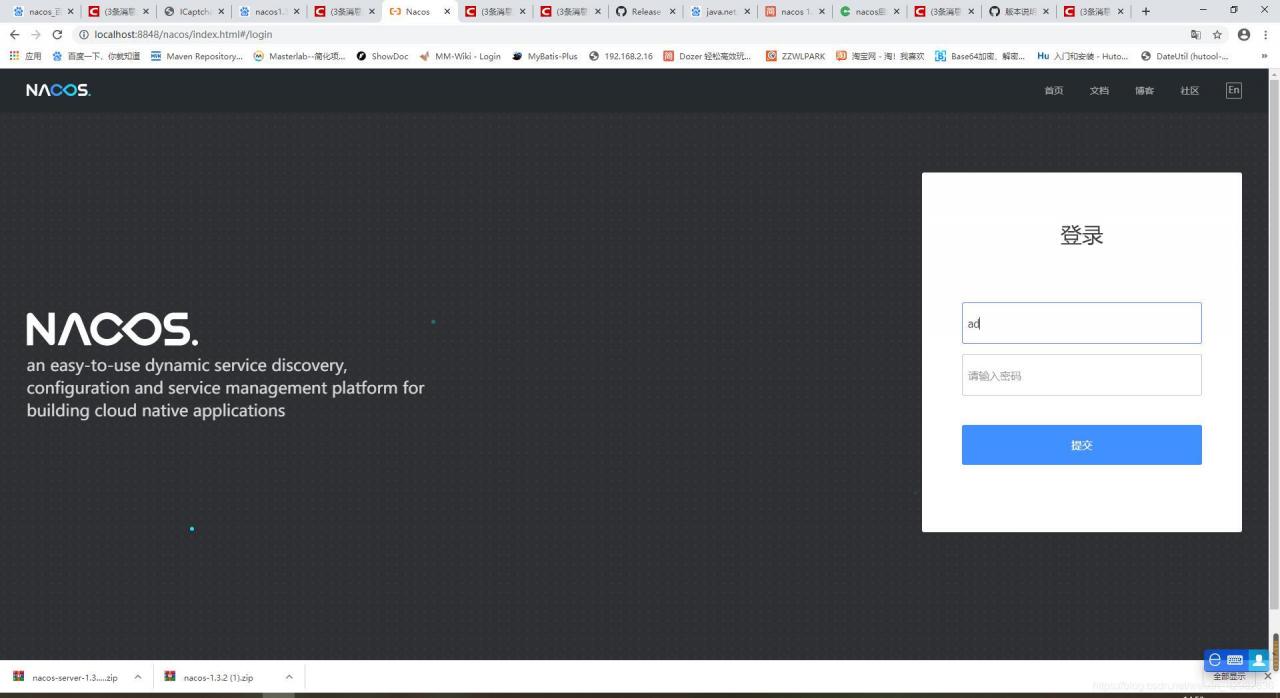