Background:
In order to add jest to the existing projects, many pits have been stepped on. The pits are listed as follows, the adopted solutions, new problems and final treatment methods.
First of all, my project is vue2, and the Babel of package.json is @ Babel, so the following problems will occur. The handling method of this version is to use the CLI plug-in to start jest instead of the Babel version; If it is Babel version, the normal NPM install – G jest configuration script is enough, which will be described later; The jest installation of vue3 is simpler and is not covered in this article.
Pit 1: it runs directly in Vue project and reports an error
requires Babel "^7.0.0-0", but was loaded with "6.26.3". If you are sure you have a compatible version of @babel/core, it is likely that something in your build process is loading the wrong version. Inspect the stack trace of this error to look for the first entry that doesn't mention "@babel/core" or "babel-core" to see what is calling Babe
Tried:
npm install --save-dev "babel-core@^7.0.0-bridge.0"
Various @Babel or Babel are also installed
But it didn’t work. It is speculated that the conflict is caused by jest’s own package.
Pit 2: then upgrade jest to the latest version, 27 +. report errors
TypeError: Cannot read property 'instrument' of undefined
What should I do?It seems that we can’t find a good method on the Internet. Then go to GitHub and see the projects that @ Babel + vue2 + jest can run. Here are the minimum installation items.
Solution:
npm install @vue/test-utils -D
npm install @vue/cli-service -D
npm install @vue/cli-plugin-unit-jest -D
Jest.conf.js is placed in the root directory, or in the tests directory, or whatever you want
module.exports = {
preset: '@vue/cli-plugin-unit-jest',
collectCoverage: false
}
Package.json configuration script and configuration file directory
"scripts": {
"test:unit": "vue-cli-service test:unit --config ./tests/jest.conf.js",
}
If you report an error:
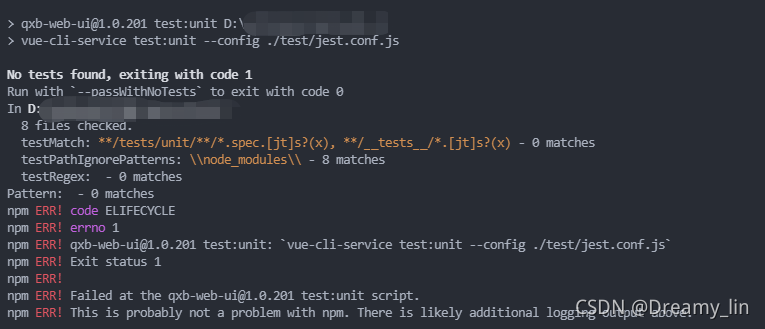
solution: description of the error information. The paths do not match and the test file cannot be found, so change the directory to tests/unit.
Error reporting:
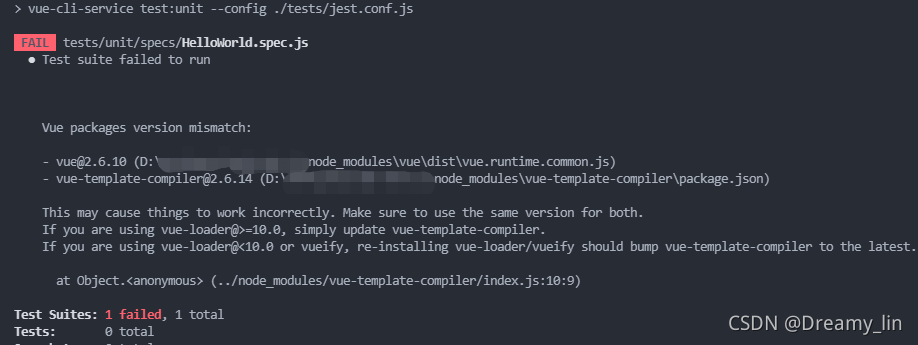
solution: according to the error reporting information, Vue template compiler and Vue need to be the same version, so install the Vue version corresponding to Vue template compiler. My is NPM install Vue template- [email protected]
So far, the unit test is running, and I’m always excited
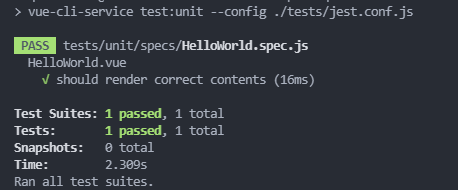
supplement:
My project can run without changing the. Babelrc file
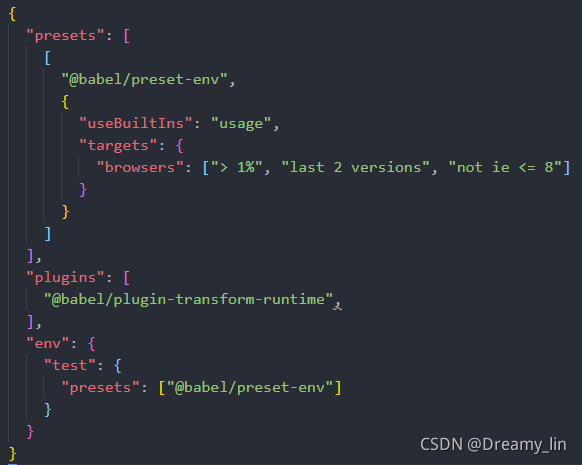
My package.json:
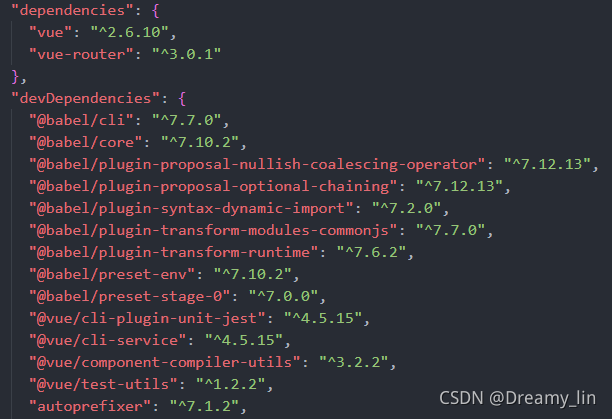
Here, for the installation and configuration of the Babel version of jest, first look at the package. JSON
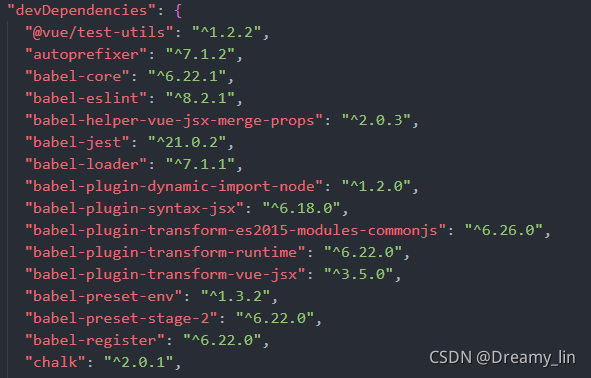

Simply start a project
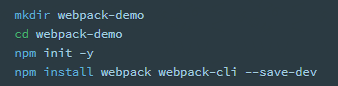
install jest
Global: NPM install – G jest or local: NPM install – D jest
specify the test script in package.json:
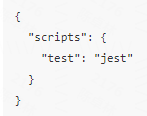
run the script to test.
Finally, for TS, you need to install additional packages and configure additional. If you can’t find it on the Internet, go to GitHub.