Problem Description:
Express JWT is not a function
Cause analysis:
The express JWT I installed is version 7.7.5, and the usage has changed.
https://www.npmjs.com/package/express-jwt
Check the official documents for the latest usage
Solution:
1. import
var { expressjwt:jwt } = require("express-jwt")
2. registration
app.use(jwt({
secret: 'shhhhhhared-secret', algorithms: ['HS256']
}).unless({ path:[/^\/api\//] }))
.unless ({specify the path without access permission with regular})
Or another method, directly add the unique protected path address as a parameter:
app.use("/api", jwt({
secret: "shhhhhhared-secret", algorithms: ["HS256"]
}));
3. user data
Once parsed successfully, the user data contained in the Token will be automatically mounted on req.auth
// This is an API interface with permissions
app.get('/admin/getinfo', function (req, res) {
// TODO_05: use req.auth to get the user information and use the data property to send the user information to the client
console.log(req.auth);
res.send({
status: 200,
message: 'User information retrieved successfully! ,
data: req.auth// the user information to be sent to the client
})
})
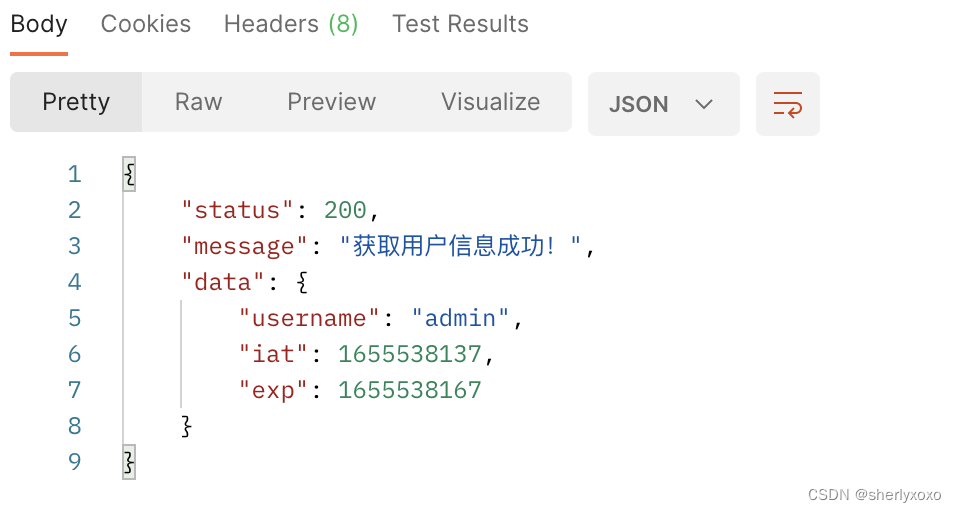