In lerna.json, if the packages attribute is not configured with the right package name, an error will be reported as lerna warn no packages found where npmlog can be added
Tag Archives: front end
React native android: How to Upload Formdata
let resizedImage = file // file
let formData = new FormData();
let name = `xxxx.jpeg`;
let file = { uri: "file:///" + resizedImage.path.split("file:/").join(""), type: 'image/png', name: escape(resizedImage.name), fileType: 'image/\*' }; //The key here (uri and type and name) cannot be changed,
formData.append("file", file); //the files here are the key needed by the backend
formData.append("token", token); //the files here are the key needed by the backend
formData.append("key", Math.random()+'__'+name); //the files here are the keys needed by the backend
Vue project error: browserslist: caniuse Lite is out dated
After modifying the code, restart the project, and the progress card will not move at 69%
and report an error
Browserslist: caniuse-lite is outdated.
Reason: a component has more than one root element (vue2. X)
java.lang.AbstractMethodError:com.mysql.jdbc.Connection.isValid(I)Z
In the actual combat of Java Web project, we encounter the following problems:
This is actually due to the low version of your MySQL driver package https://mvnrepository.com/artifact/mysql
Download the high version of MySQL driver package and put it in lib.
Module build failed: Error: Node Sass version 6.0.0 is incompatible with ^4.0.0.
When we use sass in a project, we need to parse sass into CSS through sass loader. If node sass is not installed, sass loader will not work.
Sometimes, if the node sass version is too high, an error will be reported. The log is as follows:
Module build failed: Error: Node Sass version 6.0.0 is incompatible with ^4.0.0.
terms of settlement:
1. Uninstall the installed version of NPM install node sass
2. Install NPM install [email protected]
3. Restart the project
div>
Ant Design ‘cross env’ is not an internal or external command, nor is it an error reporting problem for a runnable program
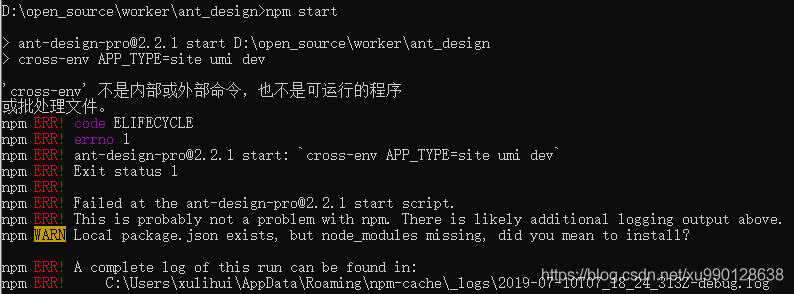
D:\open_source\worker\ant_design>npm start
> [email protected] start D:\open_source\worker\ant_design
> cross-env APP_TYPE=site umi dev
‘cross-env’ is not an internal or external command, nor is it a runnable program
or batch file.
npm ERR! code ELIFECYCLE
npm ERR! errno 1
npm ERR! [email protected] start: `cross-env APP_TYPE=site umi dev`
npm ERR! Exit status 1
npm ERR!
npm ERR! Failed at the [email protected] start script.
npm ERR! This is probably not a problem with npm. There is likely additional logging output above.
npm WARN Local package.json exists, but node_modules missing, did you mean to install?
npm ERR! A complete log of this run can be found in:
npm ERR! C:\Users\AppData\Roaming\npm-cache\_logs\2019-07-10T07_25_09_552Z-debug.log
2. run as administrator, execute the npm install command

Vue introduces ecarts, init initializes and reports an error
Vue introduces ecarts, init initializes and reports an error
1. Download ecarts
npm install echarts --save
2. I have only one page to refer to ecarts, so I directly introduce it into the page
import echarts from 'echarts'
3. Write corresponding examples in methods
myEcharts() {
this.chart = echarts.init(document.getElementById('main'))
this.chart.setOption({
tooltip: {
trigger: 'item',
formatter: '{a} <br/>{b} : {c} ({d}%)'
},
legend: {
bottom: 10,
left: 'center',
data: ['rented', 'not rented', 'booked']
},
series: [
{
name: 'Vehicle Rental Status',
type: 'pie',
radius: '55%',
center: ['50%', '40%'],
data: [
{ value: 40, name: 'rented' },
{ value: 30, name: 'not rented' },
{ value: 30, name: 'booked' }
],
emphasis: {
itemStyle: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: 'rgba(0, 0, 0, 0.5)'
}
}
}
]
})
}
4. Reference method in mounted
mounted() {
this.myEcharts()
}
Results an error was reported, init initialization error was reported
it took half an hour to find out the reason, because the import mode was wrong
the original import accessories from 'accessories'
should be changed to Import * as accessories from' accessories'
. The updated writing method in the official website. After modification, refresh the page
if ecarts is needed in many parts of the system, it can be introduced globally in main.js
import * as echarts from 'echarts'
Vue.prototype.$echarts = echarts
Vue Error: Error in v-on handler (Promise/async): “Error: Error“
The error message is as follows
[Vue warn]: Error in v-on handler (Promise/async): "Error: Error"
found in
---> <Navbar> at src/layout/components/Navbar.vue
<Layout> at src/layout/index.vue
<App> at src/App.vue
<Root>
The solution is as follows
1. F12 enter the developer mode and click the area in the box
2. Check the response code, which is the value on the right side of res.code
3. Add code to easy mock
Node.js operation mysql error Cannot enqueue Handshake after invoking quit
Error Background:
When the database operation is performed for the first time, it is successful. The second time a database operation is performed, an error is reported as shown in the title.
The reason:
This is because after we close the connection with the.end() method, we need to re-call createConnection to recreate a connection.
Solutions:
For example, the following encapsulates a method that operates on database queries and additions. Each time a new connection is created using mysql.createconnection () before the database operation is performed, instead of putting it directly to the outermost layer.
var mysql = require('mysql');
exports.find=function(findSql,callback){
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : '123456',
port: '3306',
database: 'namesharing',
});
connection.connect();
connection.query(findSql,function (err, result) {
if(err){
console.log('[SELECT ERROR] - ',err.message);
return;
}
connection.end();
callback(result)
});
}
exports.insert=function(addSql,addSqlParams,callback){
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : '123456',
port: '3306',
database: 'namesharing',
});
connection.connect();
connection.query(addSql,addSqlParams,function (err, result) {
if(err){
console.log('[INSERT ERROR] - ',err.message);
return;
}
connection.end();
// console.log('INSERT ID:',result);
callback(result)
});
}
Angular_ Error: Cannot assign to a reference or variable!
Cannot assign a value to a reference or variable!
<form class="form-horizontal" (ngSubmit)="login(loginForm.value)" role="form" #loginForm="ngForm">
<div class="form-group">
<label for="username"></label>
<input class="form-control" required name="username" id="username" type="textbox" placeholder="Email"
[(ngModel)]="name" #username="ngModel">
<div *ngIf="username.invalid && (username.dirty || username.touched)" class="alert alert-danger">
<div *ngIf="username.errors.required">
Name is required.
</div>
</div>
</div>
<div class="form-group">
<label for="password"></label>
<input class="form-control" required name="password" id="password" [(ngModel)]="password" type="password"
placeholder="Password" #passWord="ngModel">
<div *ngIf="passWord.invalid && (passWord.dirty || passWord.touched)" class="alert alert-danger">
<div *ngIf="passWord.errors.required">
Name is required.
</div>
<div *ngIf="passWord.errors.minlength">
Name must be at least 4 characters long.
</div>
</div>
</div>
<div class="row" style="margin-top: 40px;">
<div class="col-sm-6">
<!-- <a class="login-link" routerlinkactive="active" ng-reflect-router-link="/register"
ng-reflect-router-link-active="active" href="/register"></a> -->
</div>
<div class="col-sm-6">
<button type="submit" class="btn btn-primary pull-right btn-login" [disabled]="!loginForm.form.valid">
Login</button>
</div>
</div>
</form>
The input #password and the variable defined in the TS file have the same name.
Change can be
Uncaught (in promise) Error: Delete success at __webpack_exports__.default 405 error
Problem description
After deleting a user, you want to get the user list again, but you don’t get it again. You can see that the system reports uncaught (in promise) error: deletion succeeded at webpack_ Exports. Default this error
Problem solving
The reason is that the request is encapsulated in the project, and the response status code is hijacked. Find the processing location of the response in the corresponding project, annotate it and return it to the response directly
Typescript error “Cannot write file xxx because it would overwrite input file
The reason for this problem is that allowjs is turned on.
Because allowjs allows typescript compiler to compile JS. The compiled output file, namely XXX. JS, is the same as the source file.
So an error like “input file will be overwritten” will be reported.
In fact, we use third-party packaging tools such as webpack for our daily development. The compilation output is the responsibility of TS loader, so it is not necessary to care about the output of each TS file.
In this case, noemits can be set to true
true
No Emit –
Do not emit compiler output files like JavaScript source code, source-maps or declarations.
This makes room for another tool like Babel, or swc to handle converting the TypeScript file to a file which can run inside a JavaScript environment.
You can then use TypeScript as a tool for providing editor integration, and as a source code type-checker.
In addition, you can specify the output directory to avoid conflicts.
For more details, please refer to https://github.com/kulshekhar/ts-jest/issues/1471