Manifest merge failed with multiple errors, see logs solution
In component-based development, when testing a single component, such problems sometimes occur in compilation
Simulate with my project:
The current test is lib_news
lib_news requires webView of lib_web
lib_news build gradle
if (isRelease) {
apply plugin: 'com.android.library'
} else {
apply plugin: 'com.android.application'
}
apply plugin: 'org.jetbrains.kotlin.android'
apply plugin: 'kotlin-android'
apply plugin: 'kotlin-kapt'
apply plugin: 'kotlin-parcelize'
android{
……
defaultConfig {
……
kapt {
arguments {
arg("AROUTER_MODULE_NAME", project.getName())
}
}
buildConfigField("boolean", "isRelease", String.valueOf(isRelease))
}
sourceSets{
main {
if (isRelease) {
//if library,then compile AndroidManifest.xml in manifest
manifest.srcFile 'src/main/manifest/AndroidManifest.xml'
} else {
//if application, then compile theAndroidManifest.xml in the home directory.
manifest.srcFile 'src/main/AndroidManifest.xml'
}
}
}
……
dependencies{
……
implementation project(path: ':lib_web') //import lib_web
}
}
project config.gradle
ext{
isRelease = false
//isUseWeb = true Comment first, the general configuration file is only configured with isRelease
……
}
lib_web build.gradle
if (isRelease) {
apply plugin: 'com.android.library'
} else {
apply plugin: 'com.android.application'
}
apply plugin: 'org.jetbrains.kotlin.android'
apply plugin: 'kotlin-android'
apply plugin: 'kotlin-kapt'
apply plugin: 'kotlin-parcelize'
android{
……
defaultConfig {
……
kapt {
arguments {
arg("AROUTER_MODULE_NAME", project.getName())
}
}
buildConfigField("boolean", "isRelease", String.valueOf(isRelease))
}
sourceSets{
main {
if (isRelease) {
manifest.srcFile 'src/main/manifest/AndroidManifest.xml'
} else {
manifest.srcFile 'src/main/AndroidManifest.xml'
}
}
}
……
dependencies{
……
}
}
In general, we only set isrelease
to determine whether a single component test or integrated compilation
so the test lib_news
, directly change isrelease
to false
, and also lib_web
will also become Application
, which is equivalent to us in lib_news
importing Application
instead of Library
, will involve the problem of androidmanifest.xml
Show me androidmanifest.xml
lib_web
component mode
Lib_ Web
integration mode
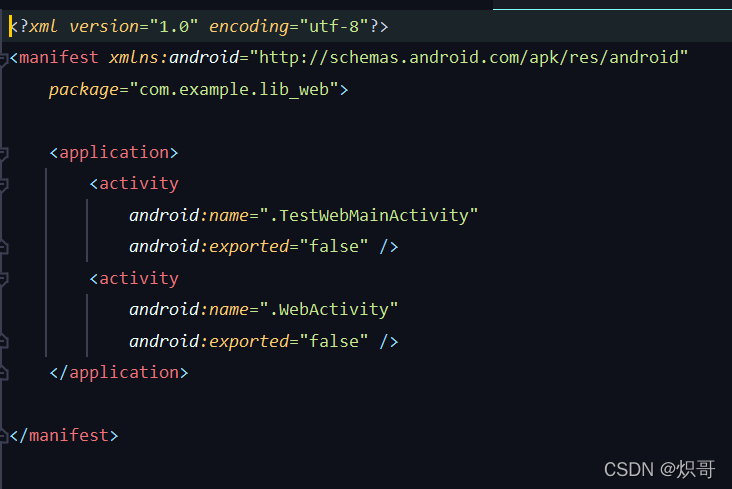
Solution:
project config.gradle
add a isuseweb
to identify the lib_web to be used in the componentization mode
ext{
isRelease = false
isUseWeb = true
……
}
Modify lib_web
build.gradle
if (isUseWeb) {
apply plugin: 'com.android.library'
} else {
apply plugin: 'com.android.application'
}
android {
……
defaultConfig {
……
buildConfigField("boolean", "isUseWeb", String.valueOf(isUseWeb))
}
……
sourceSets{
main {
if (isUseWeb) {
manifest.srcFile 'src/main/manifest/AndroidManifest.xml'
} else {
manifest.srcFile 'src/main/AndroidManifest.xml'
}
}
}
}