An error is reported when installing the go plug-in for vscode under Windows:
...
Installing golang.org/x/tools/gopls@latest FAILED
{
"killed": false,
"code": 1,
"signal": null,
"cmd": "D:\\Program Files\\Go\\bin\\go.exe install -v golang.org/x/tools/gopls@latest",
"stdout": "",
"stderr": "go install: golang.org/x/tools/gopls@latest: module golang.org/x/tools/gopls: Get \"https:///goproxy.cn/golang.org/x/tools/gopls/@v/list\": http: no Host in request URL\n"
}
...
Most people can’t go to GitHub, but I can go up here. The report here is no host
after a lot of queries, it is found that most of them are agents, and then go to GitHub to download them, and then execute them. I still report an error after downloading
the solution is recorded here.
Method 1: update the environment variable and configure the download agent address
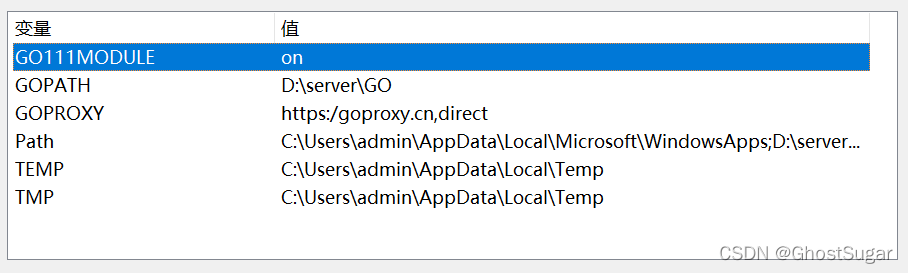
Or open PowerShell and enter:
$env:GO111MODULE="on"
$env:GOPROXY="https://goproxy.io"
go env -w GOPROXY=https://goproxy.cn,direct
//go env -w GOPROXY=https://goproxy.io,direct
go env -w GOPRIVATE=*.corp.example.com
goproxy.IO and goproxy.Cn is OK
Executing the following command in the terminal will output go Evn configuration
go env
set GO111MODULE=on
set GOARCH=amd64
set GOBIN=D:\Program Files\Go\bin
set GOCACHE=C:\Users\admin\AppData\Local\go-build
set GOENV=C:\Users\admin\AppData\Roaming\go\env
set GOEXE=.exe
set GOEXPERIMENT=
set GOFLAGS=
set GOHOSTARCH=amd64
set GOHOSTOS=windows
set GOINSECURE=
set GOMODCACHE=D:\server\GO\pkg\mod
set GONOPROXY=*.corp.example.com
set GONOSUMDB=*.corp.example.com
set GOOS=windows
set GOPATH=D:\server\GO
set GOPRIVATE=*.corp.example.com
set GOPROXY=https://goproxy.io
set GOROOT=D:\Program Files\Go
set GOSUMDB=sum.golang.org
set GOTMPDIR=
set GOTOOLDIR=D:\Program Files\Go\pkg\tool\windows_amd64
set GOVCS=
set GOVERSION=go1.17.5
set GCCGO=gccgo
set AR=ar
set CC=gcc
set CXX=g++
set CGO_ENABLED=1
set GOMOD=NUL
set CGO_CFLAGS=-g -O2
set CGO_CPPFLAGS=
set CGO_CXXFLAGS=-g -O2
set CGO_FFLAGS=-g -O2
set CGO_LDFLAGS=-g -O2
set PKG_CONFIG=pkg-config
set GOGCCFLAGS=-m64 -mthreads -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=C:\Users\admin\AppData\Local\Temp\go-build957788953=/tmp/go-build -gno-record-gcc-switches
Note that gopath is the dependent package directory of go, and goroot is the installation directory of go
If it is executed in the vscode terminal, the update will not be seen until the terminal is closed and then output. Only restart the vscode terminal, and the configuration displayed here will not change!
Then, Ctrl + Shift + P in vscode to call up the search term, enter go install, select go: Install/update tools, and then select all to execute
Here I see that many people have successfully implemented it, but I don’t think it will work.
Method 2: manually download the plug-in project (clone)
Create a folder in the% gopath% Directory:
Src/golang org/x/
Open the terminal and switch to% gopath%/SRC/golang Under org/X/directory, execute clone:
cd $env:GOPATH/src/golang.org/x/
git clone https://github.com/golang/tools.git
git clone https://github.com/golang/lint.git
My% gopath% directory is D:\server\go, and the current directory is as follows:
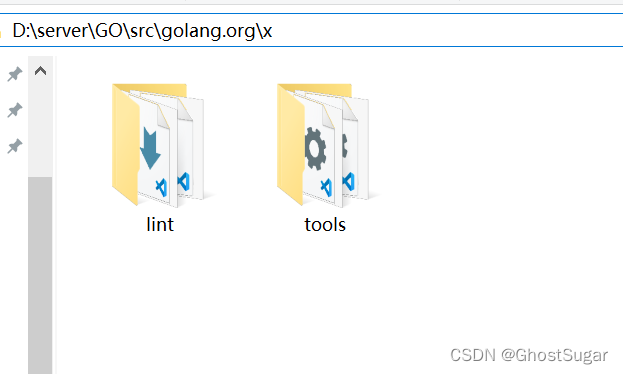
Then switch to% gopath%\SRC\golang. In the terminal Org\x\
go install -v golang.org/x/tools/gopls
But I still failed here. Hint:
PS D:\server\GO\src> go install -v golang.org/x/tools/gopls
go install: version is required when current directory is not in a module
Try 'go install golang.org/x/tools/gopls@latest' to install the latest version
After adding the @latest version, the installation succeeded:
PS D:\server\GO\src> go install -v golang.org/x/tools/gopls@latest
...
PS D:\server\GO\src> go install -v github.com/haya14busa/goplay/cmd/goplay@latest
...
PS D:\server\GO\src> go install -v github.com/go-delve/delve/cmd/dlv@latest
...
PS D:\server\GO\src> go install -v github.com/uudashr/gopkgs/v2/cmd/gopkgs@latest
PS D:\server\GO\src> go install -v github.com/cweill/gotests/gotests@latest
PS D:\server\GO\src> go install -v github.com/fatih/gomodifytags@latest
PS D:\server\GO\src> go install -v github.com/josharian/impl@latest
PS D:\server\GO\src> go install -v github.com/haya14busa/goplay/cmd/goplay@latest
In front of this is the directory used, followed by GitHub COM, because my local error is vs automatic installation, I reported both addresses, so I tried to install both
above.