Problems encountered
A very old C++ project, before the compilation was all right, just after I introduced a few new.h and.cpp files, the compilation failed to pass, reported the following error:
(On the premise, of course, that there is no problem with the new files I introduced being double-checked, otherwise I would have started with those files and there would have been no article.)
Error 2 error LNK2005: "void * __cdecl operator new(unsigned int)" (??2@YAPAXI@Z) already defined in LIBCMTD.lib(new.obj)
C:\Work\Demo\DemoApplication\nafxcwd.lib(afxmem.obj) Sentinel-XP
1>------ Build started: Project: DemoApplication, Configuration: Debug Win32 ------
1> text.cpp
1>gbk.obj : warning LNK4075: ignoring '/EDITANDCONTINUE' due to '/SAFESEH' specification
1>nafxcwd.lib(afxmem.obj) : error LNK2005: "void * __cdecl operator new(unsigned int)" (??2@YAPAXI@Z) already defined in LIBCMTD.lib(new.obj)
1>nafxcwd.lib(afxmem.obj) : error LNK2005: "void __cdecl operator delete(void *)" (??3@YAXPAX@Z) already defined in LIBCMTD.lib(dbgdel.obj)
1>nafxcwd.lib(afxmem.obj) : error LNK2005: "void __cdecl operator delete[](void *)" (??_V@YAXPAX@Z) already defined in LIBCMTD.lib(delete2.obj)
1>Debug\DemoApplication.exe : fatal error LNK1169: one or more multiply defined symbols found
========== Build: 0 succeeded, 1 failed, 0 up-to-date, 0 skipped ==========

Problem analysis
There is no such thing as a gratuitous compilation error. After an extensive search, there is an explanation on MSDN that I think is very good. Here is an excerpt:
The CRT libraries use weak external linkage for the new, delete, and DllMain functions. The MFC libraries also contain new, delete, and DllMain functions. These functions require the MFC libraries to be linked before the CRT library is linked.
I will not translate it into Chinese, I am lazy, and I believe everyone can understand this sentence.
This way, we give the MSDN link: https://support.microsoft.com/en-us/kb/148652
To solve the problem
In this article on the MSDN: https://msdn.microsoft.com/en-us/library/72zdcz6f.aspx
The following sentence was given:
To fix, add /FORCE:MULTIPLE to the linker command line options, and make sure that ... is the first library referenced.
is just like this:
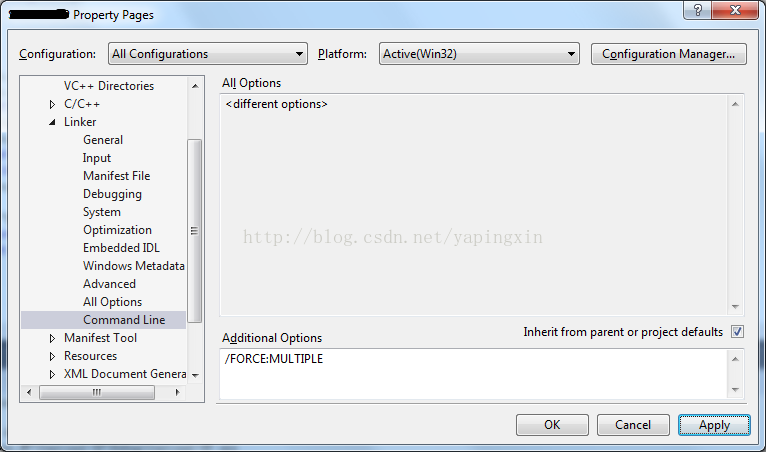
Then it was compiled. Although there were some warnings, the compilation passed:
1>------ Build started: Project: DemoApplication, Configuration: Debug Win32 ------
1> ...
1> ...
1> Compiling...
1> ...
1> Generating Code...
1>LINK : warning LNK4075: ignoring '/INCREMENTAL' due to '/FORCE' specification
1>gbk.obj : warning LNK4075: ignoring '/EDITANDCONTINUE' due to '/OPT:LBR' specification
1>nafxcwd.lib(afxmem.obj) : warning LNK4006: "void * __cdecl operator new(unsigned int)" (??2@YAPAXI@Z) already defined in LIBCMTD.lib(new.obj); second definition ignored
1>nafxcwd.lib(afxmem.obj) : warning LNK4006: "void __cdecl operator delete(void *)" (??3@YAXPAX@Z) already defined in LIBCMTD.lib(dbgdel.obj); second definition ignored
1>nafxcwd.lib(afxmem.obj) : warning LNK4006: "void __cdecl operator delete[](void *)" (??_V@YAXPAX@Z) already defined in LIBCMTD.lib(delete2.obj); second definition ignored
1>Debug\DemoApplication.exe : warning LNK4088: image being generated due to /FORCE option; image may not run
1> DemoApplication.vcxproj -> C:\Work\Demo\DemoApplication\Debug\DemoApplication.exe
========== Build: 1 succeeded, 0 failed, 0 up-to-date, 0 skipped ==========
works fine.
Of course, this is not the root of the problem, but given the old project document, I can’t easily change it too much, so let’s leave it as an imperfect solution.
reference
A LNK2005 error occurs when the CRT library and the MFC libraries are linked in the wrong order in Visual c + + https://support.microsoft.com/en-us/kb/148652
would Tools error LNK2005 https://msdn.microsoft.com/en-us/library/72zdcz6f.aspx
selectany https://msdn.microsoft.com/en-us/library/5tkz6s71 (v = versus 80). Aspx
LNK2005, “Already defined error” would error in MSVC2010 http://stackoverflow.com/questions/8343303/lnk2005-already-defined-error-linker-error-in-msvc2010