1. When using the Android Room database, the following error occurs:
Schema export directory is not provided to the annotation processor so we cannot export the schema. You can either provide room.schemaLocation
annotation processor argument OR set exportSchema to false.
2. There are two kinds of solutions.
1). Set the exportSchema annotation to false for RoomDatabase. default is true
@Database(entities = [TableItem::class], version = 1,exportSchema = false)
abstract class AppDataBase : RoomDatabase() {
abstract fun tableItemDao(): TableItemDao
2) Add in the build.gradle of the app (recommended)
There are different configuration syntaxes for java environment and kotlin environment. Depending on the personal environment configuration, only one way of writing can exist for both. My environment is a kotlin environment, so use the kotlin environment writing method. At the beginning, I used the java environment writing method, and the result kept reporting errors, and only after changing to kotlin did it work.
defaultConfig {
applicationId "XXX"
minSdkVersion 21
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
//Specify the path to the file generated by room.schemaLocation, java environment (choose one of the two, depending on the project environment)
javaCompileOptions {
annotationProcessorOptions {
arguments = ["room.schemaLocation": "$projectDir/schemas".toString()]
}
}
//Specify the path to the file generated by room.schemaLocation, kotlin environment (choose one of the two, depending on the project environment)
kapt {
arguments {
arg("room.schemaLocation", "$projectDir/schemas")
}
}
}
3. After configuration and compilation, JSON structure files of data will be generated in the following directory:
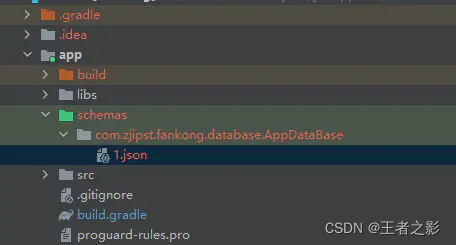