Python+OpenCV:How to Use Background Subtraction Methods
Background subtraction (BS) is a common and widely used technique for generating a foreground mask (namely, a binary image containing the pixels belonging to moving objects in the scene) by using static cameras. As the name suggests, BS calculates the foreground mask performing a subtraction between the current frame and a background model, containing the static part of the scene or, more in general, everything that can be considered as background given the characteristics of the observed scene.
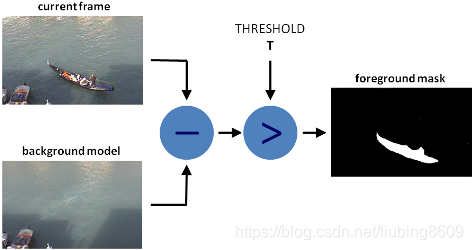
Background modeling consists of two main steps:
Background subtraction (BS) is a common and widely used technique for generating a foreground mask (namely, a binary image containing the pixels belonging to moving objects in the scene) by using static cameras. As the name suggests, BS calculates the foreground mask performing a subtraction between the current frame and a background model, containing the static part of the scene or, more in general, everything that can be considered as background given the characteristics of the observed scene.
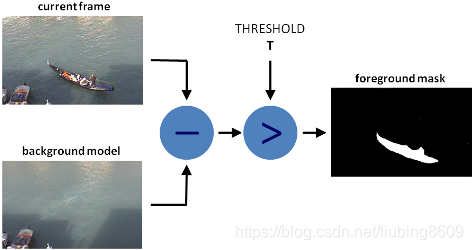
Background modeling consists of two main steps:
- Background Initialization;Background Update.
In the first step, an initial model of the background is computed, while in the second step that model is updated in order to adapt to possible changes in the scene.
Background Subtraction in OpenCV
####################################################################################################
# 图像背景减法(Background Subtraction Methods)
def lmc_cv_background_subtraction():
"""
Function: Background Subtraction Methods.
""""
# Read the vide
parser = argparse.ArgumentParser(description='This program shows how to use background subtraction methods\
provided by OpenCV. You can process both videos and images.')
parser.add_argument('--input', type=str, help='Path to a video or a sequence of image.',
default='D:/99-Research/Python/Image/box.mp4')
parser.add_argument('--algo', type=str, help='Background subtraction method (KNN, MOG2).', default='MOG2')
args = parser.parse_args()
# A lmc_cv::BackgroundSubtractor object will be used to generate the foreground mask.
if args.algo == 'MOG2':
back_sub = lmc_cv.createBackgroundSubtractorMOG2()
else:
back_sub = lmc_cv.createBackgroundSubtractorKNN()
# A lmc_cv::VideoCapture object is used to read the input video or input images sequence.
capture = lmc_cv.VideoCapture(lmc_cv.samples.findFileOrKeep(args.input))
if not capture.isOpened:
print('Unable to open: ' + args.input)
exit(0)
while True:
ret, frame = capture.read()
if frame is None:
break
# Every frame is used both for calculating the foreground mask and for updating the background.
fg_mask = back_sub.apply(frame)
# get the frame number and write it on the current frame
lmc_cv.rectangle(frame, (10, 2), (100, 20), (255, 255, 255), -1)
lmc_cv.putText(frame, str(capture.get(lmc_cv.CAP_PROP_POS_FRAMES)), (15, 15),
lmc_cv.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 0))
# show the current frame and the ForeGround masks
lmc_cv.imshow('Original Frame', frame)
lmc_cv.imshow('ForeGround Mask', fg_mask)
# quit
keyboard = lmc_cv.waitKey(30)
if keyboard == 'q' or keyboard == 27:
break
return
Read More:
- [Solved] cv2.error: OpenCV(4.6.0) D:\a\opencv-python\opencv-python\opencv\modules\……
- Python: How to Costomize the Background of PS
- [Solved] opencv-python: recipe for target ‘modules/python3/CMakeFiles/opencv_python3.dir/all‘ failed
- [Solved] python opencv Error: findContours() Can only use the Grayscale
- Opencv-python Install is Stuck Error: running setup.py bdist_wheel for opencv-python
- How to Solve Opencv Reads Video Error: cv2.error
- Opencv Python realizes the paint filling function in PS, one click filling color and the possible reasons for opencv’s frequent errors
- Python: How to Find the square root and square of numbers (Several methods)
- Python: How to Use os.path.join()
- Python: How to Use try exception to Display Abnormal Error Information
- [Solved] Pyinstaller package opencv error: ImportError: OpenCV loader: missing configuration file: [‘config.py’]. Check OpenCV installation.
- RuntimeWarning: overflow encountered in ubyte_Scalars pixel addition and subtraction overflow exception
- Opencv: How to Draw Palette
- How to Solve Python WARNING: Ignoring invalid distribution -ip (e:\python\python_dowmload\lib\site-packages)
- [Solved] ERROR: Failed building wheel for opencv-python-headless
- How to Fix Errors encountered in executing Python scripts with command line parameters
- Ab3dmot vehicle pedestrian recognition (How to Use)
- Python Use PIP to install pyinstaller Error [Solved]