For example, you can use “Speech_Recognition” for your certificate using Python. For example, you can use “Speech_Recognition” for your certificate using Python. For example, you can use “Speech_Recognition” for your certificate using Python instead of using “username+ PWD”
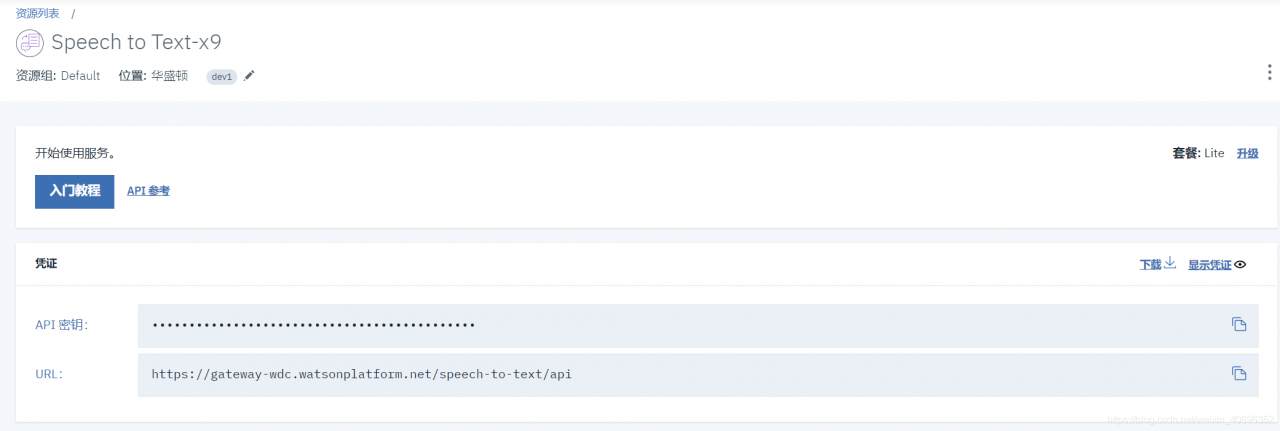
Using the curl command, you can use the URL to convert audio to text.
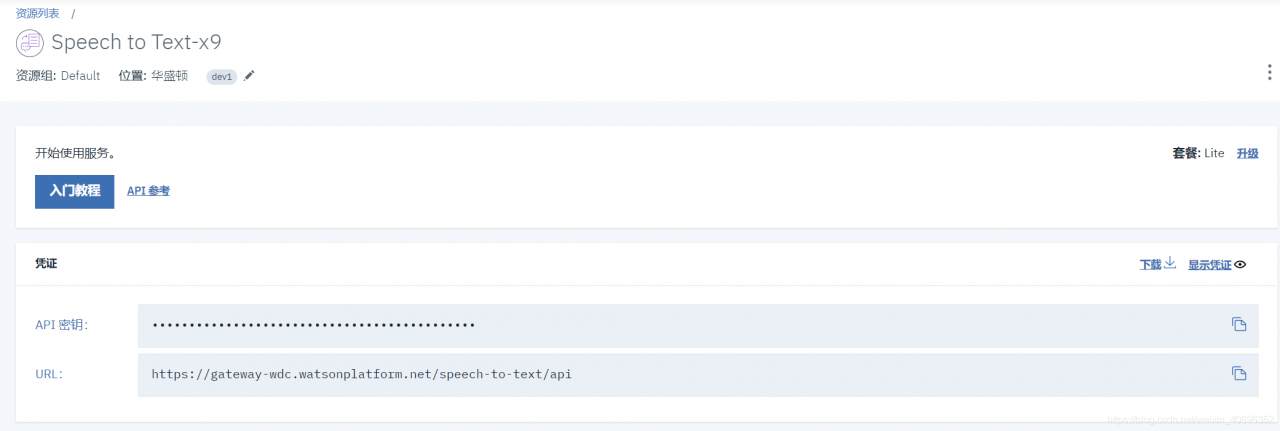
Using the curl command, you can use the URL to convert audio to text.
curl -X POST -u "apikey:{apikey}" --header "Content-Type: audio/flac" --data-binary @{path_to_file}audio-file.flac "{url}/v1/recognize"
To do this, you need to use the curl command to do something that you want to do. To do this, you need to do something that you want to do. To do this, you need to do something that you want to do.
import requests
headers = {
'Content-Type': 'audio/flac',
}
data = open('audio-file.flac', 'rb').read()
r = requests.post('https://gateway-wdc.watsonplatform.net/speech-to-text/api/v1/recognize', headers=headers, data=data, auth=('apikey', '***************************'))
print(r.text)
Test effect:
This doesn’t even need to pack well….. It’s OK to request the interface directly. Finally, attach the original version of the code to call the interface implementation method:
import speech_recognition as sr
import requests
harvard = sr.AudioFile('23.wav')
r = sr.Recognizer()
with harvard as source:
audio = r.record(source)
print(type(audio))
IBM_USERNAME = '************************'
IBM_PASSWORD = '************************'
text = r.recognize_google(audio, username= IBM_USERNAME, password = IBM_PASSWORD, language = 'zh-CN')
print(text)
Read More:
- Resolve Audio: failed to create voice ` goldfish_ Audio ‘error
- An error is reported when starting the Android emulator: Emulator: audio: Failed to create voice `goldfish_audio_in’
- [HTML] Python extracts HTML text to TXT
- Python error TypeError:can ‘t convert complex to float
- A method of generating subtitle file for MP3
- Latex: How to Set text Center
- How to convert iconfont to PNG transparent image
- How to Fix Spring Boot OTS parsing error: Failed to convert WOFF 2.0
- How to Use ffmpeg to convert MP4 and other formats to MP3 format
- How to align MathType formula with text in word
- python cx_Oracle.DatabaseError: Error while trying to retrieve text for error ORA-01804
- Failed to install realtek HD audio driver Code:0xE0000247
- Solve the audio control and report domexception: the play() request was interrupted by a call to pause in the chrome console
- Parsing the difference between “R” and “RB” patterns of text files (Python)
- Error: failed to find conversion function from unknown to text
- Python implements inserting content in the specified position of text
- Solve the problem of unable to locate package python3.6 when upgrading from python3.5 to python3.6 in ubantu16.04
- Summary of three methods for pandas to convert dict into dataframe
- How to import Python from relative path
- Tensorflow ValueError: Failed to convert a NumPy array to a Tensor