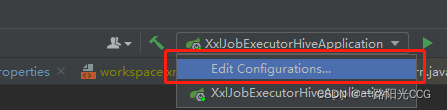
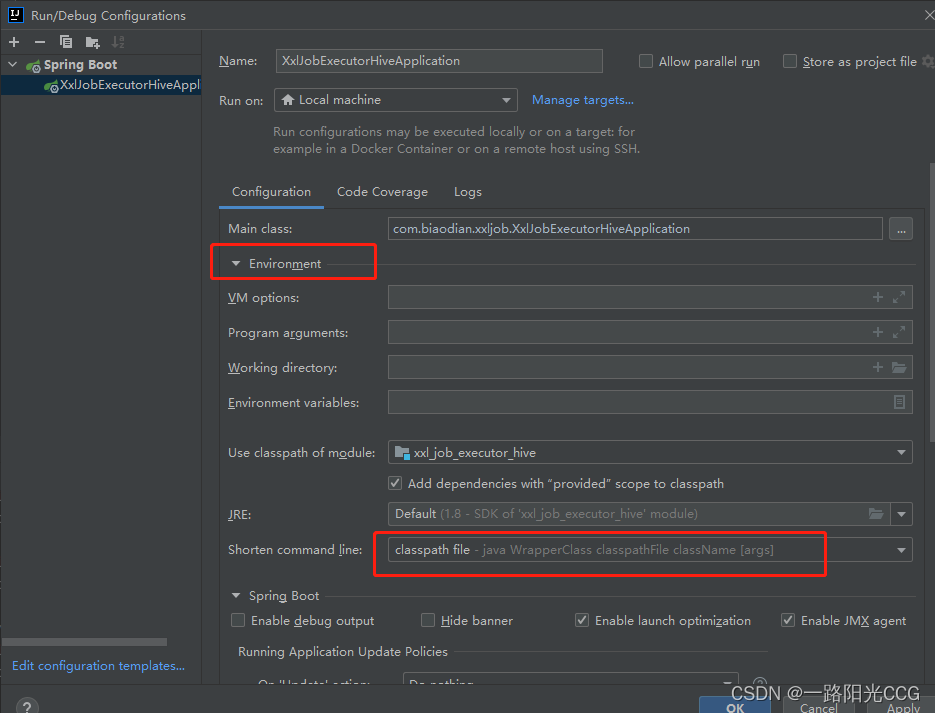
Description:
Field elasticsearchRestTemplate in cn.lili.modules.material.serviceImpl.QrMaterialServiceImpl required a bean of type ‘org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate’ that could not be found. The injection point has the following annotations: - @org.springframework.beans.factory.annotation.Autowired(required=true) Action: Consider defining a bean of type ‘org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate’ in your configuration. Process finished with exit code 1
Solution:
Add lazy loading annotation: @Lazy
Background error reporting problem log
2021-12-30 15:41:24.675 WARN [nio-9008-exec-1] [] i.s.m.p.AbstractSerializableParameter [421] : Illegal DefaultValue 1024 for parameter type integer
java.lang.NumberFormatException: For input string: ""
at java.lang.NumberFormatException.forInputString(NumberFormatException.java:65)
at java.lang.Long.parseLong(Long.java:601)
at java.lang.Long.valueOf(Long.java:803)
at io.swagger.models.parameters.AbstractSerializableParameter.getExample(AbstractSerializableParameter.java:412)
at sun.reflect.GeneratedMethodAccessor149.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at com.fasterxml.jackson.databind.ser.BeanPropertyWriter.serializeAsField(BeanPropertyWriter.java:689)
at com.fasterxml.jackson.databind.ser.std.BeanSerializerBase.serializeFields(BeanSerializerBase.java:755)
Troubleshooting
In abstractserializableparameter.getexample (abstractserializableparameter.java:412) break point debugging. You can find that the value of example is an empty string and execute long Valueof (this.example) will throw ‘Java.lang.numberformatexception’ exception
Solution:
1. modify source code
2. Download the source code and change the if (this.example = = null) judgment to if (this.example = = null | example.isempty())
3. adjust dependency (recommended)
4. Exclude swagger models of swagger2 and add swagger models with version 1.5.21
<!-- Illegal DefaultValue null for parameter type integer -->
<dependency>
<groupId>io.swagger</groupId>
<artifactId>swagger-models</artifactId>
<version>1.5.21</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.10.5</version>
<scope>compile</scope>
<exclusions>
<exclusion>
<groupId>io.swagger</groupId>
<artifactId>swagger-models</artifactId>
</exclusion>
</exclusions>
</dependency>
After adjustment, you can see that the new version has modified the decision logic
after the above operations, no error will be reported when accessing the swagger document address.
Question
When starting the springboot service, the service reports an error. The specific error information is as follows:
Description:
The Bean Validation API is on the classpath but no implementation could be found
Action:
Add an implementation, such as Hibernate Validator, to the classpath
reason:
Dependency in POM
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>7.0.0.Final</version>
</dependency>
And
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
<version>2.2.6.RELEASE</version>
</dependency>
Conflict.
Solution:
The dependency is deleted because it is not used in the service
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>7.0.0.Final</version>
</dependency>
Restart the service normally.
Tomcat does not publish the jar package added by Maven dependency when publishing the project
Solution:
eclipse project right click -> properties -> Deployment Assembly -> Add -> Java Build Path Entries -> select Maven Dependencies -> Apply -> Finish -> it’s OK
This will publish the corresponding Maven dependencies to tomcat, and tomcat will be able to find the dependencies
Special case: tomcat service (Servers) has more than one project, will also report such an error, the project does not need to start to remove can be
Solution:
Since the Dubbo framework is used, on the premise that we confirm that there is no problem with JUnit unit test environment:
First, check whether the Dubbo service is enabled; Secondly, if the test class is written in the consumer module, you need to check whether the provider service is enabled;
Start the corresponding service to solve the problem.
Detailed error reporting information is as follows:
[main] ERROR org. springframework. test. context. TestContextManager – Caught exception while allowing TestExecutionListener [org.springframework.test.context.support. DependencyInjectionTestExecutionListener@333398f ] to prepare test instance [com.yangdaxian.test. MyTest@103c97ff ]
Some detailed error messages are as follows:
19:51:44.947 [main] ERROR org.springframework.test.context.TestContextManager - Caught exception while allowing TestExecutionListener [org.springframework.test.context.support.DependencyInjectionTestExecutionListener@333398f] to prepare test instance [com..test.MyTest@103c97ff]
java.lang.IllegalStateException: Failed to load ApplicationContext
at org.springframework.test.context.cache.DefaultCacheAwareContextLoaderDelegate.loadContext(DefaultCacheAwareContextLoaderDelegate.java:125) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.DefaultTestContext.getApplicationContext(DefaultTestContext.java:108) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.DependencyInjectionTestExecutionListener.injectDependencies(DependencyInjectionTestExecutionListener.java:118) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.DependencyInjectionTestExecutionListener.prepareTestInstance(DependencyInjectionTestExecutionListener.java:83) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.TestContextManager.prepareTestInstance(TestContextManager.java:246) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.junit4.SpringJUnit4ClassRunner.createTest(SpringJUnit4ClassRunner.java:227) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.junit4.SpringJUnit4ClassRunner$1.runReflectiveCall(SpringJUnit4ClassRunner.java:289) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12) [junit-4.12.jar:4.12]
at org.springframework.test.context.junit4.SpringJUnit4ClassRunner.methodBlock(SpringJUnit4ClassRunner.java:291) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.junit4.SpringJUnit4ClassRunner.runChild(SpringJUnit4ClassRunner.java:246) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.junit4.SpringJUnit4ClassRunner.runChild(SpringJUnit4ClassRunner.java:97) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) [junit-4.12.jar:4.12]
at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) [junit-4.12.jar:4.12]
at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) [junit-4.12.jar:4.12]
at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) [junit-4.12.jar:4.12]
at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) [junit-4.12.jar:4.12]
at org.springframework.test.context.junit4.statements.RunBeforeTestClassCallbacks.evaluate(RunBeforeTestClassCallbacks.java:61) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.junit4.statements.RunAfterTestClassCallbacks.evaluate(RunAfterTestClassCallbacks.java:70) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.junit.runners.ParentRunner.run(ParentRunner.java:363) [junit-4.12.jar:4.12]
at org.springframework.test.context.junit4.SpringJUnit4ClassRunner.run(SpringJUnit4ClassRunner.java:190) [spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.junit.runner.JUnitCore.run(JUnitCore.java:137) [junit-4.12.jar:4.12]
at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:69) [junit-rt.jar:?]
at com.intellij.rt.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:33) [junit-rt.jar:?]
at com.intellij.rt.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:221) [junit-rt.jar:?]
at com.intellij.rt.junit.JUnitStarter.main(JUnitStarter.java:54) [junit-rt.jar:?]
Caused by: org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'newsController': Injection of @DubboReference dependencies is failed; nested exception is java.lang.IllegalStateException: Failed to check the status of the service com..service.NewsService. No provider available for the service com..service.NewsService from the url dubbo://192.168.66.1/com.yangziqiang.service.NewsService?application=demo-consumer&dubbo=2.0.2&init=false&interface=com.yangziqiang.service.NewsService&metadata-type=remote&methods=count1,listNews,showMsg&pid=17984®ister.ip=192.168.66.1&release=2.7.10&side=consumer&sticky=false×tamp=1640001099664 to the consumer 192.168.66.1 use dubbo version 2.7.10
at com.alibaba.spring.beans.factory.annotation.AbstractAnnotationBeanPostProcessor.postProcessPropertyValues(AbstractAnnotationBeanPostProcessor.java:149) ~[spring-context-support-1.0.10.jar:?]
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.populateBean(AbstractAutowireCapableBeanFactory.java:1400) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean(AbstractAutowireCapableBeanFactory.java:592) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean(AbstractAutowireCapableBeanFactory.java:515) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.AbstractBeanFactory.lambda$doGetBean$0(AbstractBeanFactory.java:320) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton(DefaultSingletonBeanRegistry.java:222) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:318) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:199) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons(DefaultListableBeanFactory.java:849) ~[spring-beans-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization(AbstractApplicationContext.java:877) ~[spring-context-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:549) ~[spring-context-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.AbstractGenericContextLoader.loadContext(AbstractGenericContextLoader.java:128) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.AbstractGenericContextLoader.loadContext(AbstractGenericContextLoader.java:60) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.AbstractDelegatingSmartContextLoader.delegateLoading(AbstractDelegatingSmartContextLoader.java:275) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.support.AbstractDelegatingSmartContextLoader.loadContext(AbstractDelegatingSmartContextLoader.java:243) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.cache.DefaultCacheAwareContextLoaderDelegate.loadContextInternal(DefaultCacheAwareContextLoaderDelegate.java:99) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
at org.springframework.test.context.cache.DefaultCacheAwareContextLoaderDelegate.loadContext(DefaultCacheAwareContextLoaderDelegate.java:117) ~[spring-test-5.1.5.RELEASE.jar:5.1.5.RELEASE]
... 24 more
Caused by: java.lang.IllegalStateException: Failed to check the status of the service
Error running ‘Tomcat: run’: cannot run program “Tomcat: run” (in directory “D: \ myideaproject \ springmvc \ spring \ Web”): CreateProcess error = 2, the system cannot find the specified file.
reason:
You need to use the MVN Tomcat: run command.
The following problems may occur during operation
The reason may be that there is a problem with Maven’s default Tomcat. The solution is to add a Tomcat plug-in
Add the following codes to pom.xml
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<configuration>
<port>8080</port>
<path>/</path>
</configuration>
</plugin>
Then start with the MVN tomcat7: run command
When uploading files, use multipartfile Transferto() saves the file to the local path:
report errors:
java.io.IOException: java.io.FileNotFoundException: C:\Users\XXXXX\AppData\Local\Temp\tomcat. 8350081478984499756.8080\work\Tomcat\localhost\ROOT\app\file\xxxx. Xlsx (the system cannot find the specified path.)
@Override
public String store(MultipartFile file, String fileName) throws IOException {
String destPath "/app/file/";
File filePath = new File(destPath);
File dest = new File(filePath, fileName);
if (!filePath.exists()) {
filePath.mkdirs();
}
try {
file.transferTo(dest);
log.info("file save success");
} catch (IOException e) {
log.error("File upload Error: ", e);
throw e;
}
return dest.getCanonicalPath();
}
Cause analysis:
file. When the transferto method is called, it is judged that if it is a relative path, the temp directory is used as the parent directory
so it is saved in the temporary work directory of Tomcat.
Solution:
Use absolute path: filepath.getAbsolutePath()
@Override
public String store(MultipartFile file, String fileName) throws IOException {
String destPath "/app/file/";
File filePath = new File(destPath);
// Convert to absolute path
File dest = new File(filePath.getAbsolutePath(), fileName);
if (!filePath.exists()) {
filePath.mkdirs();
}
try {
file.transferTo(dest);
log.info("file save success");
} catch (IOException e) {
log.error("File upload Error: ", e);
throw e;
}
return dest.getCanonicalPath();
}
Supplement:
You can also file Getbytes() gets the byte array, or file Getinputstream() performs stream data operation and writes it to disk.
Access to uploaded files
spring:
resources:
static-locations: file:/app/file/ #Access external system resources and map the files in this directory to the system
or
import java.io.File;
import java.util.concurrent.TimeUnit;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.CacheControl;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
public void addResourceHandlers(ResourceHandlerRegistry registry) {
String absolutePath = new File("/app/file/").getAbsolutePath();
registry.addResourceHandler("/upload/**") // External Access Addresses
.addResourceLocations("file:" + absolutePath)// SpringBoot needs to add the file protocol prefix
.setCacheControl(CacheControl.maxAge(30, TimeUnit.MINUTES));// set the browser cache
}
}
preface
When configuring multiple environment files for springboot, you need to configure multiple YML files. The configuration before version 2.4 is as follows:
spring:
profiles:
active: dev
But if SpringBoot’s version 2.4 later, it’s a reward.
org.springframework.boot.context.config.InvalidConfigDataPropertyException: Property ‘spring.profiles.active[0]’ imported from location ‘class path resource [application-work.yml]’ is invalid in a profile specific resource [origin: class path resource [application-work.yml] - 9:7]
Solution:
General layout changes as follows:
spring:
config:
activate:
on-profile:
- dev
Of course, it can also be solved by returning the spring boot version to before 2.4
Swagger reported an error, IllegalStateException
I encountered a novel mistake today. Take a note.
Because of the project problem, I imported the swagger dependency into the common module. Then, because common is introduced into the gateway, check the dependency tree and confirm that the gateway also introduces the swagger dependency by default.
Due to the introduction of a problem, an error is reported when starting the gateway. The error is as follows:
java.lang.IllegalStateException: Failed to introspect Class [com.tang.config.SwaggerConfiguration] from ClassLoader [sun.misc.Launcher$AppClassLoader@18b4aac2]
at org.springframework.util.ReflectionUtils.getDeclaredMethods(ReflectionUtils.java:481)
at org.springframework.util.ReflectionUtils.doWithMethods(ReflectionUtils.java:358)
at org.springframework.util.ReflectionUtils.getUniqueDeclaredMethods(ReflectionUtils.java:414)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory
Caused by: java.lang.NoClassDefFoundError: springfox/documentation/spring/web/plugins/Docket
at java.lang.Class.getDeclaredMethods0(Native Method)
at java.lang.Class.privateGetDeclaredMethods(Class.java:2701)
at java.lang.Class.getDeclaredMethods(Class.java:1975)
at org.springframework.util.ReflectionUtils.getDeclaredMethods(ReflectionUtils.java:463)
... 33 common frames omitted
Caused by: java.lang.ClassNotFoundException: springfox.documentation.spring.web.plugins.Docket
at java.net.URLClassLoader.findClass(URLClassLoader.java:382)
at java.lang.ClassLoader.loadClass(ClassLoader.java:424)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:349)
at java.lang.ClassLoader.loadClass(ClassLoader.java:357)
... 37 common frames omitted
Because the error occurred after the swagger dependency was introduced. So I preliminarily determined that the error occurred in the two swagger dependencies I imported.
<!-- swagger -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
</dependency>
After querying the boss on stackoverflow, the answer is that swagger needs web dependency when it is started. If it does not exist, an error will be reported. I tried to add and found
A respectful error is reported on the console:
**********************************************************
Spring MVC found on classpath, which is incompatible with Spring Cloud Gateway at this time. Please remove spring-boot-starter-web dependency.
**********************************************************
Because it was an error found in the gateway. Therefore, it is obvious that the spring cloud gateway integrates weblux and thus the web. Therefore, adding spring-boot-starter-web
to the project will cause the above error.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-gateway-server</artifactId>
<version>2.2.7.RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
<version>2.3.8.RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-loadbalancer</artifactId>
<version>2.2.7.RELEASE</version>
<scope>compile</scope>
<optional>true</optional>
</dependency>
Solution:
Because the gateway in the project does not need to integrate swagger, when introducing the basic module package with swagger in the gateway configuration, it only needs to be in POM XML settings <exclusions></exclusions>
<exclusions>
<exclusion>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
</exclusion>
<exclusion>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
</exclusion>
</exclusions>
Finally, because the related configuration classes of swagger are also written in the basic module. An error will also be reported because the swagger dependency is not imported. At this time, you only need to ignore the injection in the gateway gateway startup class.
@ComponentScan(excludeFilters = @ComponentScan.Filter(type = FilterType.ASSIGNABLE_TYPE, value = SwaggerConfiguration.class))
Solution:
Reason: the data returned by the method called remotely is not added with @ResponseBody
Original:
After modification:
HttpMediaTypeNotSupportedException: Content type ‘application/json;charset=UTF-8’ not supported
Error:
{
"timestamp": "2021-12-13T11:49:33.305+00:00",
"status": 415,
"error": "Unsupported Media Type",
"path": "/api/v1/product/add"
}
If you are sure that there is no problem with your parameters, it is likely that the problem lies in your @requestbody AAA a
the problem I encounter here is that protobuf is used, but no corresponding bean is injected, resulting in an error. Just add the following code to the application:
PS: protobufmodule is our own extended com fasterxml.jackson.databind.Module class, it’s inconvenient to put it out. You can consult the data by yourself
@Bean
ProtobufJsonFormatHttpMessageConverter protobufHttpMessageConverter() {
return new ProtobufJsonFormatHttpMessageConverter();
}
@Bean
public Jackson2ObjectMapperBuilderCustomizer jsonCustomizer() {
return builder -> builder.serializationInclusion(JsonInclude.Include.NON_NULL)
.modules(new ProtobufModule(), new JavaTimeModule());
}