Swagger reported an error, IllegalStateException
I encountered a novel mistake today. Take a note.
Because of the project problem, I imported the swagger dependency into the common module. Then, because common is introduced into the gateway, check the dependency tree and confirm that the gateway also introduces the swagger dependency by default.
Due to the introduction of a problem, an error is reported when starting the gateway. The error is as follows:
java.lang.IllegalStateException: Failed to introspect Class [com.tang.config.SwaggerConfiguration] from ClassLoader [sun.misc.Launcher$AppClassLoader@18b4aac2]
at org.springframework.util.ReflectionUtils.getDeclaredMethods(ReflectionUtils.java:481)
at org.springframework.util.ReflectionUtils.doWithMethods(ReflectionUtils.java:358)
at org.springframework.util.ReflectionUtils.getUniqueDeclaredMethods(ReflectionUtils.java:414)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory
Caused by: java.lang.NoClassDefFoundError: springfox/documentation/spring/web/plugins/Docket
at java.lang.Class.getDeclaredMethods0(Native Method)
at java.lang.Class.privateGetDeclaredMethods(Class.java:2701)
at java.lang.Class.getDeclaredMethods(Class.java:1975)
at org.springframework.util.ReflectionUtils.getDeclaredMethods(ReflectionUtils.java:463)
... 33 common frames omitted
Caused by: java.lang.ClassNotFoundException: springfox.documentation.spring.web.plugins.Docket
at java.net.URLClassLoader.findClass(URLClassLoader.java:382)
at java.lang.ClassLoader.loadClass(ClassLoader.java:424)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:349)
at java.lang.ClassLoader.loadClass(ClassLoader.java:357)
... 37 common frames omitted
Because the error occurred after the swagger dependency was introduced. So I preliminarily determined that the error occurred in the two swagger dependencies I imported.
<!-- swagger -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
</dependency>
After querying the boss on stackoverflow, the answer is that swagger needs web dependency when it is started. If it does not exist, an error will be reported. I tried to add and found
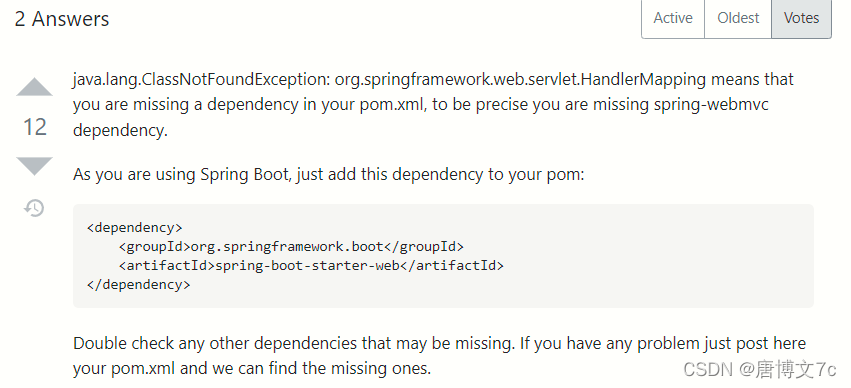
A respectful error is reported on the console:
**********************************************************
Spring MVC found on classpath, which is incompatible with Spring Cloud Gateway at this time. Please remove spring-boot-starter-web dependency.
**********************************************************
Because it was an error found in the gateway. Therefore, it is obvious that the spring cloud gateway integrates weblux and thus the web. Therefore, adding spring-boot-starter-web
to the project will cause the above error.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-gateway-server</artifactId>
<version>2.2.7.RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
<version>2.3.8.RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-loadbalancer</artifactId>
<version>2.2.7.RELEASE</version>
<scope>compile</scope>
<optional>true</optional>
</dependency>
Solution:
Because the gateway in the project does not need to integrate swagger, when introducing the basic module package with swagger in the gateway configuration, it only needs to be in POM XML settings <exclusions></exclusions>
<exclusions>
<exclusion>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
</exclusion>
<exclusion>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
</exclusion>
</exclusions>
Finally, because the related configuration classes of swagger are also written in the basic module. An error will also be reported because the swagger dependency is not imported. At this time, you only need to ignore the injection in the gateway gateway startup class.
@ComponentScan(excludeFilters = @ComponentScan.Filter(type = FilterType.ASSIGNABLE_TYPE, value = SwaggerConfiguration.class))