I want to achieve the following effects: click the column above to switch the content of the column below
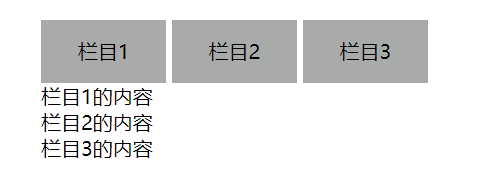
Write the code as follows (mainly see the JS part)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.tab{
display: inline-block;
width: 100px;
height: 50px;
background-color: #aaa;
}
.current{
background-color: yellow;
}
.content{
display: none;
}
</style>
</head>
<body>
<div class = "tab">
<div class = "tab_list">
<li>栏目1</li>
<li>栏目2</li>
<li>栏目3</li>
</div>
<div class = "tab_con" style="display: block;">栏目1的内容</div>
<div class = "tab_con">栏目2的内容</div>
<div class = "tab_con">栏目3的内容</div>
</div>
<script>
var tab_list = document.querySelector(".tab_list").querySelectorAll("li");
var tab_con = document.querySelectorAll(".tab_con");
for(var i = 0;i<tab_list.length;i++){
tab_list[i].onclick = function(){
for(var j = 0;j<tab_list.length;j++){
tab_list[j].className = "tab";
}
tab_list[i].className = "tab red";
for(var j = 0;j<tab_con.length;j++){
tab_con[j].style.display = "none";
}
tab_con[i].style.display = "block";
}
}
</script>
</body>
</html>
The result shows an error: uncaught typeerror: cannot set properties of undefined (setting 'classname') at htmldivelement.Tab.<computed>.onclick
Baidu tested it and found the following code:
<script>
var tab_list = document.querySelector(".tab_list").querySelectorAll("li");
var tab_con = document.querySelectorAll(".tab_con");
for(var i = 0;i<tab_list.length;i++){
tab_list[i].onclick = function(){
console.log("栏目" + i + "被点击了");
}
}
</script>
In printing, I is 3 instead of 0, 1 and 2.
After consulting the data, we know that:
In the for loop, for each tab_List is bound to the onclick event to listen, but when the function is executed, I has ended the loop, so the printout is 3.
That is, the event listening function in the for loop needs to avoid using the loop variable I
So, if tab is involved_List [i], we can use this; If tab is involved_Con [i], that is, use I to get other elements, so we can give tab_List adds an attribute index, and then in the onclick function, we get this attribute, that is, we get the I we want
The code is as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
*{
margin: 0;
padding: 0;
}
.tab{
width: 400px;
margin: 100px auto;
}
.tab .tab_list li{
display: inline-block;
width: 100px;
height: 50px;
line-height: 50px;
text-align: center;
background-color: #aaa;
}
.tab .tab_list .current{
background-color: yellow;
}
.content{
display: none;
}
</style>
</head>
<body>
<div class = "tab">
<div class = "tab_list">
<li>栏目1</li>
<li>栏目2</li>
<li>栏目3</li>
</div>
<div class = "tab_con" style="display: block;">栏目1的内容</div>
<div class = "tab_con">栏目2的内容</div>
<div class = "tab_con">栏目3的内容</div>
</div>
<script>
var tab_list = document.querySelector(".tab_list").querySelectorAll("li");
var tab_con = document.querySelectorAll(".tab_con");
for(var i = 0;i<tab_list.length;i++){
tab_list[i].setAttribute("index",i);
tab_list[i].onclick = function(){
var index = this.getAttribute("index");
console.log("栏目" + index + "被点击了");
for(var j = 0;j<tab_list.length;j++){
tab_list[j].className = "";
}
tab_list[index].className = "current";
console.log(this.className);
for(var j = 0;j<tab_con.length;j++){
tab_con[j].style.display = "none";
}
tab_con[index].style.display = "block";
}
}
</script>
</body>
</html>