As shown below, use create in Sqlalchemy_ Engine creates a database connection;
conn = create_engine('mssql+pymssql://' + dbuser + ':' + dbpassword + '@' + dbhost + '/' + database)
Then through pandas’ to_SQL method to the database,
df = pd.read_csv(filename, sep='|', header=0, quoting=3)
df.to_sql(name=filename + ty, con=conn, if_exists='replace', index=True)
Result error:
Traceback (most recent call last):
File "src\pymssql.pyx", line 450, in pymssql.Cursor.execute
File "src\_mssql.pyx", line 1064, in _mssql.MSSQLConnection.execute_query
File "src\_mssql.pyx", line 1095, in _mssql.MSSQLConnection.execute_query
File "src\_mssql.pyx", line 1228, in _mssql.MSSQLConnection.format_and_run_query
File "src\_mssql.pyx", line 1639, in _mssql.check_cancel_and_raise
File "src\_mssql.pyx", line 1683, in _mssql.maybe_raise_MSSQLDatabaseException
_mssql.MSSQLDatabaseException: (102, b"Incorrect syntax near '('.DB-Lib error message 20018, severity 15:\nGeneral SQL Server error: Check messages from the SQL Server\n")
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
Supplementary error reporting exception information:
Traceback (most recent call last):
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\base.py", line 1264, in _execute_context
cursor, statement, parameters, context
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\default.py", line 587, in do_executemany
cursor.executemany(statement, parameters)
File "src\pymssql.pyx", line 476, in pymssql.Cursor.executemany
File "src\pymssql.pyx", line 465, in pymssql.Cursor.execute
pymssql.ProgrammingError: (102, b"Incorrect syntax near '('.DB-Lib error message 20018, severity 15:\nGeneral SQL Server error: Check messages from the SQL Server\n")
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\contextlib.py", line 130, in __exit__
self.gen.throw(type, value, traceback)
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\pandas\io\sql.py", line 948, in run_transaction
yield tx
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\pandas\io\sql.py", line 641, in insert
self._execute_insert(conn, keys, chunk_iter)
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\pandas\io\sql.py", line 616, in _execute_insert
conn.execute(self.insert_statement(), data)
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\base.py", line 1020, in execute
return meth(self, multiparams, params)
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\sql\elements.py", line 298, in _execute_on_connection
return connection._execute_clauseelement(self, multiparams, params)
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\base.py", line 1139, in _execute_clauseelement
distilled_params,
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\base.py", line 1324, in _execute_context
e, statement, parameters, cursor, context
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\base.py", line 1518, in _handle_dbapi_exception
sqlalchemy_exception, with_traceback=exc_info[2], from_=e
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\util\compat.py", line 178, in raise_
raise exception
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\base.py", line 1264, in _execute_context
cursor, statement, parameters, context
File "C:\Users\****\AppData\Local\Programs\Python\Python37-32\lib\site-packages\sqlalchemy\engine\default.py", line 587, in do_executemany
cursor.executemany(statement, parameters)
File "src\pymssql.pyx", line 476, in pymssql.Cursor.executemany
File "src\pymssql.pyx", line 465, in pymssql.Cursor.execute
sqlalchemy.exc.ProgrammingError: (pymssql.ProgrammingError) (102, b"Incorrect syntax near '('.DB-Lib error message 20018, severity 15:\nGeneral SQL Server error: Check messages from the SQL Server\n")
Mainly look at this line, which literally means [syntax error near ‘(‘):
_mssql.MSSQLDatabaseException: (102, b"Incorrect syntax near '('.DB-Lib error message 20018, severity 15:\nGeneral SQL Server error: Check messages from the SQL Server\n")
Generally, we will first check whether the SQL is correct (or whether there are ‘special characters’ in the data), but strangely
When this program runs locally, it reads the same file and imports the same database table. Everything is normal!
At this time, it is suspected that the running environment on the local and remote servers is inconsistent, and the inspection found that it was not surprising; The version of Sqlalchemy on the remote is rather old, only 0.23.0
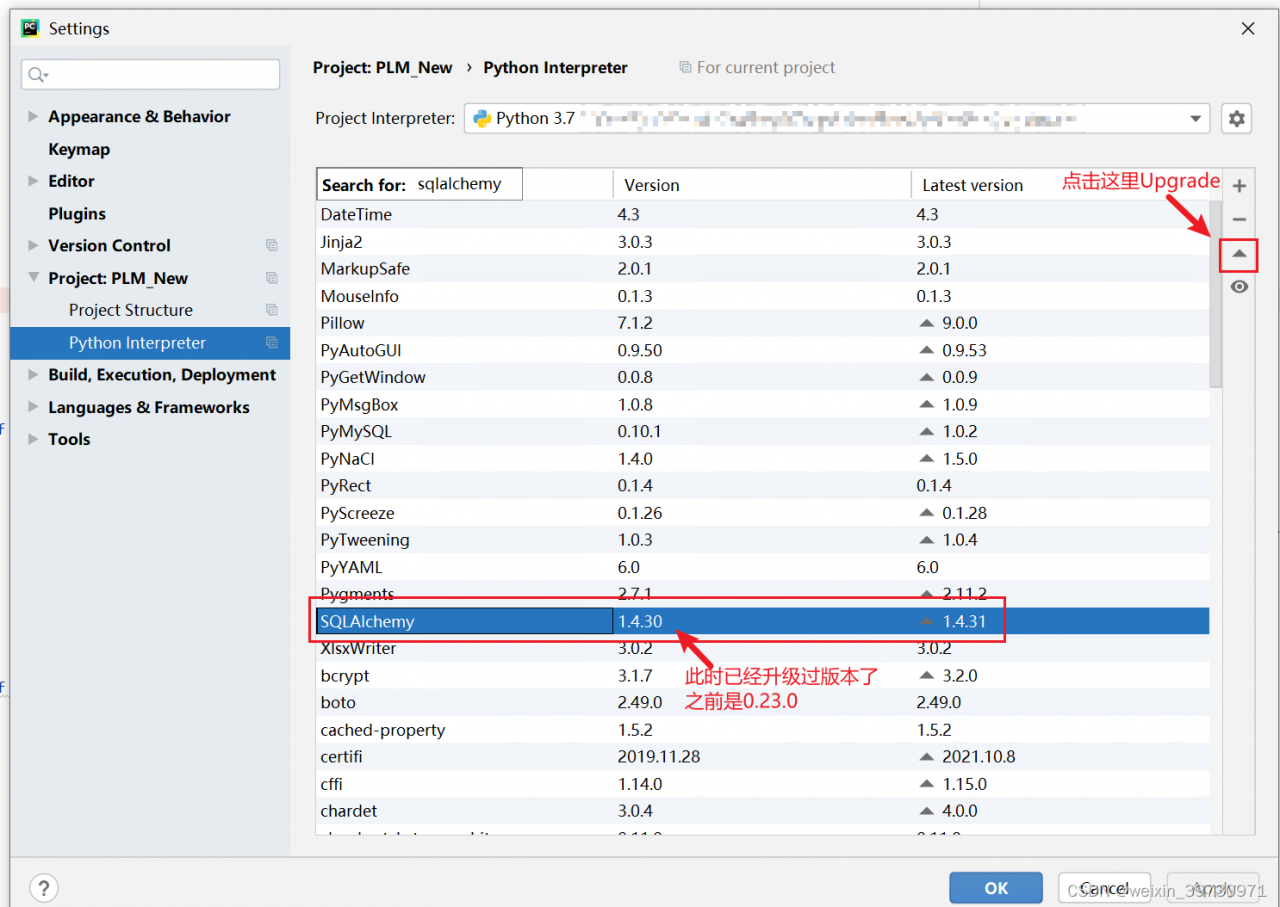
So I decided to upgrade and run again. Everything was normal~