After updating Fuller, the following problems will be reported:
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':app:checkDebugAarMetadata'.
> Multiple task action failures occurred:
> A failure occurred while executing com.android.build.gradle.internal.tasks.CheckAarMetadataWorkAction
> The minCompileSdk (31) specified in a
dependency's AAR metadata (META-INF/com/android/build/gradle/aar-metadata.properties)
is greater than this module's compileSdkVersion (android-30).
Dependency: androidx.window:window-java:1.0.0-beta04.
AAR metadata file: C:\Users\liqiang\.gradle\caches\transforms-2\files-2.1\9f515ee58db509b5f4759e97c8eb6aa2\jetified-window-java-1.0.0-beta04\META-INF\com\android\build\gradle\aar-metadata.properties.
> A failure occurred while executing com.android.build.gradle.internal.tasks.CheckAarMetadataWorkAction
> The minCompileSdk (31) specified in a
dependency's AAR metadata (META-INF/com/android/build/gradle/aar-metadata.properties)
is greater than this module's compileSdkVersion (android-30).
Dependency: androidx.window:window:1.0.0-beta04.
AAR metadata file: C:\Users\liqiang\.gradle\caches\transforms-2\files-2.1\a909ff21160c236fa8213aba5c707997\jetified-window-1.0.0-beta04\META-INF\com\android\build\gradle\aar-metadata.properties.
* Try:
Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. Run with --scan to get full insights.
* Get more help at https://help.gradle.org
BUILD FAILED in 4s
Exception: Gradle task assembleDebug failed with exit code 1
According to the error message The minCompileSdk (31) specified, the final sdk needs to be version 31, but our current sdk version is 30, which is greater than this version, so we need to modify the following configuration to 31
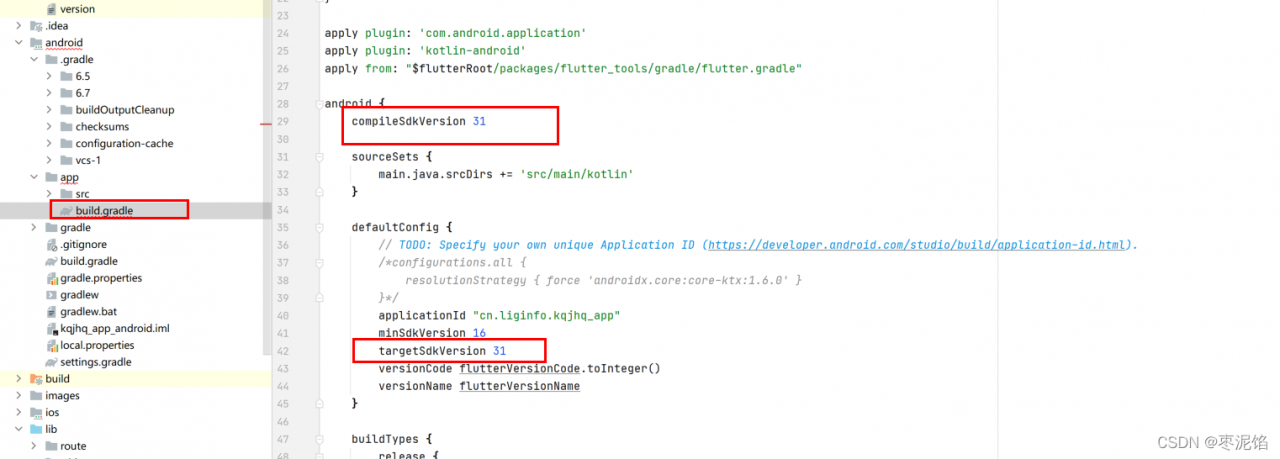
android {
compileSdkVersion 31
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
defaultConfig {
// TODO: Specify your own unique Application ID (https://developer.android.com/studio/build/application-id.html).
/*configurations.all {
resolutionStrategy { force 'androidx.core:core-ktx:1.6.0' }
}*/
applicationId "cn.liginfo.kqjhq_app"
minSdkVersion 16
targetSdkVersion 31
versionCode flutterVersionCode.toInteger()
versionName flutterVersionName
}
buildTypes {
release {
// TODO: Add your own signing config for the release build.
// Signing with the debug keys for now, so `flutter run --release` works.
signingConfig signingConfigs.debug
}
}
}
After modification, because there is no version 31 sdk, it will download automatically, but after downloading, the following error message appears again
Flutter assets will be downloaded from https://storage.flutter-io.cn. Make sure you trust this source!
Launching lib\main.dart on sdk gphone x86 arm in debug mode...
Running Gradle task 'assembleDebug'...
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/0dd39ff9faeeb501ffb52162e269003e/jetified-kotlin-stdlib-1.5.31.jar!/META-INF/kotlin-stdlib.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/2ea246a87a592f122121cfd0846ffbea/jetified-kotlin-stdlib-jdk7-1.5.30.jar!/META-INF/kotlin-stdlib-jdk7.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/9234cfcee40ec23fc2213363e9726513/jetified-window-1.0.0-beta04-api.jar!/META-INF/window_release.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/9af1d6ff9000d18681a83cd875960957/jetified-kotlinx-coroutines-core-jvm-1.5.2.jar!/META-INF/kotlinx-coroutines-core.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/b0b1eecff74e71ed1ef092403ad1018c/jetified-kotlin-stdlib-common-1.5.31.jar!/META-INF/kotlin-stdlib-common.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/d91778f7878bf943ae593906c9fd7c75/jetified-kotlin-stdlib-jdk8-1.5.30.jar!/META-INF/kotlin-stdlib-jdk8.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/df6880e9350e429186aa0973bbf49093/jetified-window-java-1.0.0-beta04-api.jar!/META-INF/window-java_release.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
e: C:/Users/liqiang/.gradle/caches/transforms-2/files-2.1/e484fc5cda5fe73d156ca3b50e47d38e/jetified-kotlinx-coroutines-android-1.5.2.jar!/META-INF/kotlinx-coroutines-android.kotlin_module: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is 1.5.1, expected version is 1.1.15.
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':app:compileDebugKotlin'.
> Compilation error. See log for more details
* Try:
Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. Run with --scan to get full insights.
* Get more help at https://help.gradle.org
BUILD FAILED in 7s
┌─ Flutter Fix ────────────────────────────────────────────────────────────────────────────────┐
│ [!] Your project requires a newer version of the Kotlin Gradle plugin. │
│ Find the latest version on https://kotlinlang.org/docs/gradle.html#plugin-and-versions, then │
│ update D:\javadeveloping\Android\workspace\kqjhq_app\android\build.gradle: │
│ ext.kotlin_version = '<latest-version>' │
└──────────────────────────────────────────────────────────────────────────────────────────────┘
Exception: Gradle task assembleDebug failed with exit code 1
From the error message, it is said that the version of kotlin is wrong, and a specific solution is given
Your project requires a newer version of the Kotlin Gradle plugin. │
│ Find the latest version on https://kotlinlang.org/docs/gradle.html#plugin-and-versions, then │
│ update D:\javadeveloping\Android\workspace\kqjhq_app\android\build.gradle: │
│ ext.kotlin_version = '<latest-version>'
You need an up-to-date plugin named kotlin gradle
, Find the recent version in https://kotlinlang.org/docs/gradle.html#plugin-and-versions
and update the the latest-version
value in ext.kotlin_version = ‘’
of the D:\javadeveloping\Android\workspace\kqjhq_app\android\build.gradle
file.
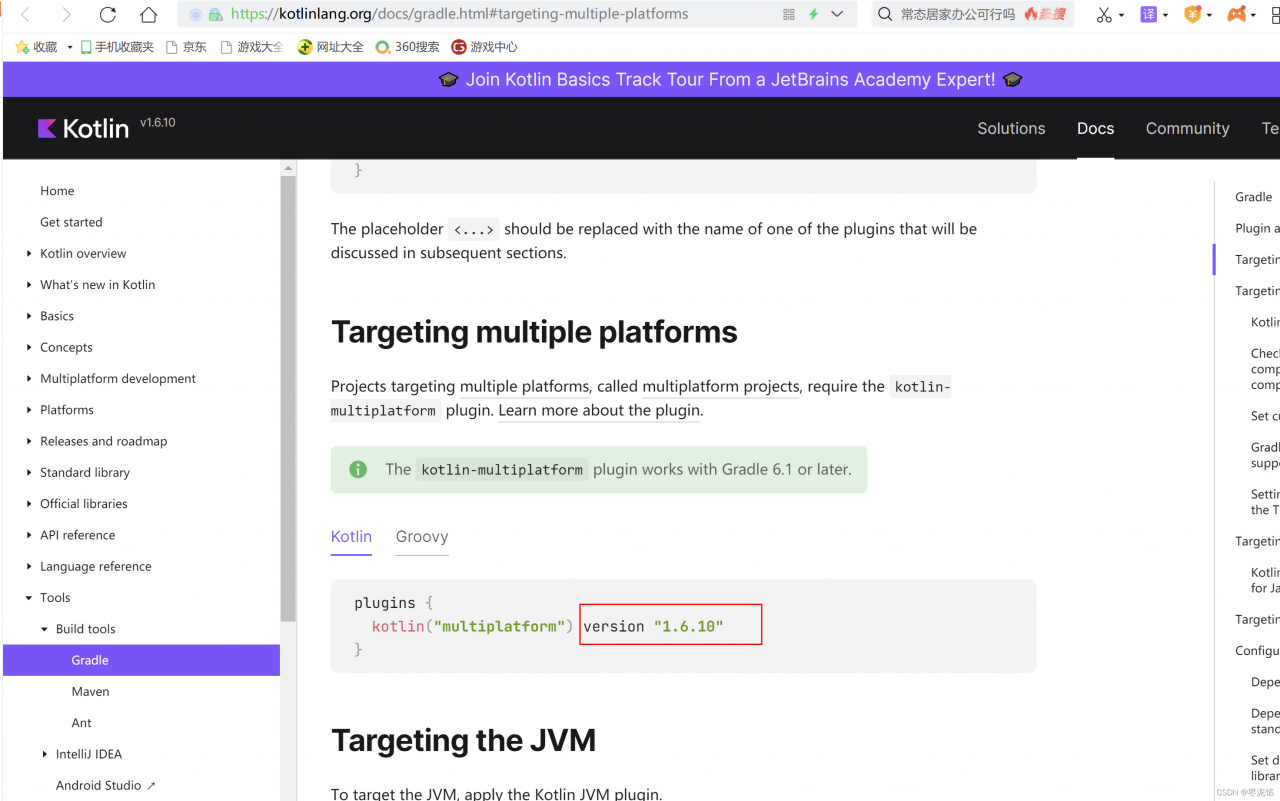
after opening the web page, we found that the latest version is 1.6.10, while our previous version is 1.3.5:
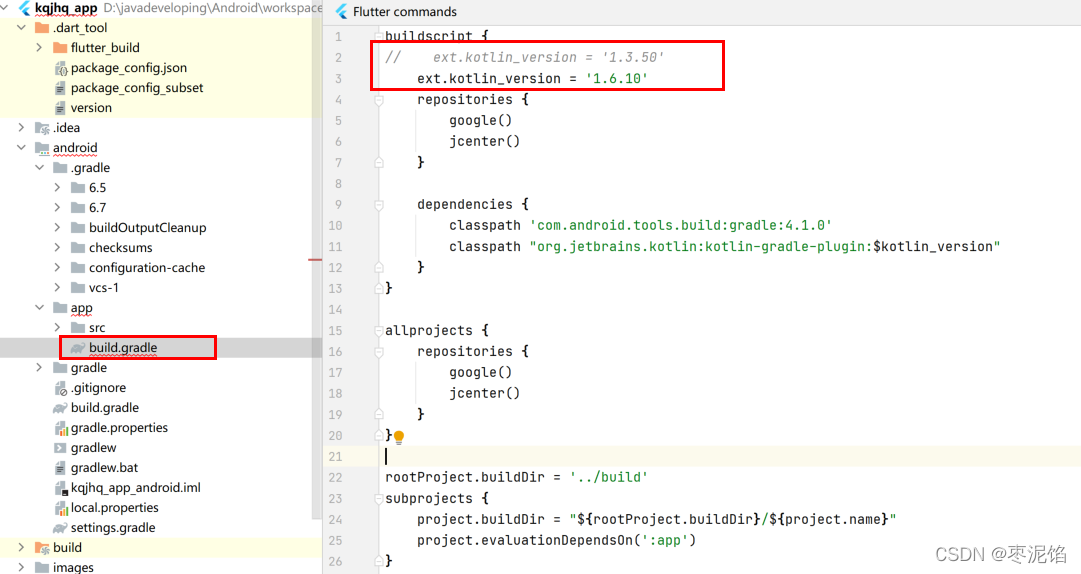
just change to 1.6.10 and package normally