preface
When using pycharm to install a third-party package in the python virtual environment in Python interpreter
settings, it is likely to report an error: non zero exit code (2)
, as shown in the figure:
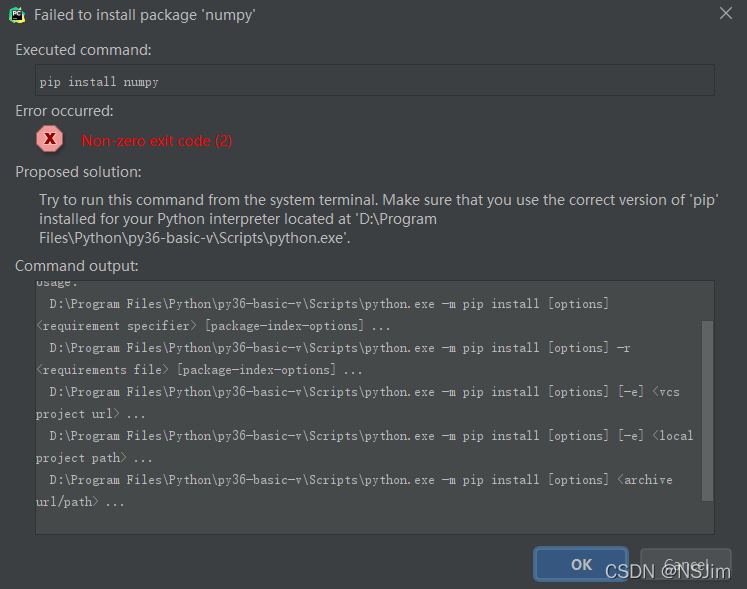
even upgrading pip will report an error, as shown in the figure:
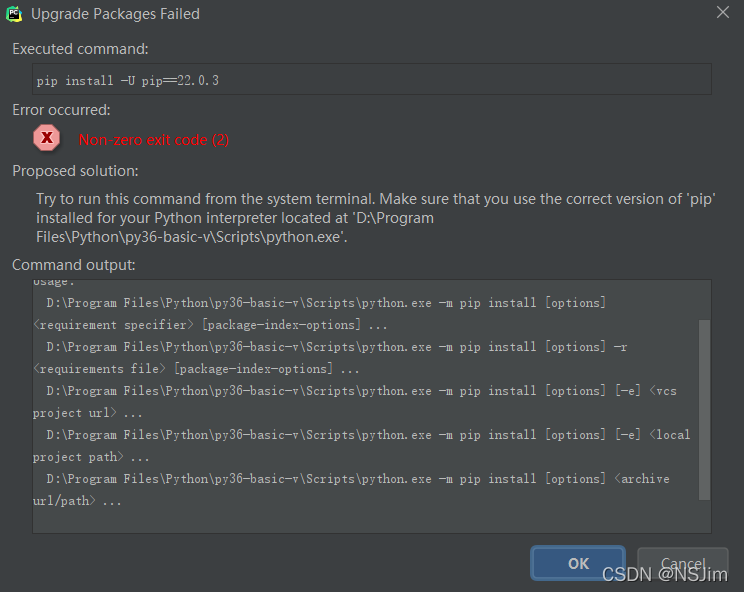
Tips are as follows:
Try to run this command from the system terminal.
Make sure that you use the correct version of 'pip' installed for your Python interpreter located at
'D:\Program Files\Python\py36-basic-v\Scripts\python.exe'.
As above belongs, the official says the possible reason is that pip is not installed in the specified directory of the virtual environment, and suggests running the pip command in the terminal to install the third-party package.
The official hint has a certain degree of reasonableness, but it does not try all cases, you can check whether the pip module exists in the directory of the virtual environment, if it does not exist, the pip module can be installed in the specified directory; if it exists, it is not the problem of the pip module path, so what exactly is the reason?
After investigation, it is the problem of pip version, my Python version is 3.6, pip version is 21.3.1, this version of pip will definitely appear the problem, the solution is also very simple, the pip version can be downgraded to 20.2.4, operation method see the following program 2: Downgrade pip version. (It is not recommended to upgrade pip, because new versions of pip may have the same problem)
Solution 1 (recommended): install the third-party package using the terminal terminal
Click Terminal
at the bottom of pycharm toolbar , as shown in the following figure:
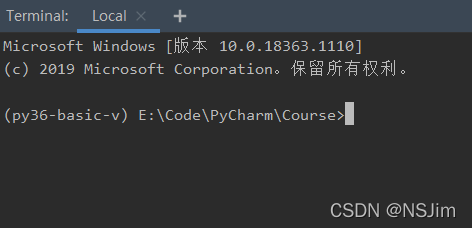
enter the PIP install
command to install the third-party package, as shown in the figure:
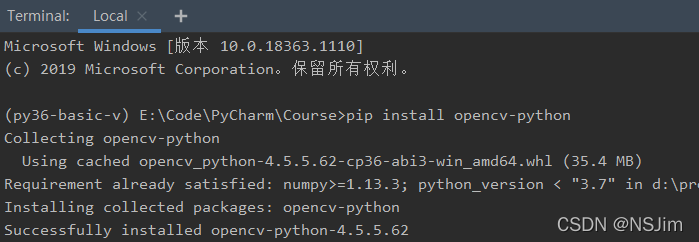
But this solution treats the symptom but not the root cause, the problem is not solved, only the installation of third-party packages by alternative methods, if you want to solve the problem at all, please see solution 2 below.
Solution 2 (fundamental solution): downgrade the PIP version
First, open a project using pycharm. If there is no Python environment, you need to create a virtual environment first, as shown in the following figure:
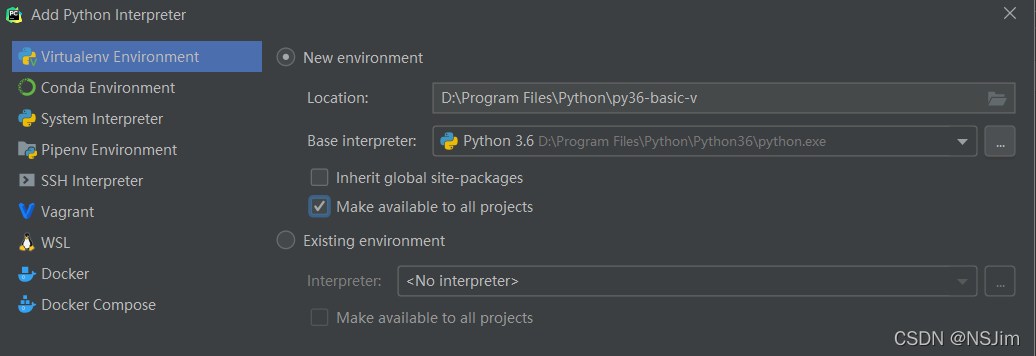
In file - Settings - Python interpreter
, we can see that the version of PIP in Python virtual environment is 21.3.1. As shown in the figure below, we need to downgrade the version of Pip to 20.2.4
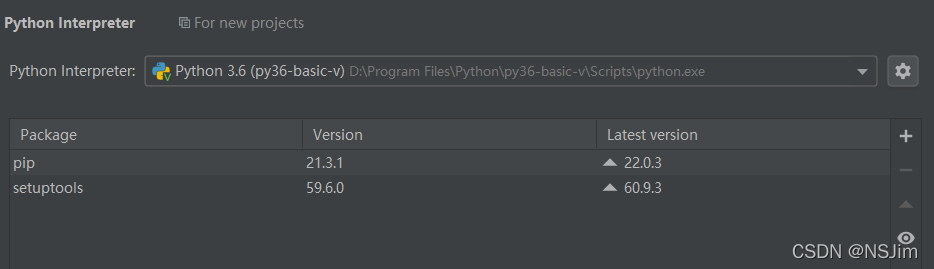
Click Terminal
on the toolbar at the bottom of pychart, as shown in the following figure:
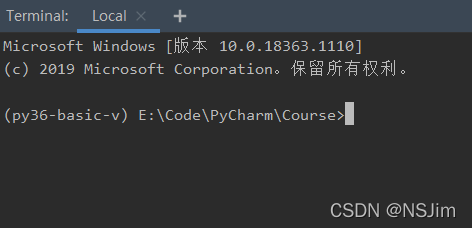
enter the following command. Note that there must be Python -m
, otherwise there is no permission:
python -m pip install pip==20.2.4
After entering the command, the following prompt appears to prove that the downgrade is successful:
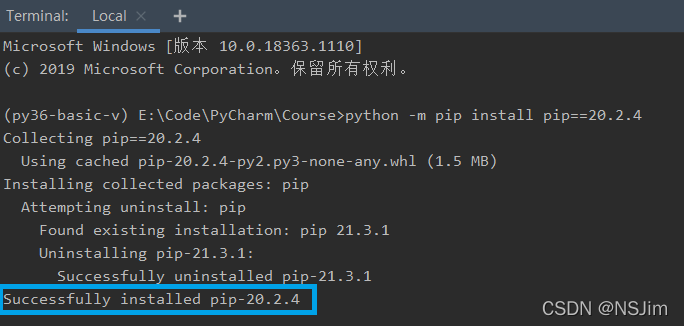
enter the command PIP - V
to view the current PIP version, as shown in the following figure:
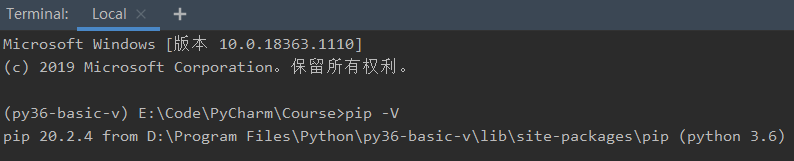
the problem has been successfully solved so far. The third-party package can be successfully installed in Settings
, as shown in the following figure:
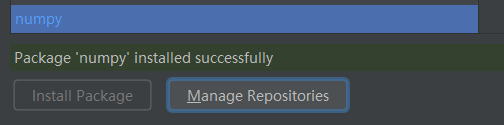