Problem Description:
The project started well in the morning, but not in the afternoon 😂 What is this situation… At first, the project could not be started because the HTTP resources would be filtered out after Maven version 3.8.1. Later, it was still impossible to change HTTP to HTTPS,
The best way is to modify the Maven version. I changed it to Maven version 3.6.3. After that, I can download the package. However, it didn’t take long before there was another problem. Malformed \uxxxx encoding.
Let’s start with this error report today: malformed \ uxxxx encoding (the problem encountered today has been solved for half an afternoon and the reason has finally been found…)
Solution Summary:
First of all, the solutions are nothing more than the following:
1, first check the project .properties, .yml, pom.xml, logback and other configurations, whether there is a path error in the use of
2, update the maven repository, re-download the jar package
3, delete the path-to-the-library or resolver-status.properties file
In either case, it is recommended to restart the editor. For idea, click the Invalidate Caches/ Restart button to clear the cache and restart the idea
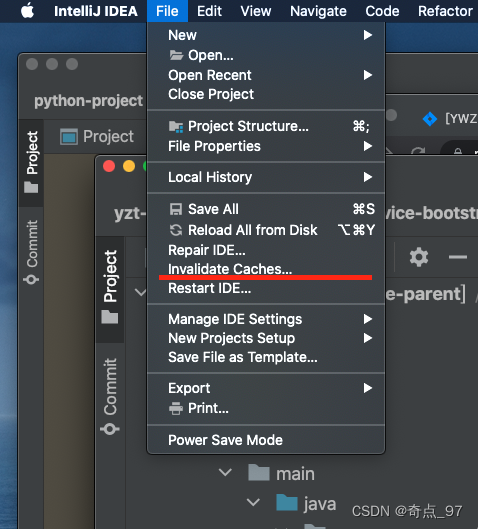
After opening IDEA and updating the project to start.
1, suddenly found build failure reported a strange error: Malformed \uxxxx encoding.
2. The <project> tag in the pom.xml file is also marked in red with the error.
But but but the code in the pom is exactly the same as on master, others are fine, but not their own, some of the import classes in the file will also report an error Can’t find.
Reason: When updating the jar packages that the project depends on, the downloaded jar packages may be incomplete due to network problems.
Solution: Delete the downloaded jar package and download it again.
In fact, it is not necessary to delete and re-download the package in general, so I think there must be a way to find the problematic package. To do accurate deletion
The quick solution is:
# Use the -X parameter to type out the error file information
mvn pakage -X
# Check the log, then delete or change the name of the file with the error to something else
# This will allow you to re-download the wrong file
I solved this problem through method 3
(1) In the . /m2/ folder (modify as you see fit), find path-to-the-library and delete it (if this file is not present, you can simply ignore this step).
(2) In the . /m2/repository (modify according to your situation) folder, search globally for the file: resolver-status.properties, delete all the files found, and recompile.