[Arthas failed to bind telnet or http port! Telnet port: 3658, http port: 8563; Error creating bean with name ‘documentationPluginsBootstrapper’ defined in URL; Error creating bean with name ‘webMvcRequestHandlerProvider’ defined in URL]
Cause analysis:
Error will be reported when two identical items are started: port conflict!!! (Even if the ports of the two projects are different in the yml file, an error will be reported.)
Arthas failed to bind telnet or http port! Telnet port: 3658, http port: 8563
Solution:
Comment out the arthas in two projects.
<!-- Monitor system performance: do not allow two identical projects to run; comment to enable two -->
<!-- <dependency>-->
<!-- <groupId>com.taobao.arthas</groupId>-->
<!-- <artifactId>arthas-spring-boot-starter</artifactId>-->
<!-- <version>3.6.0</version>-->
<!-- </dependency>-->
Arthas is an online monitoring and diagnosis product. It can view the status information of application load, memory, gc, and threads in real time from a global perspective, and diagnose business problems without modifying the application code, including checking the access parameters and exceptions of method calls, time-consuming monitoring method execution, class loading information, etc., greatly improving the efficiency of online problem troubleshooting.
Error 1
org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'documentationPluginsBootstrapper' defined in URL
[jar:file:/D:/JAVA1/scm-repository/io/springfox/springfox-spring-web/3.0.0/springfox-spring-web-3.0.0.jar!/springfox/documentation/spring/web/plugins/DocumentationPluginsBootstrapper.class]:
Unsatisfied dependency expressed through constructor parameter 1; nested exception is org.springframework.beans.factory.UnsatisfiedDependencyException:
Error creating bean with name 'webMvcRequestHandlerProvider' defined in URL
[jar:file:/D:/JAVA1/scm-repository/io/springfox/springfox-spring-webmvc/3.0.0/springfox-spring-webmvc-3.0.0.jar!/
springfox/documentation/spring/web/plugins/WebMvcRequestHandlerProvider.class]: Unsatisfied dependency expressed through constructor parameter 2; nested exception is org.springframework.beans.factory.BeanCreationException:
Error creating bean with name 'webEndpointServletHandlerMapping' defined in class path resource [org/springframework/boot/actuate/autoconfigure/endpoint/web/servlet/WebMvcEndpointManagementContextConfiguration.class]:
Bean instantiation via factory method failed; nested exception is org.springframework.beans.BeanInstantiationException: Failed to instantiate
[org.springframework.boot.actuate.endpoint.web.servlet.WebMvcEndpointHandlerMapping]: Factory method 'webEndpointServletHandlerMapping' threw exception;
nested exception is org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'arthasEndPoint': Unsatisfied dependency expressed through field 'arthasAgent'; nested exception is
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'arthasAgent' defined in class path resource
[com/alibaba/arthas/spring/ArthasConfiguration.class]: Bean instantiation via factory method failed; nested exception is
org.springframework.beans.BeanInstantiationException: Failed to instantiate [com.taobao.arthas.agent.attach.ArthasAgent]: Factory method 'arthasAgent' threw exception; nested exception is java.lang.IllegalStateException:
java.lang.reflect.InvocationTargetException
Caused by: org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'webMvcRequestHandlerProvider' defined in URL
[jar:file:/D:/JAVA1/scm-repository/io/springfox/springfox-spring-webmvc/3.0.0/springfox-spring-webmvc-
3.0.0.jar!/springfox/documentation/spring/web/plugins/WebMvcRequestHandlerProvider.class]: Unsatisfied dependency expressed through constructor parameter 2; nested exception is org.springframework.beans.factory.BeanCreationException: Error
creating bean with name 'webEndpointServletHandlerMapping' defined in class path resource
[org/springframework/boot/actuate/autoconfigure/endpoint/web/servlet/WebMvcEndpointManagementContextConfiguration.class]: Bean instantiation via factory method failed; nested exception is org.springframework.beans.BeanInstantiationException: Failed to instantiate
[org.springframework.boot.actuate.endpoint.web.servlet.WebMvcEndpointHandlerMapping]: Factory method 'webEndpointServletHandlerMapping' threw exception;
nested exception is org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'arthasEndPoint': Unsatisfied dependency expressed through field 'arthasAgent'; nested exception is
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'arthasAgent' defined in class path resource
[com/alibaba/arthas/spring/ArthasConfiguration.class]: Bean instantiation via factory method failed; nested exception is org.springframework.beans.BeanInstantiationException:
Failed to instantiate [com.taobao.arthas.agent.attach.ArthasAgent]: Factory method 'arthasAgent' threw exception; nested exception is java.lang.IllegalStateException: java.lang.reflect.InvocationTargetException
Caused by: org.springframework.beans.BeanInstantiationException: Failed to instantiate
[org.springframework.boot.actuate.endpoint.web.servlet.WebMvcEndpointHandlerMapping]: Factory method 'webEndpointServletHandlerMapping' threw exception; nested exception is org.springframework.beans.factory.UnsatisfiedDependencyException:
Error creating bean with name 'arthasEndPoint': Unsatisfied dependency expressed through field 'arthasAgent'; nested exception is
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'arthasAgent' defined in class path resource [com/alibaba/arthas/spring/ArthasConfiguration.class]: Bean instantiation via factory method failed; nested exception is
org.springframework.beans.BeanInstantiationException: Failed to instantiate
[com.taobao.arthas.agent.attach.ArthasAgent]: Factory method 'arthasAgent' threw exception; nested exception is java.lang.IllegalStateException:
java.lang.reflect.InvocationTargetException
at org.springframework.beans.factory.support.SimpleInstantiationStrategy.instantiate(SimpleInstantiationStrategy.java:185)
at org.springframework.beans.factory.support.ConstructorResolver.instantiate(ConstructorResolver.java:653)
... 57 common frames omitted
Caused by: org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'arthasEndPoint': Unsatisfied dependency expressed through field 'arthasAgent'; nested exception is
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'arthasAgent' defined in class path resource
[com/alibaba/arthas/spring/ArthasConfiguration.class]: Bean instantiation via factory method failed; nested exception is
org.springframework.beans.BeanInstantiationException: Failed to instantiate [com.taobao.arthas.agent.attach.ArthasAgent]:
Factory method 'arthasAgent' threw exception; nested exception is java.lang.IllegalStateException: java.lang.reflect.InvocationTargetException
Caused by: org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'arthasAgent' defined in class path resource
[com/alibaba/arthas/spring/ArthasConfiguration.class]: Bean instantiation via factory method failed; nested exception is org.springframework.beans.BeanInstantiationException: Failed to instantiate [com.taobao.arthas.agent.attach.ArthasAgent]: Factory method 'arthasAgent' threw exception; nested exception is java.lang.IllegalStateException:
java.lang.reflect.InvocationTargetException
Caused by: java.lang.IllegalStateException: Arthas failed to bind telnet or http port! Telnet port: 3658, http port: 8563
at com.taobao.arthas.core.server.ArthasBootstrap.bind(ArthasBootstrap.java:448)
at com.taobao.arthas.core.server.ArthasBootstrap.<init>
(ArthasBootstrap.java:156)
at com.taobao.arthas.core.server.ArthasBootstrap.getInstance(ArthasBootstrap.java:581)
... 112 common frames omitted
Disconnected from the target VM, address: '127.0.0.1:58928', transport: 'socket'
Error creating bean with name 'arthasEndPoint': Unsatisfied dependency expressed through field 'arthasAgent'; nested exception is
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'arthasAgent' defined in class path resource
[com/alibaba/arthas/spring/ArthasConfiguration.class]: Bean instantiation via factory method failed; nested exception is
org.springframework.beans.BeanInstantiationException: Failed to instantiate [com.taobao.arthas.agent.attach.ArthasAgent]:
Factory method 'arthasAgent' threw exception; nested exception is java.lang.IllegalStateException: java.lang.reflect.InvocationTargetException
Error 2
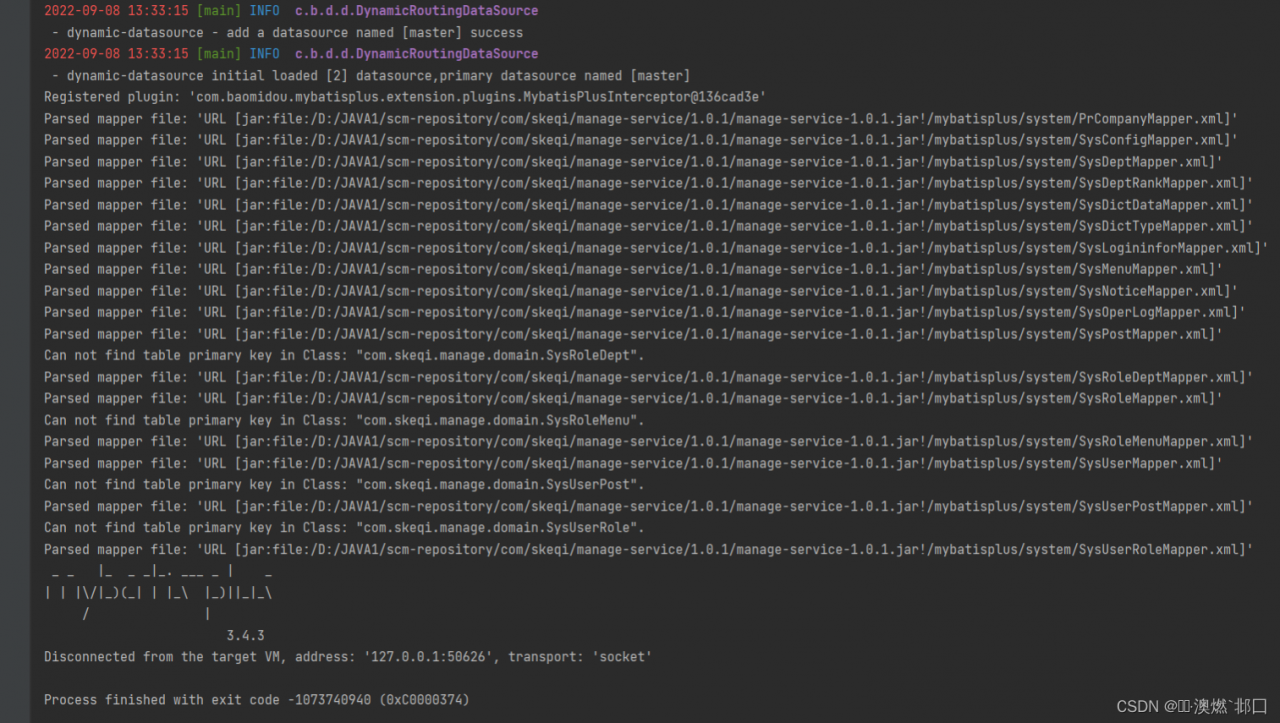
2022-09-08 13:33:15 [main] INFO c.b.d.d.DynamicRoutingDataSource
- dynamic-datasource - add a datasource named [master] success
2022-09-08 13:33:15 [main] INFO c.b.d.d.DynamicRoutingDataSource
- dynamic-datasource initial loaded [2] datasource,primary datasource named [master]
Registered plugin: 'com.baomidou.mybatisplus.extension.plugins.MybatisPlusInterceptor@136cad3e'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/PrCompanyMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysConfigMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysDeptMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysDeptRankMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysDictDataMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysDictTypeMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysLogininforMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysMenuMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysNoticeMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysOperLogMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysPostMapper.xml]'
Can not find table primary key in Class: "com.skeqi.manage.domain.SysRoleDept".
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysRoleDeptMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysRoleMapper.xml]'
Can not find table primary key in Class: "com.skeqi.manage.domain.SysRoleMenu".
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysRoleMenuMapper.xml]'
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysUserMapper.xml]'
Can not find table primary key in Class: "com.skeqi.manage.domain.SysUserPost".
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysUserPostMapper.xml]'
Can not find table primary key in Class: "com.skeqi.manage.domain.SysUserRole".
Parsed mapper file: 'URL [jar:file:/D:/JAVA1/scm-repository/com/skeqi/manage-service/1.0.1/manage-service-1.0.1.jar!/mybatisplus/system/SysUserRoleMapper.xml]'
_ _ |_ _ _|_. ___ _ | _
| | |\/|_)(_| | |_\ |_)||_|_\
/ |
3.4.3
Disconnected from the target VM, address: '127.0.0.1:50626', transport: 'socket'
Process finished with exit code -1073740940 (0xC0000374)