When mysql2 is used to operate the database in express, the paging query will report an error error incorrect arguments to mysqld_stmt_execute
Question
Error reporting: error: incorrect arguments to mysqld_stmt_execute
// Query notes based on number of notes and number of pages
async getSomeNote(num, page) {
const fromNum = (page - 1) * num
const statement = `SELECT id,note_title,note_describe,note_createtime,note_sort FROM notes_test LIMIT ? ,? ;`
// There is a problem with the parameters fromNum, num passed in here, they are numeric at this point
const result = await connections.execute(statement, [fromNum, num])
console.log(result[0]);
return result[0]
}
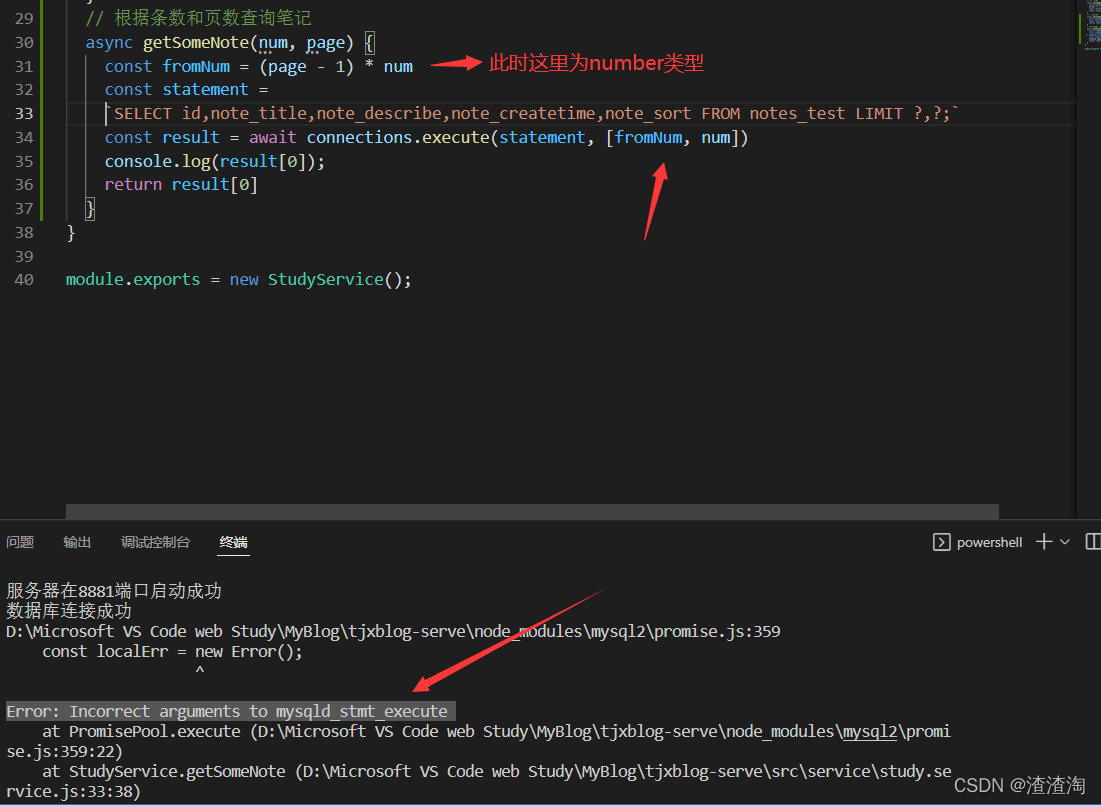
reason
Statement is a query statement for operating the database and is of string type. The contents of the second parameter of execute will be inserted into the statement. At this time, the number type inserted should be of string type, so an error “wrong parameter” is reported.
Solution:
Change the passed in parameter to string type.
// Query notes based on number of notes and number of pages
async getSomeNote(num, page) {
const fromNum = (page - 1) * num
const statement = `SELECT id,note_title,note_describe,note_createtime,note_sort FROM notes_test LIMIT ? ,? ;`
// Direct string concatenation, converting number types to character types
const result = await connections.execute(statement, [fromNum+'', num+''])
console.log(result[0]);
return result[0]
}
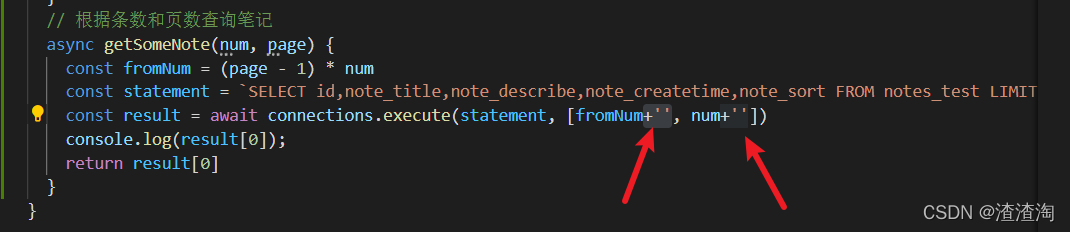