For example, we have access to mysql through the API provided by PHP extension Mysqli. The error report is: Class ‘mysqli’ not found. The reason for this error is that PHP was unable to load the dynamic library php_mysqli.dll. The solution is to configure the relevant variables so that the program finds the location.
By default php_mysqli. DLL is existing in the PHP installation directory under ext directory:
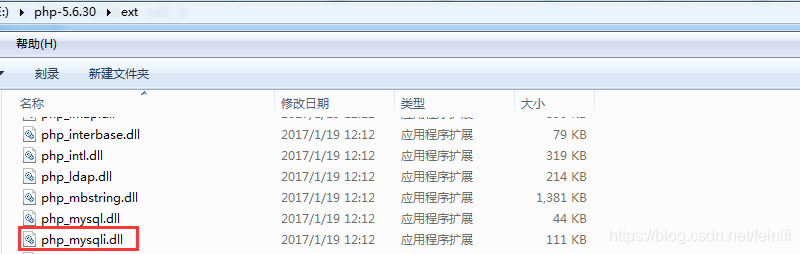
The first thing we need to do is to modify the PHP configuration file. The configuration file in Windows is in php.ini under the PHP installation directory. If not, you can make a copy through PHp.ini-Development. We found one of them; Extension =php_mysqli.dll, remove the semicolon at the beginning to allow the configuration to take effect. This means that you can use the MySQli extension.

In general, this is fine, but our configuration file PHp.ini will not take effect if our system does not configure the environment variable PHPRC. In order for php.ini to take effect, we need to configure the environment variable PHPRC, which specifies the PHP installation directory.
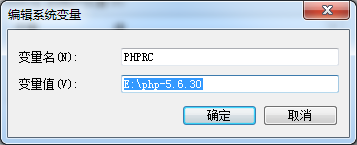
After configuring the environment variables, we can restart the Apache server. We can verify our PHP-related information by using phpInfo (). First, the Configuration File is in the Loaded Configuration File, not “none”, but php.ini under the PHP installation directory.

The second is that the mySQli extension appears in the middle of the page. If one of the above two steps is missing, this part will not be displayed. When we call the MYSQli API in our PHP code, we will report an error:

said that php_mysqli.dll from ext in the PHP installation directory should be copied to C:\Windows\ System32. This is because php.ini has not taken effect, and php.ini should be copied to C:\Windows directory.
Next, we can query the emP table ID and name in the mysql database test through a program, the code is as follows:
<?php
$servername = "127.0.0.1";
$username = "root";
$password = "root";
$dbname = "test";
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connect Fail " . $conn->connect_error);
}
$sql = "SELECT id, name FROM emp";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "id: " . $row["id"]. " - name: " . $row["name"]. "<br>";
}
} else {
echo "0 output";
}
$conn->close();
We visited the PHP file on the page and got the following results:
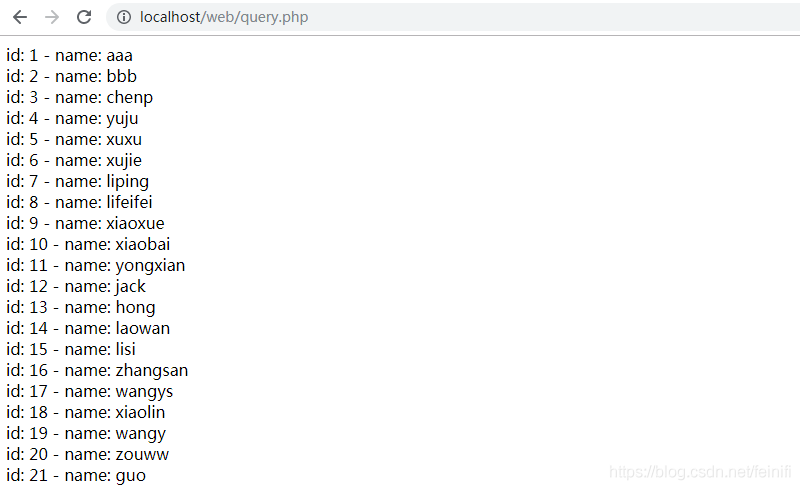
A simple mysqli application and the problem of mysqli extension are solved.